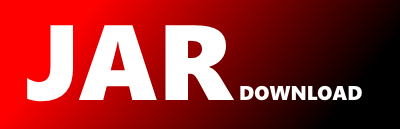
gdt.jgui.entity.graph.JGraphViews Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Graph Show documentation
Show all versions of Graph Show documentation
The graph extension for the entigrator application
The newest version!
package gdt.jgui.entity.graph;
/*
* Copyright 2016 Alexander Imas
* This file is extension of JEntigrator.
JEntigrator is free software: you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
JEntigrator is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with JEntigrator. If not, see .
*/
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Properties;
import java.util.logging.Logger;
import javax.swing.JMenu;
import javax.swing.JMenuItem;
import javax.swing.JOptionPane;
import javax.swing.JPopupMenu;
import javax.swing.event.MenuEvent;
import javax.swing.event.MenuListener;
import gdt.data.entity.BaseHandler;
import gdt.data.entity.EdgeHandler;
import gdt.data.entity.EntityHandler;
import gdt.data.entity.GraphHandler;
import gdt.data.entity.facet.ExtensionHandler;
import gdt.data.grain.Core;
import gdt.data.grain.Identity;
import gdt.data.grain.Locator;
import gdt.data.grain.Sack;
import gdt.data.store.Entigrator;
import gdt.jgui.console.JConsoleHandler;
import gdt.jgui.console.JContext;
import gdt.jgui.console.JItemPanel;
import gdt.jgui.console.JItemsListPanel;
import gdt.jgui.console.JMainConsole;
import gdt.jgui.console.JRequester;
import gdt.jgui.tool.JTextEditor;
public class JGraphViews extends JItemsListPanel implements JRequester{
private static final long serialVersionUID = 1L;
public static final String ACTION_SAVE_VIEW="action save view";
public static final String ACTION_SHOW_VIEW="action show view";
private static final String ACTION_RENAME_VIEW="action rename view";
public static final String VIEW_COMPONENT_KEY="view component key";
public static final String VIEW_KEY="view key";
public static final String VIEW_NAME="view name";
private String entihome$;
private String entityKey$;
private String entityLabel$;
String viewComponentKey$;
String action$;
JMenu menu1;
JPopupMenu popup;
boolean debug=false;
/**
* The default constructor.
*/
public JGraphViews()
{
super();
}
/**
* Get the context locator.
* @return the context locator.
*/
@Override
public String getLocator() {
try{
Properties locator=new Properties();
locator.setProperty(BaseHandler.HANDLER_CLASS,JGraphViews.class.getName());
locator.setProperty(BaseHandler.HANDLER_SCOPE,JConsoleHandler.CONSOLE_SCOPE);
locator.setProperty( JContext.CONTEXT_TYPE,getType());
locator.setProperty(Locator.LOCATOR_TITLE,getTitle());
locator.setProperty(BaseHandler.HANDLER_LOCATION,GraphHandler.EXTENSION_KEY);
if(entityKey$!=null)
locator.setProperty(EntityHandler.ENTITY_KEY,entityKey$);
if(entihome$!=null){
locator.setProperty(Entigrator.ENTIHOME,entihome$);
/*
if(console!=null){
Entigrator entigrator=console.getEntigrator(entihome$);
String icon$= ExtensionHandler.loadIcon(entigrator, GraphHandler.EXTENSION_KEY,"views.png");
if(icon$!=null)
locator.setProperty(Locator.LOCATOR_ICON,icon$);
}
*/
}
locator.setProperty( Locator.LOCATOR_ICON_CONTAINER, Locator.LOCATOR_ICON_CONTAINER_CLASS);
locator.setProperty( Locator.LOCATOR_ICON_CLASS, getClass().getName());
locator.setProperty( Locator.LOCATOR_ICON_FILE, "views.png");
locator.setProperty( Locator.LOCATOR_ICON_CLASS_LOCATION,GraphHandler.EXTENSION_KEY);
if(viewComponentKey$!=null)
locator.setProperty(VIEW_COMPONENT_KEY,viewComponentKey$);
if(action$!=null)
locator.setProperty(JRequester.REQUESTER_ACTION,action$);
return Locator.toString(locator);
}catch(Exception e){
Logger.getLogger(getClass().getName()).severe(e.toString());
return null;
}
}
/**
* Create a new facet renderer.
* @param console the main console.
* @param locator$ the locator string.
* @return the fields editor.
*/
@Override
public JContext instantiate(JMainConsole console, String locator$) {
try{
this.console=console;
this.locator$=locator$;
Properties locator=Locator.toProperties(locator$);
entihome$=locator.getProperty(Entigrator.ENTIHOME);
entityKey$=locator.getProperty(EntityHandler.ENTITY_KEY);
Entigrator entigrator=console.getEntigrator(entihome$);
entityLabel$=entigrator.indx_getLabel(entityKey$);
action$=locator.getProperty(JRequester.REQUESTER_ACTION);
String viewComponentLabel$=entityLabel$+".view";
viewComponentKey$=entigrator.indx_keyAtLabel(viewComponentLabel$);
//initPopup(locator$);
if(viewComponentKey$==null){
Sack viewComponent=entigrator.ent_new("graph.vew", viewComponentLabel$);
viewComponentKey$=viewComponent.getKey();
Sack graph=entigrator.getEntityAtKey(entityKey$);
entigrator.col_addComponent(graph, viewComponent);
}else{
Sack viewComponent=entigrator.getEntityAtKey(viewComponentKey$);
Core[] ca=viewComponent.elementGet("views");
if(ca!=null){
JGraphRenderer gr=new JGraphRenderer();
String gr$=gr.getLocator();
gr$=Locator.append(gr$, Entigrator.ENTIHOME, entihome$);
gr$=Locator.append(gr$, EntityHandler.ENTITY_KEY, entityKey$);
gr$=Locator.append(gr$, VIEW_COMPONENT_KEY, viewComponentKey$);
gr$=Locator.append(gr$, Locator.LOCATOR_CHECKABLE,Locator.LOCATOR_TRUE);
gr$=Locator.append(gr$, JRequester.REQUESTER_ACTION,ACTION_SHOW_VIEW);
ArrayListipl=new ArrayList();
JItemPanel ip;
for(Core c:ca){
gr$=Locator.append(gr$, VIEW_KEY, c.name);
gr$=Locator.append(gr$, VIEW_NAME, c.value);
gr$=Locator.append(gr$, Locator.LOCATOR_TITLE, c.value);
ip=new JItemPanel(console,gr$);
addPopup(ip);
ipl.add(ip);
}
Collections.sort(ipl,new ItemPanelComparator());
putItems(ipl.toArray(new JItemPanel[0]));
return this;
}
}
}catch(Exception e){
Logger.getLogger(getClass().getName()).severe(e.toString());
}
return this;
}
private void addPopup(final JItemPanel ip){
JPopupMenu popup = new JPopupMenu();
JMenuItem renameItem=new JMenuItem("Rename");
popup.add(renameItem);
renameItem.setHorizontalTextPosition(JMenuItem.RIGHT);
renameItem.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
try{
if(debug)
System.out.println("JGraphView:addPopup:locator="+ip.getLocator());
Properties locator=Locator.toProperties(ip.getLocator());
String title$=locator.getProperty(Locator.LOCATOR_TITLE);
locator.setProperty(BaseHandler.HANDLER_CLASS, JGraphViews.class.getName());
locator.setProperty(BaseHandler.HANDLER_METHOD, "response");
locator.setProperty(JRequester.REQUESTER_ACTION, ACTION_RENAME_VIEW);
JTextEditor te=new JTextEditor();
String teLocator$=te.getLocator();
teLocator$=Locator.append(teLocator$, JTextEditor.TEXT, title$);
teLocator$=Locator.append(teLocator$, Entigrator.ENTIHOME, entihome$);
teLocator$=Locator.append(teLocator$,JRequester.REQUESTER_RESPONSE_LOCATOR,Locator.compressText(Locator.toString(locator)));
JConsoleHandler.execute(console, teLocator$);
}catch(Exception ee){
Logger.getLogger(getClass().getName()).info(ee.toString());
}
}
});
ip.setPopupMenu(popup);
}
/**
* Get the context menu.
* @return the context menu.
*/
@Override
public JMenu getContextMenu() {
menu1=new JMenu("Context");
menu=super.getContextMenu();
if(menu!=null){
int cnt=menu.getItemCount();
mia=new JMenuItem[cnt];
for (int i=0;i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy