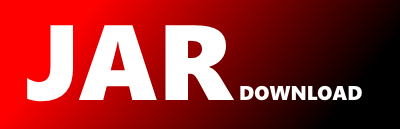
vertx.effect.exp.SequentialAny Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vertx-effect Show documentation
Show all versions of vertx-effect Show documentation
When actors meet Functional Programming
package vertx.effect.exp;
import io.vertx.core.Future;
import vertx.effect.RetryPolicy;
import vertx.effect.Val;
import java.util.List;
import java.util.function.BiFunction;
import java.util.function.Predicate;
import java.util.stream.Collectors;
import static java.util.Objects.requireNonNull;
final class SequentialAny extends Any {
private static final String ATTEMPTS_LOWER_THAN_ONE_ERROR = "attempts < 1";
SequentialAny(final List> exps) {
this.exps = requireNonNull(exps);
}
final List> exps;
@Override
public Val retry(final int attempts) {
if (attempts < 1)
return Cons.failure(new IllegalArgumentException(ATTEMPTS_LOWER_THAN_ONE_ERROR));
return new SequentialAny(exps.stream()
.map(it -> it.retry(attempts))
.collect(Collectors.toList()));
}
@Override
public Val retry(final int attempts,
final BiFunction> retryPolicy) {
if (attempts < 1)
return Cons.failure(new IllegalArgumentException(ATTEMPTS_LOWER_THAN_ONE_ERROR));
if (retryPolicy == null)
return Cons.failure(new NullPointerException("retryPolicy is null"));
return new SequentialAny(exps.stream()
.map(it -> it.retry(attempts,
retryPolicy
)
)
.collect(Collectors.toList()));
}
@Override
public Val retry(final Predicate predicate,
final int attempts) {
if (attempts < 1)
return Cons.failure(new IllegalArgumentException(ATTEMPTS_LOWER_THAN_ONE_ERROR));
if(predicate==null)
return Cons.failure(new NullPointerException("predicate is null"));
return new SequentialAny(exps.stream()
.map(it -> it.retry(predicate,
attempts
))
.collect(Collectors.toList()));
}
@Override
public Val retry(final Predicate predicate,
final int attempts,
final RetryPolicy retryPolicy) {
if (attempts < 1)
return Cons.failure(new IllegalArgumentException(ATTEMPTS_LOWER_THAN_ONE_ERROR));
if (retryPolicy == null)
return Cons.failure(new NullPointerException("retryPolicy is null"));
if(predicate==null)
return Cons.failure(new NullPointerException("predicate is null"));
return new SequentialAny(exps.stream()
.map(it -> it.retry(predicate,
attempts,
retryPolicy
))
.collect(Collectors.toList()));
}
@Override
public Future get() {
return get(exps);
}
private Future get(List> exps) {
if (exps.size() == 1) return exps.get(0)
.get();
else return exps.get(0)
.get()
.flatMap(bool -> {
if (bool) return Future.succeededFuture(true);
else return get(exps.subList(1,
exps.size()
)
);
}
);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy