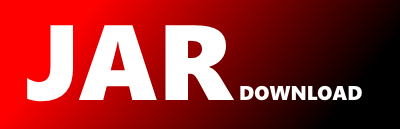
vertx.effect.VertxModule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vertx-effect Show documentation
Show all versions of vertx-effect Show documentation
When actors meet Functional Programming
package vertx.effect;
import io.vertx.core.AbstractVerticle;
import io.vertx.core.DeploymentOptions;
import io.vertx.core.Promise;
import io.vertx.core.eventbus.DeliveryOptions;
import io.vertx.core.eventbus.Message;
import io.vertx.core.eventbus.ReplyException;
import vertx.effect.core.Functions;
import vertx.effect.exp.ListExp;
import vertx.effect.exp.MapExp;
import vertx.effect.exp.Pair;
import java.util.Map;
import java.util.function.Consumer;
import static io.vertx.core.eventbus.ReplyFailure.RECIPIENT_FAILURE;
import static java.util.Objects.requireNonNull;
import static vertx.effect.Failures.EXCEPTION_DEPLOYING_MODULE_CODE;
/**
A module it's a Verticle that when deployed exposes a set of functions that represents other Verticles. Verticles are deployed in the
{@link VertxModule#deploy()} method and then the module functions are initialized in the
{@link VertxModule#initialize()} method.
*/
public abstract class VertxModule extends AbstractVerticle {
private static final DeploymentOptions DEFAULT_DEPLOYMENT_OPTIONS = new DeploymentOptions();
protected final DeploymentOptions deploymentOptions;
private ListExp idValSeq;
private MapExp> refValMap;
private Map> refMap;
@SuppressWarnings({"rawtypes", "unchecked", "squid:S3740"})
private VertxModule(final MapExp refExp,
final DeploymentOptions deploymentOptions) {
this.refValMap = requireNonNull(refExp);
this.deploymentOptions = requireNonNull(deploymentOptions);
idValSeq = ListExp.sequential();
}
/**
Creates an instance of this module
@param options deployments options
*/
@SuppressWarnings("unchecked")
public VertxModule(final DeploymentOptions options) {
this(MapExp.sequential(),
requireNonNull(options)
);
idValSeq = ListExp.sequential();
}
/**
Creates an instance of this module.
{@code
public class MyModule extends VertxModule{...}
MyModule module = new MyModule();
vertx.deployVerticle(module);
}
*/
@SuppressWarnings("unchecked")
public VertxModule() {
this(MapExp.sequential(),
DEFAULT_DEPLOYMENT_OPTIONS
);
idValSeq = ListExp.sequential();
}
/**
override this method to initialize the instance fields of this class that represent the functions exposed by
this module. You can establish a dialogue with the Verticle using
the method {@link VerticleRef#ask()}, which returns a function, or just send it messages without waiting for the
response with the method {@link VerticleRef#tell()}, which returns a consumer. You may be interested in deploying
Verticles on the fly to get a greater level of parallelism with the function {@link VertxRef#spawn(String, λ)}.
*/
protected abstract void initialize();
/**
override this method and deploy the Verticles you want to be exposed by your module.
using the functions {@link VertxModule#deploy(String, λ)} (String, Function)} or
{@link VertxModule#deployConsumer(String, Consumer)}.
You can also deployed regular Verticles with the method {@link VertxModule#deployVerticle(AbstractVerticle)}
*/
protected abstract void deploy();
/**
Factory to deploy or spawn verticles
*/
protected VertxRef vertxRef;
@Override
public void start(final Promise start) {
try {
vertxRef = new VertxRef(requireNonNull(vertx),
deploymentOptions
);
deploy();
Pair.parallel(idValSeq,
refValMap
)
.onComplete(event -> {
if (event.failed()) {
start.fail(event.cause());
}
else {
try {
refMap = event.result()
._2();
initialize();
start.complete();
} catch (Exception e) {
start.fail(new ReplyException(RECIPIENT_FAILURE,
EXCEPTION_DEPLOYING_MODULE_CODE,
Functions.getErrorMessage(e)
));
}
}
})
.get();
} catch (Exception e) {
start.fail(new ReplyException(RECIPIENT_FAILURE,
EXCEPTION_DEPLOYING_MODULE_CODE,
Functions.getErrorMessage(e)
));
}
}
@SuppressWarnings({"unchecked"})
protected λ ask(final String address) {
return ((VerticleRef) refMap.get(requireNonNull(address))).ask();
}
@SuppressWarnings({"unchecked"})
protected λ ask(final String address,
final DeliveryOptions options) {
return ((VerticleRef) refMap.get(requireNonNull(address))).ask(options);
}
@SuppressWarnings({"unchecked"})
protected λc trace(final String address) {
return ((VerticleRef) refMap.get(requireNonNull(address))).trace();
}
@SuppressWarnings({"unchecked"})
protected λc trace(final String address,
final DeliveryOptions options) {
return ((VerticleRef) refMap.get(requireNonNull(address))).trace(options);
}
@SuppressWarnings({"unchecked"})
protected Consumer tell(final String address) {
return ((VerticleRef) refMap.get(requireNonNull(address))).tell();
}
@SuppressWarnings({"unchecked"})
protected Consumer tell(final String address,
final DeliveryOptions options) {
return ((VerticleRef) refMap.get(requireNonNull(address))).tell(options);
}
protected void deployVerticle(final AbstractVerticle verticle) {
idValSeq = idValSeq.append(vertxRef.deployVerticle(requireNonNull(verticle)));
}
protected void deployVerticle(final AbstractVerticle verticle,
final DeploymentOptions options) {
idValSeq = idValSeq.append(vertxRef.deployVerticle(requireNonNull(verticle),
requireNonNull(options)
));
}
protected void deployConsumer(final String address,
final Consumer> consumer) {
Val> exp = vertxRef.deployConsumer(requireNonNull(address),
requireNonNull(consumer),
deploymentOptions
);
refValMap = refValMap.set(address,
requireNonNull(exp)
);
}
protected void deployConsumer(final String address,
final Consumer> consumer,
final DeploymentOptions options) {
Val> exp = vertxRef.deployConsumer(requireNonNull(address),
requireNonNull(consumer),
requireNonNull(options)
);
refValMap = refValMap.set(address,
exp
);
}
protected void deploy(final String address,
final λ lambda) {
Val> exp = vertxRef.deploy(requireNonNull(address),
lambda,
deploymentOptions
);
refValMap = refValMap.set(requireNonNull(address),
exp
);
}
protected void deploy(final String address,
final λc lambda) {
Val> exp = vertxRef.deploy(requireNonNull(address),
lambda,
deploymentOptions
);
refValMap = refValMap.set(requireNonNull(address),
exp
);
}
protected void deploy(final String address,
final λ lambda,
final DeploymentOptions options) {
Val> exp = vertxRef.deploy(requireNonNull(address),
lambda,
options
);
refValMap = refValMap.set(requireNonNull(address),
exp
);
}
protected void deploy(final String address,
final λc lambda,
final DeploymentOptions options) {
Val> exp = vertxRef.deploy(requireNonNull(address),
lambda,
options
);
refValMap = refValMap.set(requireNonNull(address),
exp
);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy