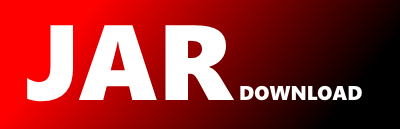
vertx.effect.exp.ParallelJsArrayExp Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vertx-effect Show documentation
Show all versions of vertx-effect Show documentation
When actors meet Functional Programming
package vertx.effect.exp;
import io.vavr.collection.List;
import io.vertx.core.CompositeFuture;
import io.vertx.core.Future;
import jsonvalues.JsArray;
import jsonvalues.JsValue;
import vertx.effect.RetryPolicy;
import vertx.effect.Val;
import java.util.function.BiFunction;
import java.util.function.Predicate;
import java.util.function.Supplier;
/**
Represents a supplier of a completable future which result is a json array. It has the same
recursive structure as a json array. Each index of the array is a completable future that it's
executed asynchronously. When all the futures are completed, all the results are combined into
a json array.
*/
final class ParallelJsArrayExp extends JsArrayExp {
private static final String ATTEMPTS_LOWER_THAN_ONE_ERROR = "attempts < 1";
private List> seq = List.empty();
static final ParallelJsArrayExp EMPTY = new ParallelJsArrayExp();
ParallelJsArrayExp(List> seq) {
this.seq = seq;
}
ParallelJsArrayExp() {
}
@SafeVarargs
ParallelJsArrayExp(final Val extends JsValue> val,
final Val extends JsValue>... others
) {
seq = seq.append(val);
for (Val extends JsValue> other : others) {
seq = seq.append(other);
}
}
/**
it triggers the execution of all the completable futures, combining the results into a JsArray
@return a CompletableFuture of a json array
*/
@Override
@SuppressWarnings({"unchecked", "rawtypes"})
public Future get() {
java.util.List futures = seq.map(Supplier::get)
.toJavaList();
return CompositeFuture.all(futures)
.map(result -> {
java.util.List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy