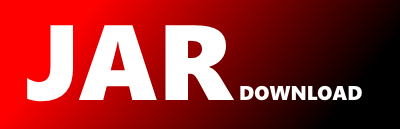
vertx.effect.httpserver.HttpServerBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vertx-effect Show documentation
Show all versions of vertx-effect Show documentation
When actors meet Functional Programming
package vertx.effect.httpserver;
import io.vertx.core.Handler;
import io.vertx.core.Vertx;
import io.vertx.core.http.HttpServer;
import io.vertx.core.http.HttpServerOptions;
import io.vertx.core.http.HttpServerRequest;
import vertx.effect.Val;
import vertx.effect.exp.Cons;
import java.util.Objects;
public class HttpServerBuilder {
private final Vertx vertx;
private final HttpServerOptions options;
private static final String DEFAULT_HOST = "localhost";
private final Handler reqHandler;
public HttpServerBuilder(final Vertx vertx,
final HttpServerOptions options,
final Handler reqHandler) {
this.vertx = Objects.requireNonNull(vertx);
this.options = Objects.requireNonNull(options);
this.reqHandler = Objects.requireNonNull(reqHandler);
}
public HttpServerBuilder(final Vertx vertx,
final Handler reqHandler) {
this(vertx,
new HttpServerOptions().setLogActivity(true),
reqHandler
);
}
public Val startAtRandom(final int start,
final int end) {
return startAtRandom(DEFAULT_HOST,
start,
end
);
}
public Val startAtRandom(final String localhost,
final int start,
final int end) {
if (start > end) return Cons.failure(new IllegalArgumentException("start greater than end"));
return startAtRandomRec(localhost,
start,
end
);
}
private Val startAtRandomRec(final String localhost,
final int start,
final int end) {
if (start == end) return Cons.failure(new IllegalArgumentException("range of ports exhausted"));
return start(localhost,
start
).recoverWith(error -> startAtRandomRec(localhost,
start + 1,
end
)
);
}
public Val start(final String host,
final int port) {
return Cons.of(() -> vertx.createHttpServer(options.setHost(host))
.requestHandler(reqHandler)
.listen(port)
);
}
public Val start(final int port) {
return start(DEFAULT_HOST,
port
);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy