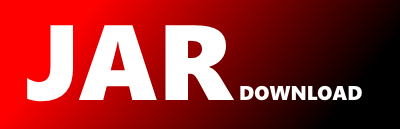
gdt.jgui.console.JItemsListPanel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of JEntigrator Show documentation
Show all versions of JEntigrator Show documentation
The entigrator application
The newest version!
package gdt.jgui.console;
/*
* Copyright 2016 Alexander Imas
* This file is part of JEntigrator.
JEntigrator is free software: you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
JEntigrator is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with JEntigrator. If not, see .
*/
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.AdjustmentEvent;
import java.awt.event.AdjustmentListener;
import java.util.ArrayList;
import java.util.Comparator;
import java.util.logging.Logger;
import javax.swing.JMenu;
import javax.swing.JMenuItem;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JScrollBar;
import javax.swing.BoxLayout;
import javax.swing.JScrollPane;
import gdt.data.grain.Locator;
/**
* This abstract class is a super class for list consoles
* which contains instances of the JItemPanel class.
* @author imasa
*
*/
public abstract class JItemsListPanel extends JPanel implements JContext{
public static final String POSITION="position";
protected JPanel panel;
protected JScrollPane scrollPane;
protected JScrollBar vertical;
protected int pos=-1;
int calls=-1;
/**
* The default constructor.
*/
public JItemsListPanel() {
setLayout(new BoxLayout(this, BoxLayout.Y_AXIS));
scrollPane = new JScrollPane();
add(scrollPane);
panel = new JPanel();
panel.setLayout(new BoxLayout(panel, BoxLayout.Y_AXIS));
scrollPane.getViewport().add(panel);
vertical = scrollPane.getVerticalScrollBar();
vertical.addAdjustmentListener( new AdjustmentListener() {
@Override
public void adjustmentValueChanged(AdjustmentEvent e) {
try{
if(calls++>2){
pos=vertical.getValue();
// System.out.println("JItemsPanel:AdjustmentListener:position="+pos);
return;
}
String position$=Locator.getProperty(locator$, POSITION);
// System.out.println("JItemsListPanel:AdjustmentListener:position given="+pos+" current="+vertical.getValue());
if(position$!=null){
int position=Integer.parseInt(position$);
if(vertical.getValue()!=pos)
vertical.setValue(pos);
}
}catch(Exception ee){
LOGGER.severe(ee.toString());
}
}
});
}
private static final long serialVersionUID = 1L;
private Logger LOGGER=Logger.getLogger(JItemsListPanel.class.getName());
protected JMainConsole console;
protected JMenuItem selectItem;
JMenuItem unselectItem;
JMenuItem recentItem;
JMenuItem countItem;
protected JMenu menu;
protected JMenuItem[] mia;
protected String locator$;
/**
* Get the basic context menu for lists.
*/
@Override
public JMenu getContextMenu() {
menu=new JMenu("Context");
selectItem = new JMenuItem("Select all");
selectItem.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
JItemPanel[] ipa=getItems();
if(ipa!=null){
for(JItemPanel ip:ipa)
ip.setChecked(true);
}
}
} );
menu.add(selectItem);
unselectItem = new JMenuItem("Unselect all");
unselectItem.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
JItemPanel[] ipa=getItems();
if(ipa!=null){
for(JItemPanel ip:ipa)
ip.setChecked(false);
}
}
} );
menu.add(unselectItem);
//
countItem = new JMenuItem("Count all");
countItem.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
int cnt=0;
JItemPanel[] ipa=getItems();
if(ipa!=null)
cnt=ipa.length;
JOptionPane.showMessageDialog(JItemsListPanel.this, String.valueOf(cnt));
}
} );
//
menu.add(countItem);
recentItem = new JMenuItem("Put as recent");
recentItem.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
console.getRecents().put(getTitle(), getLocator());
}
} );
menu.add(recentItem);
return menu;
}
/**
* Put items into list.
* @param ipa the item panel array.
*/
protected void putItems(JItemPanel[] ipa) {
panel.removeAll();
setLayout(new BoxLayout(this, BoxLayout.Y_AXIS));
if(ipa!=null)
for(JItemPanel aIpl:ipa){
panel.add(aIpl);
}
}
protected void clearItems() {
panel.removeAll();
}
/**
* Get items of the list.
* @return the array of the item panels.
*/
public JItemPanel[] getItems(){
try{
int cnt=panel.getComponentCount();
JItemPanel[] ipa=new JItemPanel[cnt];
for(int i=0;isl=new ArrayList();
for(int i=0;i{
@Override
public int compare(JItemPanel o1, JItemPanel o2) {
try{
return o1.getTitle().compareToIgnoreCase(o2.getTitle());
}catch(Exception e){
return 0;
}
}
}
public int getPosition(){
return vertical.getValue();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy