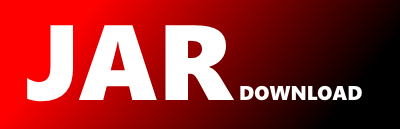
vertx.effect.core.MyVerticle Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vertx-effect Show documentation
Show all versions of vertx-effect Show documentation
When actors meet Functional Programming
package vertx.effect.core;
import io.vertx.core.AbstractVerticle;
import io.vertx.core.Promise;
import io.vertx.core.eventbus.Message;
import io.vertx.core.eventbus.MessageConsumer;
import io.vertx.core.eventbus.ReplyException;
import java.util.function.Consumer;
import static io.vertx.core.eventbus.ReplyFailure.RECIPIENT_FAILURE;
import static java.util.Objects.requireNonNull;
import static vertx.effect.Event.INTERNAL_ERROR_PROCESSING_MESSAGE;
import static vertx.effect.Event.INTERNAL_ERROR_STARTING_VERTICLE;
import static vertx.effect.Failures.*;
/**
Represents a Verticle. It's the unit of computation.
@param type of the message sent to the Verticle */
public class MyVerticle extends AbstractVerticle {
private final Consumer> consumer;
private final String address;
private MessageConsumer
© 2015 - 2025 Weber Informatics LLC | Privacy Policy