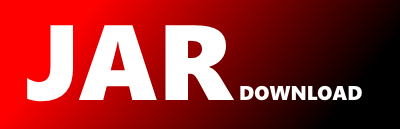
gdt.jgui.entity.edge.JBondsPanel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Graph Show documentation
Show all versions of Graph Show documentation
The graph extension for the entigrator application
The newest version!
package gdt.jgui.entity.edge;
/*
* Copyright 2016 Alexander Imas
* This file is part of JEntigrator.
JEntigrator is free software: you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
JEntigrator is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with JEntigrator. If not, see .
*/
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.Comparator;
import java.util.Properties;
import java.util.Stack;
import java.util.logging.Logger;
import gdt.data.entity.BaseHandler;
import gdt.data.entity.BondDetailHandler;
import gdt.data.entity.EdgeHandler;
import gdt.data.entity.EntityHandler;
import gdt.data.entity.NodeHandler;
import gdt.data.entity.facet.ExtensionHandler;
import gdt.data.entity.facet.FieldsHandler;
import gdt.data.grain.Core;
import gdt.data.grain.Identity;
import gdt.data.grain.Locator;
import gdt.data.grain.Sack;
import gdt.data.grain.Support;
import gdt.data.store.Entigrator;
import gdt.jgui.console.JConsoleHandler;
import gdt.jgui.console.JContext;
import gdt.jgui.console.JFacetOpenItem;
import gdt.jgui.console.JFacetRenderer;
import gdt.jgui.console.JItemPanel;
import gdt.jgui.console.JItemsListPanel;
import gdt.jgui.console.JMainConsole;
import gdt.jgui.console.JRequester;
import gdt.jgui.console.ReloadDialog;
import gdt.jgui.entity.JEntityFacetPanel;
import gdt.jgui.entity.JEntityPrimaryMenu;
import gdt.jgui.entity.JReferenceEntry;
import gdt.jgui.entity.bonddetail.JBondDetailPanel;
import gdt.jgui.entity.graph.JGraphRenderer;
import gdt.jgui.tool.JTextEditor;
import javax.swing.JMenu;
import javax.swing.JMenuItem;
import javax.swing.JOptionPane;
import javax.swing.event.MenuEvent;
import javax.swing.event.MenuListener;
import org.apache.commons.codec.binary.Base64;
/**
* This class displays a list of bonds.
* @author imasa
*
*/
public class JBondsPanel extends JItemsListPanel implements JContext,JFacetRenderer,JRequester{
private static final long serialVersionUID = 1L;
/**
* The tag of the bond key.
*/
public static final String BOND_KEY="bond key" ;
/**
* The tag of the edge key.
*/
public static final String EDGE_KEY="edge key" ;
public static final String EDGE_LABEL="edge label" ;
/**
* The 'edge' tag .
*/
public static final String EDGE="edge" ;
/**
* The 'edge detail' tag .
*/
public static final String EDGE_DETAIL="edge detail" ;
/**
* The tag of the input node key of the bond.
*/
public static final String BOND_IN_NODE_KEY="bond in node key" ;
/**
* The tag of the output node key of the bond.
*/
public static final String BOND_OUT_NODE_KEY="bond out node key " ;
/**
* The 'new entity' action tag .
*/
public static final String ACTION_NEW_ENTITY="action new entity";
/**
* The tag of the output node key .
*/
public static final String BOND_OUT="out" ;
/**
* The tag of the input node key .
*/
public static final String BOND_IN="in" ;
/**
* The tag of the bond detail entry key .
*/
public static final String BOND_DETAIL_ENTRY_KEY="bond detail entry key";
/**
* The 'select mode' tag.
*/
public static final String SELECT_MODE="select node";
/**
* The 'select mode out' tag.
*/
public static final String SELECT_MODE_OUT="select node out";
/**
* The 'select mode in' tag.
*/
public static final String SELECT_MODE_IN="select node in";
/**
* Indicates the locator type as a bond locator.
*/
public static final String LOCATOR_TYPE_BOND="locator type bond";
String entihome$;
String entityKey$;
String entityLabel$;
JMenuItem[] mia;
String requesterResponseLocator$;
String facetHandlerClass$;
String selectMode$=SELECT_MODE_OUT;
String message$;
Sack entity;
boolean ignoreOutdate=false;
static boolean debug=false;
/**
* The default constructor.
*/
public JBondsPanel() {
super();
}
/**
* Get the context locator.
* @return the context locator.
*/
@Override
public String getLocator() {
Properties locator=new Properties();
locator.setProperty(Locator.LOCATOR_TYPE, JContext.CONTEXT_TYPE);
locator.setProperty(JContext.CONTEXT_TYPE,getType());
locator.setProperty(JItemsListPanel.POSITION,String.valueOf(getPosition()));
if(entihome$!=null){
locator.setProperty(Entigrator.ENTIHOME,entihome$);
//Entigrator entigrator=console.getEntigrator(entihome$);
//String icon$= ExtensionHandler.loadIcon(entigrator,EdgeHandler.EXTENSION_KEY,"bond.png");
//locator.setProperty(Locator.LOCATOR_TITLE, getTitle());
//if(icon$!=null)
// locator.setProperty(Locator.LOCATOR_ICON,icon$);
}
locator.setProperty( Locator.LOCATOR_ICON_CONTAINER, Locator.LOCATOR_ICON_CONTAINER_CLASS);
locator.setProperty( Locator.LOCATOR_ICON_CLASS, getClass().getName());
locator.setProperty( Locator.LOCATOR_ICON_FILE, "bond.png");
locator.setProperty( Locator.LOCATOR_ICON_CLASS_LOCATION,BondDetailHandler.EXTENSION_KEY);
if(entityKey$!=null)
locator.setProperty(EntityHandler.ENTITY_KEY,entityKey$);
if(entityLabel$!=null)
locator.setProperty(EntityHandler.ENTITY_LABEL,entityLabel$);
locator.setProperty(Locator.LOCATOR_TITLE, getTitle());
locator.setProperty(BaseHandler.HANDLER_SCOPE,JConsoleHandler.CONSOLE_SCOPE);
locator.setProperty(BaseHandler.HANDLER_CLASS,getClass().getName());
locator.setProperty(BaseHandler.HANDLER_LOCATION,EdgeHandler.EXTENSION_KEY);
return Locator.toString(locator);
}
/**
* Create the context.
* @param console the main console.
* @param locator$ the locator string.
* @return the procedure context.
*/
@Override
public JContext instantiate(JMainConsole console, String locator$) {
try{
//System.out.println("JBondsPanel:instantiate:BEGIN");
this.console=console;
this.locator$=locator$;
panel.removeAll();
Properties locator=Locator.toProperties(locator$);
entityKey$=locator.getProperty(EntityHandler.ENTITY_KEY);
entihome$=locator.getProperty(Entigrator.ENTIHOME);
entityLabel$=locator.getProperty(EntityHandler.ENTITY_LABEL);
facetHandlerClass$=locator.getProperty(JFacetOpenItem.FACET_HANDLER_CLASS);
Entigrator entigrator=console.getEntigrator(entihome$);
if(Locator.LOCATOR_TRUE.equals(locator.getProperty(JFacetRenderer.ONLY_ITEM)))
return this;
if(entityLabel$==null)
entityLabel$=entigrator.indx_getLabel(entityKey$);
entity=entigrator.getEntityAtKey(entityKey$);
selectMode$=SELECT_MODE_OUT;
if(SELECT_MODE_IN.equals(entity.getElementItemAt("parameter", SELECT_MODE)))
selectMode$=SELECT_MODE_IN;
JItemPanel[] ipa;
// if(BondDetailHandler.class.getName().equals(facetHandlerClass$))
if(entity.getElementItem("fhandler",BondDetailHandler.class.getName())!=null)
ipa=getItemsAtDetail(console, entity);
else
ipa=getItems(console,entity);
if(SELECT_MODE_IN.equals(selectMode$)){
InNodeComparator inc=new InNodeComparator();
inc.entigrator=entigrator;
ArrayList ipl=new ArrayList(Arrays.asList(ipa));
Collections.sort(ipl,inc);
if(ipa!=null)
for(JItemPanel ip:ipl){
panel.add(ip);
}
//revalidate();
//repaint();
}else
putItems(ipa);
//setSelection();
try{
pos=Integer.parseInt(locator.getProperty(POSITION));
if(debug)
System.out.println("JBondsPanel:instantiate:pos="+pos);
select(pos);
}catch(Exception e){
Logger.getLogger(getClass().getName()).info(e.toString());
}
return this;
}catch(Exception e){
Logger.getLogger(getClass().getName()).severe(e.toString());
}
return null;
}
private JItemPanel[] getItems(JMainConsole console,Sack entity){
try{
ArrayListipl=new ArrayList();
Core[] ca=entity.elementGet("bond");
if(ca!=null){
if(debug)
System.out.println("JBondspanel:getItems:bonds="+ca.length);
//ca=Core.sortAtType(ca);
//JBondItem ip;
String ipLocator$;
Properties ipLocator;
//String icon$;
Entigrator entigrator=console.getEntigrator(entihome$);
String outLabel$;
String inLabel$;
String title$;
String edgeLabel$=null;
String edgeKey$;
String bondIcon$= ExtensionHandler.loadIcon(entigrator,EdgeHandler.EXTENSION_KEY,"bond.png");
JBondItem ip=new JBondItem();
if(debug)
System.out.println("JBondspanel:getItems:0");
ipLocator$=ip.getLocator();
if(debug)
System.out.println("JBondspanel:getItems:ip locator="+ipLocator$);
ipLocator=Locator.toProperties(ipLocator$);
for(Core aCa:ca){
try{
outLabel$=null;
inLabel$=null;
ipLocator.setProperty(Entigrator.ENTIHOME, entihome$);
ipLocator.setProperty(EntityHandler.ENTITY_KEY, entityKey$);
if(isEdgeEntity()){
ipLocator.setProperty(EDGE_KEY, entityKey$);
edgeLabel$=entity.getProperty("label");
}else{
edgeKey$=entity.getElementItemAt("edge",aCa.name);
if(edgeKey$!=null){
edgeLabel$=entigrator.indx_getLabel(edgeKey$);
ipLocator.setProperty(EDGE_KEY, entity.getElementItemAt("edge",aCa.name));
}
}
if(aCa.name!=null)
ipLocator.setProperty(BOND_KEY,aCa.name);
if(debug)
System.out.println("JBondspanel:getItems:bond="+aCa.name);
if(aCa.value!=null){
ipLocator.setProperty(BOND_IN_NODE_KEY,aCa.value);
inLabel$=entigrator.indx_getLabel(aCa.value);
}
if(aCa.type!=null){
ipLocator.setProperty(BOND_OUT_NODE_KEY,aCa.type);
outLabel$=entigrator.indx_getLabel(aCa.type);
}
title$=outLabel$+" --("+edgeLabel$+")-> "+inLabel$;
ipLocator.setProperty(Locator.LOCATOR_TITLE, title$);
ipLocator.setProperty(Locator.LOCATOR_TYPE, LOCATOR_TYPE_BOND);
ipLocator.setProperty(Locator.LOCATOR_CHECKABLE, Locator.LOCATOR_TRUE);
ipLocator$=Locator.toString(ipLocator);
if(debug)
System.out.println("JBondspanel:getItems:bond item locator="+ipLocator$);
ip=new JBondItem();
//ip=(JBondItem)constr.newInstance(console,ipLocator$);
ip.setIcon(bondIcon$);
ip.instantiate(console, ipLocator$);
if(debug)
System.out.println("JBondspanel:getItems:bond:2");
ipl.add(ip);
}catch(Exception ee){
Logger.getLogger(JBondsPanel.class.getName()).info(ee.toString());
}
}
}
Collections.sort(ipl,new ItemPanelComparator());
return ipl.toArray(new JBondItem[0]);
}catch(Exception e){
Logger.getLogger(JBondsPanel.class.getName()).severe(e.toString());
}
return null;
}
private JItemPanel[] getItemsAtDetail(JMainConsole console,Sack detail){
try{
ArrayListipl=new ArrayList();
Core[] ca=detail.elementGet("bond");
if(ca!=null){
JBondItem ip;
String ipLocator$;
Properties ipLocator;
//String icon$;
Entigrator entigrator=console.getEntigrator(entihome$);
String outLabel$;
String inLabel$;
String title$;
String edgeKey$;
String bondKey$;
String edgeLabel$;
JBondDetailPanel bdp=new JBondDetailPanel();
ipLocator$=bdp.getLocator();
ipLocator=Locator.toProperties(ipLocator$);
for(Core aCa:ca){
try{
outLabel$=null;
inLabel$=null;
bondKey$=aCa.name;
edgeKey$=detail.getElementItemAt("edge", bondKey$);
ipLocator.setProperty(Entigrator.ENTIHOME, entihome$);
ipLocator.setProperty(EntityHandler.ENTITY_KEY, detail.getKey());
ipLocator.setProperty(EntityHandler.ENTITY_LABEL, detail.getProperty("label"));
ipLocator.setProperty(EDGE_KEY, edgeKey$);
ipLocator.setProperty(BOND_KEY,aCa.name);
// edge=entigrator.getEntityAtKey(edgeKey$);
edgeLabel$=entigrator.indx_getLabel(edgeKey$);
if(aCa.value!=null){
ipLocator.setProperty(BOND_IN_NODE_KEY,aCa.value);
inLabel$=entigrator.indx_getLabel(aCa.value);
}
if(aCa.type!=null){
ipLocator.setProperty(BOND_OUT_NODE_KEY,aCa.type);
outLabel$=entigrator.indx_getLabel(aCa.type);
}
title$=outLabel$+" --("+edgeLabel$+")-> "+inLabel$;
ipLocator.setProperty(Locator.LOCATOR_TITLE, title$);
ipLocator.setProperty(Locator.LOCATOR_TYPE, LOCATOR_TYPE_BOND);
ipLocator.setProperty(Locator.LOCATOR_CHECKABLE, Locator.LOCATOR_TRUE);
ipLocator$=Locator.toString(ipLocator);
if(debug)
System.out.println("JBondsPanel:getItemsAtDetail:ipLocator="+ipLocator$);
ip=new JBondItem();
ip.instantiate(console, ipLocator$);
ipl.add(ip);
}catch(Exception ee){
Logger.getLogger(JBondsPanel.class.getName()).info(ee.toString());
}
}
}
Collections.sort(ipl,new ItemPanelComparator());
return ipl.toArray(new JBondItem[0]);
}catch(Exception e){
Logger.getLogger(JBondsPanel.class.getName()).severe(e.toString());
}
return null;
}
/**
* Get the context menu.
* @return the context menu.
*/
@Override
public JMenu getContextMenu() {
menu=super.getContextMenu();
int cnt=menu.getItemCount();
mia=new JMenuItem[cnt];
for (int i=0;i ipl=new ArrayList(Arrays.asList(ipa));
Collections.sort(ipl,inc);
panel.removeAll();
// System.out.println("JBondsPanel:sort in node:ipl="+ipl.size());
if(ipa!=null)
for(JItemPanel ip:ipl){
panel.add(ip);
}
revalidate();
repaint();
entity=entigrator.getEntityAtKey(entityKey$);
if(!entity.existsElement("parameter"))
entity.createElement("parameter");
entity.putElementItem("parameter", new Core(null,SELECT_MODE,selectMode$));
entigrator.save(entity);
}catch(Exception ee){}
}
} );
menu.add(sortInNode);
JMenuItem sortOutNode = new JMenuItem("Sort out node");
sortOutNode.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
try{
selectMode$=SELECT_MODE_OUT;
JItemPanel[] ipa=getItems();
ArrayList ipl=new ArrayList(Arrays.asList(ipa));
Collections.sort(ipl,new ItemPanelComparator());
panel.removeAll();
System.out.println("JBondsPanel:sort out node:ipl="+ipl.size());
if(ipa!=null)
for(JItemPanel ip:ipl){
panel.add(ip);
}
revalidate();
repaint();
Entigrator entigrator=console.getEntigrator(entihome$);
entity=entigrator.getEntityAtKey(entityKey$);
if(!entity.existsElement("parameter"))
entity.createElement("parameter");
entity.putElementItem("parameter", new Core(null,SELECT_MODE,selectMode$));
entigrator.save(entity);
}catch(Exception ee){}
}
} );
menu.add(sortOutNode);
}
@Override
public void menuDeselected(MenuEvent e) {
}
@Override
public void menuCanceled(MenuEvent e) {
}
});
return menu;
}
/**
* Get context title.
* @return the context title.
*/
@Override
public String getTitle() {
String title$= "Bonds("+entityLabel$+")";
if(message$==null)
return title$;
else
return title$+message$;
//return entityLabel$;
}
/**
* Get context subtitle.
* @return the context subtitle.
*/
@Override
public String getSubtitle() {
return entihome$;
}
/**
* Get context type.
* @return the context type.
*/
@Override
public String getType() {
return "bonds";
}
/**
* Close the context.
*/
@Override
public void close() {
try{
Entigrator entigrator=console.getEntigrator(entihome$);
Sack entity=entigrator.getEntityAtKey(entityKey$);
entigrator.replace(entity);
}catch(Exception e){
Logger.getLogger(getClass().getName()).severe(e.toString());;
}
}
/**
* Response on the call from another context
* @param console the main console
* @param locator$ the locator string
*/
@Override
public void response(JMainConsole console, String locator$) {
// System.out.println("JEdgeEditor:response:"+Locator.remove(locator$,Locator.LOCATOR_ICON ));
try{
Properties locator=Locator.toProperties(locator$);
String action$=locator.getProperty(JRequester.REQUESTER_ACTION);
entihome$=locator.getProperty(Entigrator.ENTIHOME);
Entigrator entigrator=console.getEntigrator(entihome$);
String text$=locator.getProperty(JTextEditor.TEXT);
if(ACTION_NEW_ENTITY.equals(action$)){
Sack newEntity=entigrator.ent_new("edge", text$);
newEntity.createElement("field");
newEntity.putElementItem("field", new Core(null,"Edge",text$));
newEntity.createElement("fhandler");
newEntity.putElementItem("fhandler", new Core(null,EdgeHandler.class.getName(),EdgeHandler.EXTENSION_KEY));
newEntity.putElementItem("fhandler", new Core(null,FieldsHandler.class.getName(),null));
newEntity.createElement("jfacet");
newEntity.putElementItem("jfacet", new Core("gdt.jgui.entity.edge.JEdgeFacetAddItem",EdgeHandler.class.getName(),"gdt.jgui.entity.edge.JEdgeFacetOpenItem"));
newEntity.putAttribute(new Core (null,"icon","edge.png"));
entigrator.save(newEntity);
entigrator.ent_assignProperty(newEntity, "fields", text$);
entigrator.ent_assignProperty(newEntity, "edge", text$);
String icons$=entihome$+"/"+Entigrator.ICONS;
Support.addHandlerIcon(JBondsPanel.class, "edge.png", icons$);
newEntity=entigrator.ent_reindex(newEntity);
reindex(console, entigrator, newEntity);
JEntityFacetPanel efp=new JEntityFacetPanel();
String efpLocator$=efp.getLocator();
efpLocator$=Locator.append(efpLocator$,Locator.LOCATOR_TITLE,newEntity.getProperty("label"));
efpLocator$=Locator.append(efpLocator$, Entigrator.ENTIHOME, entihome$);
efpLocator$=Locator.append(efpLocator$, EntityHandler.ENTITY_KEY, newEntity.getKey());
efpLocator$=Locator.append(efpLocator$, EntityHandler.ENTITY_LABEL, newEntity.getProperty("label"));
JEntityPrimaryMenu.reindexEntity(console, efpLocator$);
Stack s=console.getTrack();
s.pop();
console.setTrack(s);
JConsoleHandler.execute(console, efpLocator$);
return;
}
}catch(Exception e){
Logger.getLogger(getClass().getName()).severe(e.toString());
}
}
/**
* Add edge icon to the locator.
* @param locator$ the locator string.
* @return the locator string.
*
*/
@Override
public String addIconToLocator(String locator$) {
//String icon$=Support.readHandlerIcon(JBondsPanel.class, "edge.png");
/*
try{
Entigrator entigrator=console.getEntigrator(entihome$);
String icon$=ExtensionHandler.loadIcon(entigrator, EdgeHandler.EXTENSION_KEY, "edge.png");
if(icon$!=null)
return Locator.append(locator$, Locator.LOCATOR_ICON,icon$);
}catch(Exception e){
Logger.getLogger(getClass().getName()).severe(e.toString());
}
*/
return locator$;
}
/**
* Get facet handler class name.
* @return the facet handler class name.
*/
@Override
public String getFacetHandler() {
return EdgeHandler.class.getName();
}
/**
* Get the type of the entity for the facet.
* @return the entity type.
*/
@Override
public String getEntityType() {
return "edge";
}
/**
* Get facet icon as a Base64 string.
* @return the icon string.
*/
@Override
public String getCategoryIcon(Entigrator entigrator) {
return ExtensionHandler.loadIcon(entigrator,EdgeHandler.EXTENSION_KEY,"edge.png");
}
/**
* Get category title for entities having the facet type.
* @return the category title.
*/
@Override
public String getCategoryTitle() {
return "Edges";
}
/**
* Adapt cloned entity.
* @param console the main console.
* @param locator$ the locator string.
*/
@Override
public void adaptClone(JMainConsole console, String locator$) {
try{
Properties locator=Locator.toProperties(locator$);
entihome$=locator.getProperty(Entigrator.ENTIHOME);
entityKey$=locator.getProperty(EntityHandler.ENTITY_KEY);
Entigrator entigrator=console.getEntigrator(entihome$);
entity=entigrator.getEntityAtKey(entityKey$);
entigrator.ent_assignProperty(entity,"edge",entity.getProperty("label"));
}catch(Exception e){
Logger.getLogger(JBondsPanel.class.getName()).severe(e.toString());
}
}
/**
* Adapt renamed entity.
* @param console the main console.
* @param locator$ the locator string.
*/
@Override
public void adaptRename(JMainConsole console, String locator$) {
try{
Properties locator=Locator.toProperties(locator$);
entihome$=locator.getProperty(Entigrator.ENTIHOME);
entityKey$=locator.getProperty(EntityHandler.ENTITY_KEY);
Entigrator entigrator=console.getEntigrator(entihome$);
entity=entigrator.getEntityAtKey(entityKey$);
entigrator.ent_assignProperty(entity,"edge",entity.getProperty("label"));
}catch(Exception e){
Logger.getLogger(JBondsPanel.class.getName()).severe(e.toString());
}
}
/**
* No action.
*/
@Override
public void collectReferences(Entigrator entigrator, String entiyKey$, ArrayList sl) {
// TODO Auto-generated method stub
}
/**
* Rebuild entity's facet related parameters.
* @param console the main console
* @param entigrator the entigrator.
* @param entity the entity.
*/
@Override
public void reindex(JMainConsole console, Entigrator entigrator, Sack entity) {
try{
// System.out.println("JContactEditor:reindex:0:entity="+entity.getProperty("label"));
String fhandler$=EdgeHandler.class.getName();
if(entity.getElementItem("fhandler", fhandler$)!=null){
//System.out.println("JContactEditor:reindex:1:entity="+entity.getProperty("label"));
entity.putElementItem("jfacet", new Core(null,fhandler$,JEdgeFacetOpenItem.class.getName()));
entity.putElementItem("fhandler", new Core(null,fhandler$,EdgeHandler.EXTENSION_KEY));
entigrator.save(entity);
}
}catch(Exception e){
Logger.getLogger(getClass().getName()).severe(e.toString());
}
}
/**
* Create a new entity of the facet type.
* @param console the main console.
* @param locator$ the locator string.
* @return the new entity key.
*/
@Override
public String newEntity(JMainConsole console, String locator$) {
JTextEditor textEditor=new JTextEditor();
String editorLocator$=textEditor.getLocator();
editorLocator$=Locator.append(editorLocator$, JTextEditor.TEXT, "Edge"+Identity.key().substring(0,4));
editorLocator$=Locator.append(editorLocator$,Locator.LOCATOR_TITLE,"Edge entity");
JBondsPanel bp=new JBondsPanel();
String bpLocator$=bp.getLocator();
Properties responseLocator=Locator.toProperties(bpLocator$);
entihome$=Locator.getProperty(locator$,Entigrator.ENTIHOME );
if(entihome$!=null){
responseLocator.setProperty(Entigrator.ENTIHOME,entihome$);
// Entigrator entigrator=console.getEntigrator(entihome$);
// String icon$=ExtensionHandler.loadIcon(entigrator, EdgeHandler.EXTENSION_KEY, "edge.png");
// if(icon$!=null)
// editorLocator$=Locator.append(editorLocator$,Locator.LOCATOR_ICON,icon$);
}
responseLocator.setProperty(BaseHandler.HANDLER_CLASS,JBondsPanel.class.getName());
responseLocator.setProperty(BaseHandler.HANDLER_METHOD,"response");
responseLocator.setProperty(BaseHandler.HANDLER_SCOPE,JConsoleHandler.CONSOLE_SCOPE);
responseLocator.setProperty(JRequester.REQUESTER_ACTION,ACTION_NEW_ENTITY);
responseLocator.setProperty(Locator.LOCATOR_TITLE,"Edge");
String responseLocator$=Locator.toString(responseLocator);
//System.out.println("FieldsEditor:newEntity:responseLocator:=:"+responseLocator$);
String requesterResponseLocator$=Locator.compressText(responseLocator$);
editorLocator$=Locator.append(editorLocator$,JRequester.REQUESTER_RESPONSE_LOCATOR,requesterResponseLocator$);
JConsoleHandler.execute(console,editorLocator$);
return editorLocator$;
}
/**
* Remove the bond
* @param console the main console.
* @param locator$ the locator string.
*/
private static void removeBond(JMainConsole console,String locator$){
try{
if(debug)
System.out.println("JBondsPanel:remove bond:locator="+locator$);
Properties locator=Locator.toProperties(locator$);
String edgeKey$=locator.getProperty(EntityHandler.ENTITY_KEY);
String nodeInKey$=locator.getProperty(BOND_IN_NODE_KEY);
String nodeOutKey$=locator.getProperty(BOND_OUT_NODE_KEY);
String bondKey$=locator.getProperty(BOND_KEY);
String entihome$=locator.getProperty(Entigrator.ENTIHOME);
Entigrator entigrator=console.getEntigrator(entihome$);
Sack edge=entigrator.getEntityAtKey(edgeKey$);
edge.removeElementItem("bond", bondKey$);
Sack inNode=entigrator.getEntityAtKey(nodeInKey$);
inNode.removeElementItem("bond", bondKey$);
inNode.removeElementItem("edge", bondKey$);
Sack outNode=entigrator.getEntityAtKey(nodeOutKey$);
outNode.removeElementItem("bond", bondKey$);
outNode.removeElementItem("edge", bondKey$);
entigrator.save(outNode);
entigrator.save(inNode);
entigrator.save(edge);
}catch(Exception e){
Logger.getLogger(JBondsPanel.class.getName()).severe(e.toString());
}
}
private void removeBondEntry(String locator$){
try{
Properties locator=Locator.toProperties(locator$);
String bondKey$=locator.getProperty(BOND_KEY);
Entigrator entigrator=console.getEntigrator(entihome$);
Sack graph=entigrator.getEntityAtKey(entityKey$);
graph.removeElementItem("bond", bondKey$);
entigrator.save(graph);
}catch(Exception e){
Logger.getLogger(JBondsPanel.class.getName()).severe(e.toString());
}
}
private boolean isDetailEntity(){
try{
Entigrator entigrator=console.getEntigrator(entihome$);
Sack entity=entigrator.getEntityAtKey(entityKey$);
if(entity.getProperty("bond.detail")!=null)
return true;
}catch(Exception e){
Logger.getLogger(JBondsPanel.class.getName()).severe(e.toString());
}
return false;
}
private boolean isNodeEntity(){
try{
Entigrator entigrator=console.getEntigrator(entihome$);
Sack entity=entigrator.getEntityAtKey(entityKey$);
if(entity.getProperty("node")!=null)
return true;
}catch(Exception e){
Logger.getLogger(JBondsPanel.class.getName()).severe(e.toString());
}
return false;
}
private boolean isEdgeEntity(){
try{
Entigrator entigrator=console.getEntigrator(entihome$);
Sack entity=entigrator.getEntityAtKey(entityKey$);
if("edge".equals(entity.getProperty("entity")))
return true;
}catch(Exception e){
Logger.getLogger(JBondsPanel.class.getName()).severe(e.toString());
}
return false;
}
private boolean isGraphEntity(){
try{
Entigrator entigrator=console.getEntigrator(entihome$);
Sack entity=entigrator.getEntityAtKey(entityKey$);
if("graph".equals(entity.getProperty("entity")))
return true;
}catch(Exception e){
Logger.getLogger(JBondsPanel.class.getName()).severe(e.toString());
}
return false;
}
private boolean hasBondsToPaste(){
try{
String [] sa=console.clipboard.getContent();
if(sa==null)
return false;
Properties locator;
for(String s:sa){
locator=Locator.toProperties(s);
if(LOCATOR_TYPE_BOND.equals(locator.getProperty(Locator.LOCATOR_TYPE))
&& locator.getProperty(BOND_KEY)!=null)
return true;
}
}catch(Exception e){
Logger.getLogger(JBondsPanel.class.getName()).severe(e.toString());
}
return false;
}
private void pasteBonds(){
try{
String [] sa=console.clipboard.getContent();
if(sa==null)
return;
Properties locator;
Entigrator entigrator=console.getEntigrator(entihome$);
Sack graph=entigrator.getEntityAtKey(entityKey$);
if(!graph.existsElement("bond"))
graph.createElement("bond");
if(!graph.existsElement("edge"))
graph.createElement("edge");
String bondKey$;
String outNode$;
String inNode$;
String edgeKey$;
for(String s:sa){
locator=Locator.toProperties(s);
if(locator==null)
continue;
bondKey$=locator.getProperty(BOND_KEY);
inNode$=locator.getProperty(BOND_IN_NODE_KEY);
outNode$=locator.getProperty(BOND_OUT_NODE_KEY);
edgeKey$=locator.getProperty(EDGE_KEY);
if(LOCATOR_TYPE_BOND.equals(locator.getProperty(Locator.LOCATOR_TYPE))
&&bondKey$ !=null){
graph.putElementItem("bond",new Core(outNode$,bondKey$,inNode$));
graph.putElementItem("edge",new Core(null,bondKey$,edgeKey$));
}
}
entigrator.save(graph);
}catch(Exception e){
Logger.getLogger(JBondsPanel.class.getName()).severe(e.toString());
}
}
private static class InNodeComparator implements Comparator{
public Entigrator entigrator;
@Override
public int compare(JItemPanel o1, JItemPanel o2) {
try{
String l1$=o1.getLocator();
String l2$=o2.getLocator();
String i1$=Locator.getProperty(l1$, BOND_IN_NODE_KEY);
String i2$=Locator.getProperty(l2$, BOND_IN_NODE_KEY);
if(i1$==null&&i2$==null)
return 0;
if(i1$==null||"null".equals(i1$)&&i2$!=null)
return -1;
if(i2$==null||"null".equals(i2$)&&i1$!=null)
return 1;
String t1$=entigrator.indx_getLabel(i1$);
String t2$=entigrator.indx_getLabel(i2$);
// System.out.println("JBondsPanel:InNodeComparator:title 1="+t1$+" 2="+t2$);
return t1$.compareToIgnoreCase(t2$);
}catch(Exception e){
return 0;
}
}
}
@Override
public void activate() {
if(entity==null)
return;
if(ignoreOutdate){
ignoreOutdate=false;
return;
}
Entigrator entigrator=console.getEntigrator(entihome$);
if(!entigrator.ent_outdated(entity))
return;
int n=new ReloadDialog(this).show();
if(2==n){
ignoreOutdate=true;
return;
}
if(1==n){
entity.putAttribute(new Core(null,Entigrator.SAVE_ID,Identity.key()));
entigrator.save(entity);
}
if(0==n){
entity=entigrator.ent_reload(entityKey$);
instantiate(console,getLocator());
}
}
@Override
public String getFacetOpenItem() {
return JEdgeFacetOpenItem.class.getName();
}
@Override
public String getFacetIcon() {
return "bonds.png";
}
private void refresh(){
try{
if(isNodeEntity())
NodeHandler.refresh(console.getEntigrator(entihome$),entityKey$);
if(isDetailEntity())
BondDetailHandler.refresh(console.getEntigrator(entihome$),entityKey$);
}catch(Exception e){
Logger.getLogger(getClass().getName()).severe(e.toString());
}
}
private String[] listNodeLabels(){
try{
Core []ca =entity.elementGet("bond");
Entigrator entigrator=console.getEntigrator(entihome$);
ArrayListsl=new ArrayList();
String label$;
if(ca!=null)
for(Core c:ca){
label$=entigrator.indx_getLabel(c.type);
if(label$!=null&&!sl.contains(label$))
sl.add(label$);
label$=entigrator.indx_getLabel(c.value);
if(label$!=null&&!sl.contains(label$))
sl.add(label$);
}
return sl.toArray(new String[0]);
}catch(Exception e){
Logger.getLogger(getClass().getName()).severe(e.toString());
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy