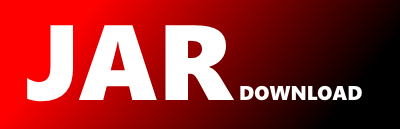
jio.jdbc.QueryStm Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jio-jdbc Show documentation
Show all versions of jio-jdbc Show documentation
Functional and reactive JDBC client in Java
package jio.jdbc;
import jio.BiLambda;
import jio.IO;
import java.time.Duration;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.Executors;
import java.util.function.Function;
/**
* Represents a utility class for executing parameterized SQL queries and processing the results. Optionally integrates
* with Java Flight Recorder for monitoring.
*
*
* Note: The operation is executed on a virtual thread.
*
*
* @param Type of the input parameters for the SQL query.
* @param Type of the objects produced by the result set mapper.
*/
final class QueryStm implements JdbcLambda> {
private final ResultSetMapper mapper;
private final String sql;
private final Function setter;
private final int fetchSize;
private final boolean enableJFR;
/**
* Constructs a {@code QueryStm} with specified parameters.
*
* @param sqlQuery The SQL query to execute.
* @param setter The parameter setter for the SQL query.
* @param mapper The result set mapper for processing query results.
* @param fetchSize The fetch size for the query results.
* @param enableJFR Indicates whether to enable Java Flight Recorder integration.
*/
QueryStm(String sqlQuery, ParamsSetter setter, ResultSetMapper mapper, int fetchSize, boolean enableJFR) {
this.sql = sqlQuery;
this.mapper = mapper;
this.setter = setter;
this.fetchSize = fetchSize;
this.enableJFR = enableJFR;
}
/**
* Applies the specified {@code DatasourceBuilder} to create a lambda function for executing the SQL query.
*
* @param dsb The datasource builder for obtaining database connections.
* @return A lambda function for executing the SQL query and processing results.
*/
@Override
public BiLambda> apply(DatasourceBuilder dsb) {
return (timeout, input) -> {
return IO.task(() -> {
try (var connection = dsb.get().getConnection()) {
try (var ps = connection.prepareStatement(sql)) {
return JfrEventDecorator.decorate(() -> {
var unused = setter.apply(input).apply(ps);
ps.setQueryTimeout((int) timeout.toSeconds());
ps.setFetchSize(fetchSize);
var rs = ps.executeQuery();
List result = new ArrayList<>();
while (rs.next()) result.add(mapper.apply(rs));
return result;
}, sql, enableJFR);
}
}
}, Executors.newVirtualThreadPerTaskExecutor());
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy