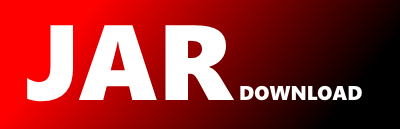
jio.Lambda Maven / Gradle / Ivy
The newest version!
package jio;
import static java.util.Objects.requireNonNull;
import java.util.Objects;
import java.util.function.Function;
import java.util.function.Predicate;
/**
* Represents a function that takes an input and produces an IO effect.
*
* @param the type of the input
* @param
© 2015 - 2025 Weber Informatics LLC | Privacy Policy