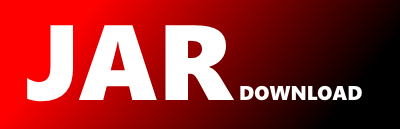
jio.IfElseExp Maven / Gradle / Ivy
The newest version!
package jio;
import static java.util.Objects.requireNonNull;
import java.util.function.BiConsumer;
import java.util.function.Function;
import java.util.function.Predicate;
import java.util.function.Supplier;
import jio.Result.Failure;
import jio.Result.Success;
/**
* Represents an expression that combines a predicate effect with two alternative effect suppliers, one for the
* consequence and another for the alternative branch. If the predicate evaluates to true, the expression is reduced to
* the consequence effect; otherwise, it is reduced to the alternative effect.
*
* @param
© 2015 - 2025 Weber Informatics LLC | Privacy Policy