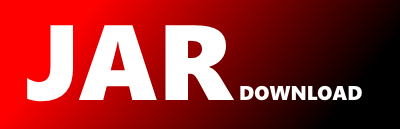
vertx.effect.exp.SequentialJsObj Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vertx-effect Show documentation
Show all versions of vertx-effect Show documentation
When actors meet Functional Programming
package vertx.effect.exp;
import io.vavr.collection.Map;
import io.vavr.collection.Set;
import io.vavr.collection.TreeMap;
import io.vertx.core.Future;
import jsonvalues.JsObj;
import jsonvalues.JsValue;
import vertx.effect.RetryPolicy;
import vertx.effect.Val;
import java.util.function.BiFunction;
import java.util.function.Predicate;
import static java.util.Objects.requireNonNull;
/**
Represents a supplier of a vertx future which result is a json object. It has the same
recursive structure as a json object. Each key has a future associated that it's
executed asynchronously. When all the futures are completed, all the results are combined into
a json object.
*/
final class SequentialJsObj extends JsObjExp {
private static final String ATTEMPTS_LOWER_THAN_ONE_ERROR = "attempts < 1";
Map> bindings = TreeMap.empty();
SequentialJsObj(){}
SequentialJsObj(final Map> bindings) {
this.bindings = bindings;
}
/**
returns a new object future inserting the given future at the given key
@param key the given key
@param future the given future
@return a new JsObjFuture
*/
@Override
public SequentialJsObj set(final String key,
final Val extends JsValue> future
) {
final Map> a = bindings.put(requireNonNull(key),
requireNonNull(future)
);
return new SequentialJsObj(a);
}
/**
it triggers the execution of all the completable futures, combining the results into a JsObj
@return a Future of a json object
*/
@Override
public Future get() {
Set keySet = bindings.keySet();
Future result = Future.succeededFuture(JsObj.empty());
for (final String key : keySet) {
result = result.flatMap(acc -> bindings.get(key)
.getOrElseThrow(IllegalStateException::new)
.get()
.flatMap(val -> Future.succeededFuture(acc.set(key,
val
)
)
)
);
}
return result;
}
@Override
public Val retry(final int attempts) {
if (attempts < 1)
return Cons.failure(new IllegalArgumentException(ATTEMPTS_LOWER_THAN_ONE_ERROR));
return new SequentialJsObj(bindings.mapValues(it -> it.retry(attempts)));
}
@Override
public Val retry(final int attempts,
final BiFunction> retryPolicy) {
if (attempts < 1)
return Cons.failure(new IllegalArgumentException(ATTEMPTS_LOWER_THAN_ONE_ERROR));
if (retryPolicy == null)
return Cons.failure(new NullPointerException("retryPolicy is null"));
return new SequentialJsObj(bindings.mapValues(it -> it.retry(attempts,
retryPolicy
)
));
}
@Override
public Val retry(final Predicate predicate,
final int attempts) {
if (attempts < 1)
return Cons.failure(new IllegalArgumentException(ATTEMPTS_LOWER_THAN_ONE_ERROR));
if (predicate == null)
return Cons.failure(new NullPointerException("predicate is null"));
return new SequentialJsObj(bindings.mapValues(it -> it.retry(predicate,
attempts
)
));
}
@Override
public Val retry(final Predicate predicate,
final int attempts,
final RetryPolicy retryPolicy) {
if (attempts < 1)
return Cons.failure(new IllegalArgumentException(ATTEMPTS_LOWER_THAN_ONE_ERROR));
if (predicate == null)
return Cons.failure(new NullPointerException("predicate is null"));
if (retryPolicy == null)
return Cons.failure(new NullPointerException("retryPolicy is null"));
return new SequentialJsObj(bindings.mapValues(it -> it.retry(predicate,
attempts,
retryPolicy
)
));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy