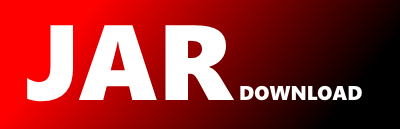
vertx.effect.exp.SequentialSeq Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vertx-effect Show documentation
Show all versions of vertx-effect Show documentation
When actors meet Functional Programming
package vertx.effect.exp;
import io.vertx.core.Future;
import vertx.effect.RetryPolicy;
import vertx.effect.Val;
import javax.naming.OperationNotSupportedException;
import java.util.ArrayList;
import java.util.List;
import java.util.function.BiFunction;
import java.util.function.Predicate;
import static java.util.Objects.requireNonNull;
class SequentialSeq extends ListExp {
@SuppressWarnings("rawtypes")
protected static final ListExp EMPTY = new SequentialSeq<>(io.vavr.collection.List.empty());
SequentialSeq(final io.vavr.collection.List> seq) {
super(seq);
}
@Override
public Val> retry(final int attempts) {
if (attempts < 1)
return Cons.failure(new IllegalArgumentException(ATTEMPTS_LOWER_THAN_ONE_ERROR));
return new SequentialSeq<>(seq.map(it -> it.retry(attempts)));
}
@Override
public Val> retry(final int attempts,
final BiFunction> retryPolicy) {
if (attempts < 1)
return Cons.failure(new IllegalArgumentException(ATTEMPTS_LOWER_THAN_ONE_ERROR));
if (retryPolicy == null)
return Cons.failure(new NullPointerException("retryPolicy is null"));
return new SequentialSeq<>(seq.map(it -> it.retry(attempts,
retryPolicy
)));
}
@Override
public Val> retry(final Predicate predicate,
final int attempts) {
if (attempts < 1)
return Cons.failure(new IllegalArgumentException(ATTEMPTS_LOWER_THAN_ONE_ERROR));
if (predicate == null)
return Cons.failure(new NullPointerException("predicate is null"));
return new SequentialSeq<>(seq.map(it -> it.retry(predicate,
attempts
))
);
}
@Override
public Val> retry(final Predicate predicate,
final int attempts,
final RetryPolicy retryPolicy) {
if (attempts < 1)
return Cons.failure(new IllegalArgumentException(ATTEMPTS_LOWER_THAN_ONE_ERROR));
if (predicate == null)
return Cons.failure(new NullPointerException("predicate is null"));
return new SequentialSeq<>(seq.map(it -> it.retry(predicate,
attempts,
retryPolicy
)));
}
@Override
@SuppressWarnings({"unchecked", "rawtypes"})
public Future> get() {
Future> acc = Future.succeededFuture(new ArrayList<>());
for (final Val extends O> val : seq)
acc = acc.flatMap(l -> val.get()
.map(it -> {
l.add(it);
return l;
}));
return acc;
}
@Override
public ListExp append(final Val extends O> exp) {
return new SequentialSeq<>(seq.append(requireNonNull(exp)));
}
@Override
public ListExp prepend(final Val extends O> exp) {
return new SequentialSeq<>(seq.prepend(requireNonNull(exp)));
}
@Override
public Val race() {
return Cons.failure(new OperationNotSupportedException("race doesn't make any sense in a sequential execution"));
}
@Override
public ListExp tail() {
return new SequentialSeq<>(seq.tail());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy