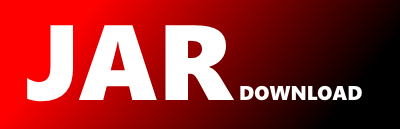
jio.Result Maven / Gradle / Ivy
The newest version!
package jio;
import java.util.concurrent.CompletionException;
import java.util.function.Function;
import java.util.function.Predicate;
/**
* Represents the result of a computation, which can be either a success or a failure. This interface is sealed and
* permits implementations of {@link Result.Success} and {@link Result.Failure}.
*
* @param
© 2015 - 2025 Weber Informatics LLC | Privacy Policy