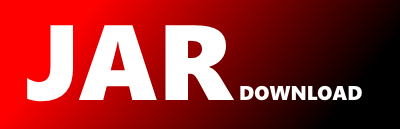
jio.BiLambda Maven / Gradle / Ivy
The newest version!
package jio;
import static java.util.Objects.requireNonNull;
import java.util.function.BiFunction;
import java.util.function.BiPredicate;
/**
* Represents a function that takes two inputs of types 'A' and 'B' and produces an 'IO' effect with a result of type
* 'O'.
*
* @param the type of the first input
* @param the type of the second input
* @param
© 2015 - 2025 Weber Informatics LLC | Privacy Policy