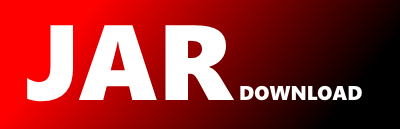
gdt.jgui.entity.bookmark.JBookmarksEditor Maven / Gradle / Ivy
package gdt.jgui.entity.bookmark;
/*
* Copyright 2016 Alexander Imas
* This file is part of JEntigrator.
JEntigrator is free software: you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
JEntigrator is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with JEntigrator. If not, see .
*/
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Properties;
import java.util.Stack;
import java.util.logging.Logger;
import gdt.data.entity.BaseHandler;
import gdt.data.entity.EntityHandler;
import gdt.data.entity.facet.BookmarksHandler;
import gdt.data.entity.facet.FieldsHandler;
import gdt.data.entity.facet.FolderHandler;
import gdt.data.grain.Core;
import gdt.data.grain.Identity;
import gdt.data.grain.Locator;
import gdt.data.grain.Sack;
import gdt.data.grain.Support;
import gdt.data.store.Entigrator;
import gdt.jgui.console.JConsoleHandler;
import gdt.jgui.console.JContext;
import gdt.jgui.console.JFacetRenderer;
import gdt.jgui.console.JItemPanel;
import gdt.jgui.console.JItemsListPanel;
import gdt.jgui.console.JMainConsole;
import gdt.jgui.console.JRequester;
import gdt.jgui.console.ReloadDialog;
import gdt.jgui.entity.JEntitiesPanel;
import gdt.jgui.entity.JEntityPrimaryMenu;
import gdt.jgui.entity.JReferenceEntry;
import gdt.jgui.tool.JIconSelector;
import gdt.jgui.tool.JTextEditor;
import javax.swing.JMenu;
import javax.swing.JMenuItem;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.event.MenuEvent;
import javax.swing.event.MenuListener;
import org.apache.commons.codec.binary.Base64;
/**
* The bookmarks editor context.
* @author imasa
*
*/
public class JBookmarksEditor extends JItemsListPanel implements JFacetRenderer,JRequester{
protected Logger LOGGER=Logger.getLogger(getClass().getName());
/**
* The tag of the bookmark key.
*/
public final static String BOOKMARK_KEY="bookmark key";
private static final String ACTION_CREATE_BOOKMARKS="action create bookmarks";
protected String entihome$;
protected String entityKey$;
protected String entityLabel$;
protected String requesterResponseLocator$;
protected String locator$;
protected JMenuItem[] mia;
int cnt=0;
protected String message$;
protected Sack entity;
boolean debug=false;
boolean ignoreOutdate=false;
private static final long serialVersionUID = 1L;
/**
* The default constructor.
*/
public JBookmarksEditor() {
super();
}
/**
* Get the panel to insert into the main console.
* @return the panel.
*/
@Override
public JPanel getPanel() {
return this;
}
/**
* Get context menu.
* @return the context menu.
*/
@Override
public JMenu getContextMenu() {
menu=super.getContextMenu();
mia=null;
int cnt=menu.getItemCount();
if(cnt>0){
mia=new JMenuItem[cnt];
for(int i=0;icl=new ArrayList();
Entigrator entigrator=console.getEntigrator(entihome$);
entity=entigrator.getEntityAtKey(entityKey$);
Core[] ca=entity.elementGet("jbookmark");
if(ca==null){
entity.createElement("jbookmark");
}else
for(Core aCa:ca)
cl.add(aCa);
String title$;
String bookmarkKey$;
locator$=getLocator();
String requesterResponseLocator$=Locator.compressText(locator$);
for(String aSa:sa){
if(debug)
System.out.println("JBookmarkEditor:paste:="+aSa);
title$=Locator.getProperty(aSa, Locator.LOCATOR_TITLE);
if(title$==null)
continue;
bookmarkKey$=Locator.getProperty(aSa, BOOKMARK_KEY);
if(bookmarkKey$==null){
bookmarkKey$=Identity.key();
aSa=Locator.append(aSa, BOOKMARK_KEY, bookmarkKey$);
aSa=Locator.append(aSa,JRequester.REQUESTER_RESPONSE_LOCATOR, requesterResponseLocator$);
aSa=Locator.append(aSa, Locator.LOCATOR_CHECKABLE, Locator.LOCATOR_TRUE);
}
cl.add( new Core(title$,bookmarkKey$,aSa));
}
ca=cl.toArray(new Core[0]);
entity.elementReplace("jbookmark", ca);
entigrator.ent_alter(entity);
JConsoleHandler.execute(console, getLocator());
}catch(Exception e){
LOGGER.severe(e.toString());
}
}
/**
* Get the context locator.
* @return the context locator.
*/
@Override
public String getLocator() {
try{
Properties locator=new Properties();
locator.setProperty(BaseHandler.HANDLER_CLASS,getClass().getName());
locator.setProperty(BaseHandler.HANDLER_SCOPE,JConsoleHandler.CONSOLE_SCOPE);
locator.setProperty( JContext.CONTEXT_TYPE,getType());
locator.setProperty(Locator.LOCATOR_TITLE,getTitle());
if(entityLabel$!=null){
locator.setProperty(EntityHandler.ENTITY_LABEL,entityLabel$);
}
if(entityKey$!=null)
locator.setProperty(EntityHandler.ENTITY_KEY,entityKey$);
if(entihome$!=null)
locator.setProperty(Entigrator.ENTIHOME,entihome$);
if(entityLabel$!=null)
locator.setProperty(EntityHandler.ENTITY_LABEL,entityLabel$);
locator.setProperty(Locator.LOCATOR_ICON_CONTAINER,Locator.LOCATOR_ICON_CONTAINER_CLASS);
locator.setProperty(Locator.LOCATOR_ICON_CLASS,getClass().getName());
locator.setProperty(Locator.LOCATOR_ICON_FILE,"bookmark.png");
return Locator.toString(locator);
}catch(Exception e){
Logger.getLogger(getClass().getName()).severe(e.toString());
return null;
}
}
/**
* Create the context.
* @param console the main console.
* @param locator$ the locator string.
* @return the bookmarks editor context.
*/
@Override
public JContext instantiate(JMainConsole console, String locator$) {
try{
if(debug)
System.out.println("JBookmarkskEditor.instantiate:locator="+locator$);
this.console=console;
Properties locator=Locator.toProperties(locator$);
entihome$=locator.getProperty(Entigrator.ENTIHOME);
entityKey$=locator.getProperty(EntityHandler.ENTITY_KEY);
Entigrator entigrator=console.getEntigrator(entihome$);
entityLabel$=entigrator.indx_getLabel(entityKey$);
if(Locator.LOCATOR_TRUE.equals(locator.getProperty(JFacetRenderer.ONLY_ITEM)))
return this;
requesterResponseLocator$=locator.getProperty(JRequester.REQUESTER_RESPONSE_LOCATOR);
entity=entigrator.getEntityAtKey(entityKey$);
entityLabel$=entity.getProperty("label");
Core[] ca=entity.elementGet("jbookmark");
Core.sortAtType(ca);
if(ca!=null){
ArrayListipl=new ArrayList();
JBookmarkItem bookmarkItem;
for(Core aCa:ca){
aCa.value=Locator.append(aCa.value, BOOKMARK_KEY, aCa.name);
aCa.value=Locator.append(aCa.value, Locator.LOCATOR_TITLE, aCa.type);
aCa.value=Locator.append(aCa.value, Entigrator.ENTIHOME, entihome$);
if(debug)
System.out.println("JBookmarkskEditor.instantiate:item locator="+aCa.value);
bookmarkItem=new JBookmarkItem(console,aCa.value);
ipl.add(bookmarkItem);
}
Collections.sort(ipl,new ItemPanelComparator());
putItems(ipl.toArray(new JItemPanel[0]));
}else
clearItems();
}catch(Exception e){
Logger.getLogger(getClass().getName()).severe(e.toString());
}
return this;
}
/**
* Get context title.
* @return the context title.
*/
@Override
public String getTitle() {
if(message$==null)
return "Bookmarks";
else
return "Bookmarks"+message$;
//return "Bookmarks";
}
/**
* Get context subtitle.
* @return the context subtitle.
*/
@Override
public String getSubtitle() {
if(entityLabel$!=null)
return entityLabel$;
return entihome$;
}
/**
* Get context type.
* @return the context type.
*/
@Override
public String getType() {
return "bookmarks editor";
}
/**
* Complete the context. No action.
*/
@Override
public void close() {
Entigrator entigrator=console.getEntigrator(entihome$);
entity=entigrator.getEntityAtKey(entityKey$);
}
/**
* Execute the response locator.
* @param console the main console.
* @param locator$ the response locator.
*
*/
@Override
public void response(JMainConsole console, String locator$) {
try{
Properties locator=Locator.toProperties(locator$);
String action$=locator.getProperty(JRequester.REQUESTER_ACTION);
if(ACTION_CREATE_BOOKMARKS.equals(action$)){
String entihome$=locator.getProperty(Entigrator.ENTIHOME);
String entityKey$=locator.getProperty(EntityHandler.ENTITY_KEY);
String text$=locator.getProperty(JTextEditor.TEXT);
Entigrator entigrator=console.getEntigrator(entihome$);
Sack bookmarks=entigrator.ent_new("bookmarks", text$);
bookmarks=entigrator.ent_assignProperty(bookmarks, "bookmarks", bookmarks.getProperty("label"));
bookmarks.putAttribute(new Core(null,"icon","bookmark.png"));
entigrator.ent_alter(bookmarks);
entigrator.saveHandlerIcon(JEntitiesPanel.class, "bookmark.png");
entityKey$=bookmarks.getKey();
JBookmarksEditor be=new JBookmarksEditor();
String beLocator$=be.getLocator();
beLocator$=Locator.append(beLocator$, Entigrator.ENTIHOME, entihome$);
beLocator$=Locator.append(beLocator$, EntityHandler.ENTITY_KEY, entityKey$);
JEntityPrimaryMenu.reindexEntity(console, beLocator$);
Stack s=console.getTrack();
s.pop();
console.setTrack(s);
entigrator.store_replace();
JConsoleHandler.execute(console, beLocator$);
return;
}
String icon$=locator.getProperty(JIconSelector.ICON);
String requesterResponseLocator$=locator.getProperty(JRequester.REQUESTER_RESPONSE_LOCATOR);
byte[] ba=Base64.decodeBase64(requesterResponseLocator$);
String bmLocator$=new String(ba,"UTF-8");
Properties bmLocator=Locator.toProperties(bmLocator$);
String entihome$=bmLocator.getProperty(Entigrator.ENTIHOME);
String entityKey$=bmLocator.getProperty(EntityHandler.ENTITY_KEY);
String bookmarkKey$=locator.getProperty(BOOKMARK_KEY);
String text$=locator.getProperty(JTextEditor.TEXT);
Entigrator entigrator=console.getEntigrator(entihome$);
entity=entigrator.getEntityAtKey(entityKey$);
Core bookmark=entity.getElementItem("jbookmark", bookmarkKey$);
if(JBookmarkItem.ACTION_RENAME.equals(action$)){
bookmark.type=text$;
}
if(JBookmarkItem.ACTION_SET_ICON.equals(action$)){
String bookmarkLocator$=bookmark.value;
bookmarkLocator$=Locator.append(bookmarkLocator$, Locator.LOCATOR_ICON_CONTAINER,Locator.LOCATOR_ICON_CONTAINER_ICONS);
bookmarkLocator$=Locator.append(bookmarkLocator$, Locator.LOCATOR_ICON_FILE,icon$);
bookmark.value= bookmarkLocator$;
}
entity.putElementItem("jbookmark", bookmark);
entigrator.ent_alter(entity);
String bmeLocator$=getLocator();
bmeLocator$=Locator.append(bmeLocator$, Entigrator.ENTIHOME, entihome$);
bmeLocator$=Locator.append(bmeLocator$, EntityHandler.ENTITY_KEY, entityKey$);
JConsoleHandler.execute(console, bmeLocator$);
}catch(Exception e){
LOGGER.severe(e.toString());
}
}
/**
* Add icon string to the locator.
* @param locator$ the origin locator.
* @return the locator.
*/
@Override
public String addIconToLocator(String locator$) {
return locator$;
}
/**
* Get facet handler class name.
* @return the facet handler class name.
*/
@Override
public String getFacetHandler() {
return BookmarksHandler.class.getName();
}
/**
* Get the type of the entity for the facet.
* @return the entity type.
*/
@Override
public String getEntityType() {
return "bookmarks";
}
/**
* Get facet icon as a Base64 string.
* @return the icon string.
*/
@Override
public String getCategoryIcon(Entigrator entigrator) {
return Support.readHandlerIcon(null,JEntitiesPanel.class, "bookmark.png");
}
/**
* Get category title for entities having the facet type.
* @return the category title.
*/
@Override
public String getCategoryTitle() {
return "Bookmarks";
}
/**
* Adapt cloned entity.
* @param console the main console.
* @param locator$ the locator string.
*/
@Override
public void adaptClone(JMainConsole console, String locator$) {
try{
Entigrator entigrator=console.getEntigrator(entihome$);
BookmarksHandler bh=new BookmarksHandler();
String bh$=bh.getLocator();
bh$=Locator.append(bh$, Entigrator.ENTIHOME, entihome$);
bh$=Locator.append(bh$, EntityHandler.ENTITY_KEY, entityKey$);
bh$=Locator.append(bh$, EntityHandler.ENTITY_LABEL, entityLabel$);
bh.instantiate(bh$);
bh.adaptClone(entigrator);
}catch(Exception e){
Logger.getLogger(getClass().getName()).severe(e.toString());
}
}
/**
* Adapt renamed entity.
* @param console the main console.
* @param locator$ the locator string.
*/
@Override
public void adaptRename(JMainConsole console, String locator$) {
try{
Entigrator entigrator=console.getEntigrator(entihome$);
BookmarksHandler bh=new BookmarksHandler();
String bh$=bh.getLocator();
bh$=Locator.append(bh$, Entigrator.ENTIHOME, entihome$);
bh$=Locator.append(bh$, EntityHandler.ENTITY_KEY, entityKey$);
bh$=Locator.append(bh$, EntityHandler.ENTITY_LABEL, entityLabel$);
bh.instantiate(bh$);
bh.adaptRename(entigrator);
}catch(Exception e){
Logger.getLogger(getClass().getName()).severe(e.toString());
}
}
/**
* Rebuild entity's facet related parameters.
* @param console the main console
* @param entigrator the entigrator.
* @param entity the entity.
*/
@Override
public void reindex(JMainConsole console, Entigrator entigrator, Sack entity) {
try{
String bookmarksHandler$=BookmarksHandler.class.getName();
if(entity.getElementItem("fhandler", bookmarksHandler$)==null){
return ;
}
if(entity.getElementItem("jfacet", bookmarksHandler$)==null){
entity.putElementItem("jfacet", new Core(JBookmarksFacetAddItem.class.getName(),bookmarksHandler$,JBookmarksFacetOpenItem.class.getName()));
entigrator.ent_alter(entity);
}
}catch(Exception e){
Logger.getLogger(getClass().getName()).severe(e.toString());
}
}
/**
* Create a new entity of the facet type.
* @param console the main console.
* @param locator$ the locator string.
* @return the new entity key.
*/
@Override
public String newEntity(JMainConsole console, String locator$) {
try{
Properties locator=Locator.toProperties(locator$);
String entihome$=locator.getProperty(Entigrator.ENTIHOME);
JTextEditor textEditor=new JTextEditor();
String teLocator$=textEditor.getLocator();
teLocator$=Locator.append(teLocator$, Entigrator.ENTIHOME,entihome$);
teLocator$=Locator.append(teLocator$, JTextEditor.TEXT_TITLE,"New bookmrks");
String text$="NewBookmarks"+Identity.key().substring(0, 4);
teLocator$=Locator.append(teLocator$, JTextEditor.TEXT,text$);
JBookmarksEditor be=new JBookmarksEditor();
String beLocator$=be.getLocator();
beLocator$=Locator.append(beLocator$, Entigrator.ENTIHOME,entihome$);
beLocator$=Locator.append(beLocator$, EntityHandler.ENTITY_KEY,entityKey$);
beLocator$=Locator.append(beLocator$, BaseHandler.HANDLER_METHOD,"response");
beLocator$=Locator.append(beLocator$, JRequester.REQUESTER_ACTION,ACTION_CREATE_BOOKMARKS);
String requesterResponseLocator$=Locator.compressText(beLocator$);
teLocator$=Locator.append(teLocator$,JRequester.REQUESTER_RESPONSE_LOCATOR,requesterResponseLocator$);
JConsoleHandler.execute(console, teLocator$);
}catch(Exception ee){
LOGGER.severe(ee.toString());
}
return null;
}
/**
* Add referenced entities into the referenced entities list.
* @param entigrator the entigrator.
* @param entityKey$ the entity key.
* @param rel the referenced entities list.
*/
@Override
public void collectReferences(Entigrator entigrator, String entityKey$, ArrayList rel) {
try{
BookmarksHandler bh=new BookmarksHandler();
String entityLocator$=EntityHandler.getEntityLocatorAtKey(entigrator, entityKey$);
if(!bh.isApplied(entigrator, entityLocator$))
return ;
entity=entigrator.getEntityAtKey(entityKey$);
Core[] ca=entity.elementGet("jbookmark");
if(ca!=null){
String referenceKey$;
for(Core c:ca){
try{
referenceKey$=Locator.getProperty(c.value, EntityHandler.ENTITY_KEY);
if(referenceKey$==null||entityKey$.equals(referenceKey$)){
continue;
}
JReferenceEntry.getReference(entigrator,referenceKey$, rel);
}catch(Exception ee){
Logger.getLogger(getClass().getName()).info(ee.toString());
}
}
}
}catch(Exception e){
Logger.getLogger(getClass().getName()).severe(e.toString());
}
}
@Override
public void activate() {
if(debug)
System.out.println("JBookmarksEditor:activate:begin");
if(ignoreOutdate){
ignoreOutdate=false;
return;
}
Entigrator entigrator=console.getEntigrator(entihome$);
if(entity==null)
return;
if(!entigrator.ent_entIsObsolete(entity)){
System.out.println("JBookmarksEditor:activate:up to date");
return;
}
int n=new ReloadDialog(this).show();
if(2==n){
ignoreOutdate=true;
return;
}
if(1==n){
entigrator.ent_alter(entity);
}
if(0==n){
JConsoleHandler.execute(console, getLocator());
}
}
@Override
public String getFacetOpenItem() {
return JBookmarksFacetOpenItem.class.getName();
}
@Override
public String getFacetIcon() {
return "bookmark.png";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy