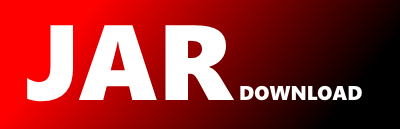
gdt.jgui.console.JMainConsole Maven / Gradle / Ivy
package gdt.jgui.console;
/*
* Copyright 2016 Alexander Imas
* This file is part of JEntigrator.
JEntigrator is free software: you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
JEntigrator is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with JEntigrator. If not, see .
*/
import gdt.data.entity.BaseHandler;
import gdt.data.grain.Locator;
import gdt.data.store.Entigrator;
import gdt.jgui.base.JBaseNavigator;
import gdt.jgui.base.JBasesPanel;
import gdt.jgui.tool.JLocatorDecryptor;
import gdt.jgui.tool.JTextEditor;
import java.awt.Component;
import java.awt.EventQueue;
import javax.swing.JComponent;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JMenuBar;
import javax.swing.JMenuItem;
import javax.swing.AbstractAction;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Hashtable;
import java.util.Map;
import java.util.Properties;
import java.util.Stack;
import java.util.logging.Logger;
import javax.swing.Action;
import javax.swing.JMenu;
import java.awt.BorderLayout;
import java.awt.event.WindowEvent;
import javax.swing.JLabel;
import javax.swing.border.BevelBorder;
import java.awt.Color;
/**
* This is the main application console.
* @author imasa
*
*/
public class JMainConsole {
public final static String ACTION_NEW_BASE="action new base";
private JFrame frmEntigrator;
private Hashtableentigrators;
private Stack track;
private final Action openWorkspace = new OpenWorkspace();
private final Action showTrack = new ShowTrack();
private final Action backTrack = new BackTrack();
private final Action clearTrack = new ClearTrack();
private final Action showClipboard = new ShowClipboard();
private final Action showRecent = new ShowRecent();
private final Action baseNavigator = new BaseNavigator();
private JMenu contextMenu;
private JMenuBar menuBar;
JLabel subtitle;
JMenuItem File;
JMenuItem BaseNavigator;
JMenu trackMenu;
Hashtable recents;
String entihome$;
public String saveId$;
public String outdatedTreatment$;
public JClipboard clipboard=new JClipboard();
static boolean debug=false;
public Mapcache;
/**
* This is the main method which displays the main application console.
* @param args Unused.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
final JMainConsole console = new JMainConsole();
console.frmEntigrator.setVisible(true);
console.entigrators=new Hashtable();
console.setTrack(new Stack());
JTrackPanel.restoreTrack(console);
JClipboard.restore(console);
JRecentPanel.restore(console);
console.frmEntigrator.addWindowListener(new java.awt.event.WindowAdapter() {
public void windowClosing(WindowEvent winEvt) {
// System.out.println("MainConsole:exit:user home="+System.getProperty("user.home") );
JTrackPanel.storeTrack(console);
JClipboard.store(console);
JRecentPanel.store(console);
System.exit(0);
}
public void windowActivated(WindowEvent e) {
try{
if(debug)
System.out.println("MainConsole:activated");
int cnt=console.frmEntigrator.getContentPane().getComponentCount();
if(cnt>0){
JContext current=(JContext)console.frmEntigrator.getContentPane().getComponent(0);
if(current!=null)
if(debug)
System.out.println("MainConsole:activated:context="+current.getTitle());
current.activate();
}
}catch(Exception ee){
Logger.getLogger(getClass().getName()).severe(ee.toString());
}
}
public void windowDeactivated(WindowEvent e){
if(debug)
System.out.println("MainConsole:deactivated.save id="+console.saveId$);
}
});
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* The default constructor.
*/
public JMainConsole() {
initialize();
}
/**
* Set track stack which contains previous shown locators.
* @param track the track stack.
*/
public void setTrack(Stack track){
this.track=track;
}
/**
* Get track stack which contains previous shown locators.
* @return the track stack.
*/
public Stack getTrack(){
if(track==null)
track=new Stack();
return track;
}
public Hashtable getRecents(){
return recents;
}
private void initialize() {
frmEntigrator = new JFrame();
frmEntigrator.setTitle("Entigrator");
frmEntigrator.setBounds(100, 100, 450, 300);
frmEntigrator.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
subtitle = new JLabel("Subtitle");
subtitle.setAlignmentX(Component.RIGHT_ALIGNMENT);
subtitle.setLabelFor(subtitle);
subtitle.setBorder(new BevelBorder(BevelBorder.RAISED));
subtitle.setBackground(Color.WHITE);
subtitle.setForeground(Color.BLACK);
menuBar = new JMenuBar();
frmEntigrator.setJMenuBar(menuBar);
JMenu fileMenu = new JMenu("File");
menuBar.add(fileMenu);
File = new JMenuItem("Bases");
File.setAction(openWorkspace);
fileMenu.add(File);
recents=new Hashtable();
cache=new HashMap();
JMenuItem newBase = new JMenuItem("New base");
newBase.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
JFileChooser chooser = new JFileChooser();
chooser.setCurrentDirectory(new java.io.File(System.getProperty("user.home")));
chooser.setDialogTitle("Select workspace");
chooser.setFileSelectionMode(JFileChooser.DIRECTORIES_ONLY);
chooser.setAcceptAllFileFilterUsed(false);
if (chooser.showOpenDialog(frmEntigrator) == JFileChooser.APPROVE_OPTION) {
String entiroot$=chooser.getSelectedFile().getPath();
Properties locator= new Properties();
locator.setProperty(BaseHandler.ENTIROOT,entiroot$);
locator.setProperty(BaseHandler.HANDLER_SCOPE,JConsoleHandler.CONSOLE_SCOPE);
locator.setProperty(BaseHandler.HANDLER_CLASS,JBasesPanel.class.getName());
locator.setProperty(Entigrator.ENTIHOME,entiroot$+"/New_base");
locator.setProperty(BaseHandler.HANDLER_METHOD,"response");
String locator$=Locator.toString(locator);
JTextEditor textEditor=new JTextEditor();
String editorLocator$=textEditor.getLocator();
Properties editorLocator=Locator.toProperties(editorLocator$);
editorLocator.setProperty(JRequester.REQUESTER_RESPONSE_LOCATOR, Locator.compressText(locator$));
editorLocator.setProperty(JTextEditor.TEXT,"New_base");
editorLocator.setProperty(Entigrator.ENTIHOME,entiroot$+"/New_base");
String teLocator$=Locator.toString(editorLocator);
JConsoleHandler.execute(JMainConsole.this, teLocator$);
}
}
} );
fileMenu.add(newBase);
trackMenu = new JMenu("Track");
menuBar.add(trackMenu);
JMenuItem backItem = new JMenuItem("Back");
backItem.setAction(backTrack);
trackMenu.add(backItem);
trackMenu.addSeparator();
JMenuItem showItem = new JMenuItem("Show");
showItem.setAction(showTrack);
trackMenu.add(showItem);
JMenuItem clipboardItem = new JMenuItem("Clipboard");
clipboardItem.setAction(showClipboard);
trackMenu.add(clipboardItem);
JMenuItem recentItem = new JMenuItem("Recent");
recentItem.setAction(showRecent);
trackMenu.add(recentItem);
JMenuItem navigatorItem = new JMenuItem("Base navigator");
navigatorItem.setAction(baseNavigator);
trackMenu.add(navigatorItem);
trackMenu.addSeparator();
JMenuItem clearItem = new JMenuItem("Clear");
clearItem.setAction(clearTrack);
trackMenu.add(clearItem);
trackMenu.addSeparator();
JMenuItem decryptItem = new JMenuItem("Decrypt locator");
decryptItem.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
JLocatorDecryptor ld=new JLocatorDecryptor();
// JConsoleHandler.execute(JMainConsole.this, ld.getLocator());
JMainConsole.this.putContext(ld,ld.getLocator());
}
} );
trackMenu.add(decryptItem);
}
/**
* Put entigrator into the cache
* @param entigrator theentigrator.
*/
public void putEntigrator(Entigrator entigrator){
if(entigrators==null)
entigrators=new Hashtable();
entigrators.put(entigrator.getEntihome(),entigrator);
}
/**
* Get entigrator.
* @param entihome$ the database directory
* @return the entigrator.
*/
public Entigrator getEntigrator(String entihome$){
try{
Entigrator entigrator= entigrators.get(entihome$);
if(entigrator==null){
entigrator =new Entigrator(new String[]{entihome$});
if(entigrator!=null){
putEntigrator(entigrator);
}
}
return entigrator;
}catch(Exception e){
Logger.getLogger(getClass().getName()).severe(e.toString());
return null;
}
}
private void clearContextMenu(){
int cnt=menuBar.getComponentCount();
ArrayListcl=new ArrayList();
for(int i=0;i0)
JTrackPanel.popMember(JMainConsole.this);
String locator$=JTrackPanel.popMember(JMainConsole.this);
if(locator$!=null){
JConsoleHandler.execute(JMainConsole.this, locator$);
}
}
public JFrame getFrame(){
return frmEntigrator;
}
private class BackTrack extends AbstractAction {
private static final long serialVersionUID = 1L;
public BackTrack() {
putValue(NAME, "Back");
putValue(SHORT_DESCRIPTION, "Previous screen");
}
public void actionPerformed(ActionEvent e) {
try{
if(track.size()>0)
JTrackPanel.popMember(JMainConsole.this);
String locator$=JTrackPanel.popMember(JMainConsole.this);
//System.out.println("JMainConsole:BackTrack:locator="+Locator.remove(locator$, Locator.LOCATOR_ICON));
if(locator$!=null){
JConsoleHandler.execute(JMainConsole.this, locator$);
}
}catch(Exception ee) {
Logger.getLogger(JMainConsole.class.getName()).severe(ee.toString());
}
}
}
private class ClearTrack extends AbstractAction {
private static final long serialVersionUID = 1L;
public ClearTrack() {
putValue(NAME, "Clear");
putValue(SHORT_DESCRIPTION, "Clear track");
}
public void actionPerformed(ActionEvent e) {
JTrackPanel.clearMembers(JMainConsole.this);
frmEntigrator.getContentPane().removeAll();
frmEntigrator.revalidate();
frmEntigrator.repaint();
}
}
private class ShowRecent extends AbstractAction {
private static final long serialVersionUID = 1L;
public ShowRecent() {
putValue(NAME, "Show recent");
putValue(SHORT_DESCRIPTION, "Display recent content");
}
public void actionPerformed(ActionEvent e) {
try{
JRecentPanel recentPanel=new JRecentPanel();
JConsoleHandler.execute(JMainConsole.this, recentPanel.getLocator());
}catch(Exception ee) {
Logger.getLogger(JMainConsole.class.getName()).severe(ee.toString());
}
}
}
private class BaseNavigator extends AbstractAction {
private static final long serialVersionUID = 1L;
public BaseNavigator() {
putValue(NAME, "Base navigator");
putValue(SHORT_DESCRIPTION, "Display base navigator");
}
public void actionPerformed(ActionEvent e) {
try{
if(entihome$==null)
return;
JBaseNavigator navigatorPanel=new JBaseNavigator();
String np$=navigatorPanel.getLocator();
np$=Locator.append(np$, Entigrator.ENTIHOME, entihome$);
JConsoleHandler.execute(JMainConsole.this, np$);
}catch(Exception ee) {
Logger.getLogger(JMainConsole.class.getName()).severe(ee.toString());
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy