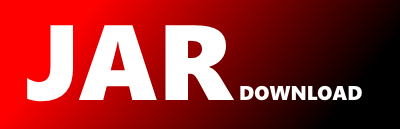
vertx.effect.Val Maven / Gradle / Ivy
Show all versions of vertx-effect Show documentation
package vertx.effect;
import io.vertx.core.AsyncResult;
import io.vertx.core.Future;
import io.vertx.core.Handler;
import java.util.function.*;
/**
A Val is just an alias for a lazy Vertx future. Laziness makes your code more functional and pure.
It allows us to describe programs before executing them. The get method triggers the execution
of the val and returns a future.
@param the type of the value produced by the future */
public interface Val extends Supplier> {
/**
Creates a new value by applying a function to the successful result of this value. If this value
returns an exception then the new value will also contain this exception.
@param fn the function which will be applied to the successful result of this value
@param the type of the returned value
@return a new value
*/
Val
map(final Function fn);
/**
Creates a new value by applying a function to the successful result of this value, and returns
the result of the function as the new value. If this value returns an exception then
the new value will also contain this exception.
@param fn the function which will be applied to the successful result of this value
@param the type of the returned value
@return a new value
*/
Val flatMap(final λ fn);
Val flatMap(final λ successMapper,
final λ failureMapper);
/**
returns a new value tha will retry its execution if it fails
@param attempts the number of attempts before returning an error
@return a new value
*/
Val retry(final int attempts);
/**
returns a new value tha will retry its execution after the an action.
@param attempts the number of attempts before returning an error
@param retryPolicy the function that produces the action to be executed before the retry
@return a new value
*/
Val retry(final int attempts,
final BiFunction> retryPolicy);
/**
returns a new value tha will retry its execution if it fails with an error that
satisfies the given predicate.
@param predicate the predicate against which the returned error will be tested on
@param attempts the number of attempts before returning an error
@return a new value
*/
Val retry(final Predicate predicate,
final int attempts
);
/**
returns a new value tha will retry its execution after an action if it fails with an
error that satisfies the given predicate.
@param predicate the predicate against which the returned error will be tested on
@param attempts the number of attempts before returning an error
@param retryPolicy the function that produces the action to be executed before the retry
@return a new value
*/
Val retry(final Predicate predicate,
final int attempts,
final RetryPolicy retryPolicy);
/**
Creates a new value that will handle any matching throwable that this value might contain.
If there is no match, or if this future contains a valid result then the new future will
contain the same.
@param fn the function to apply if this value fails
@return a new value
*/
Val recover(final Function fn);
/**
Creates a new value that will handle any matching throwable that this value might contain
by assigning it another value.
@param fn the function to apply if this Future fails
@return a new value
*/
Val recoverWith(final λ fn);
Val fallbackTo(final λ fn);
/**
Adds a consumer to be notified of the succeeded result of this value.
@param handler the consumer that will be called with the succeeded result
@return a reference to this value, so it can be used fluently
*/
Val onSuccess(final Consumer handler);
/**
Add a handler to be notified of the result.
@param successHandler the handler that will be called with the succeeded result
@param failureHandler the handler that will be called with the failed result
@return a reference to this, so it can be used fluently
*/
Val onComplete(final Consumer successHandler,
final Consumer failureHandler);
/**
Add a handler to be notified of the result.
@param handler the handler that will be called with the result
@return a reference to this, so it can be used fluently
*/
Val onComplete(final Handler> handler);
/**
returns a new value tha will retry its execution if the result satisfies the given predicate
@param predicate the given predicate
@param attempts the max number of retries
@return a new value
*/
Val retryWhile(final Predicate predicate,
final int attempts);
/**
returns a new value tha will retry its execution after an action if the result satisfies the given predicate
@param predicate the predicate against which the returned error will be tested on
@param attempts the number of attempts before returning an error
@param notExpectedValAction the function that produces the action to be executed before the retry, when the returned value doesn't satify the predicate
@param failureAction the function that produces the action to be executed before the retry, when an error happens
@return a new value
*/
Val retryWhile(final Predicate predicate,
final int attempts,
final RetryPolicy notExpectedValAction,
final RetryPolicy failureAction);
}