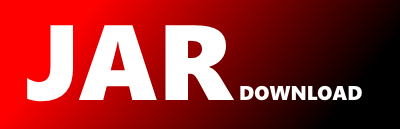
vertx.effect.mock.MockReqResp Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vertx-effect Show documentation
Show all versions of vertx-effect Show documentation
When actors meet Functional Programming
package vertx.effect.mock;
import io.vertx.core.MultiMap;
import io.vertx.core.buffer.Buffer;
import io.vertx.core.http.HttpServerRequest;
import java.util.Objects;
import java.util.function.BiPredicate;
import java.util.function.Function;
import java.util.function.IntFunction;
public class MockReqResp {
public static final BiPredicate ALWAYS = (c, req) -> true;
public static final BiPredicate FIRST_REQ = (c, req) -> c == 1;
public static final BiPredicate SECOND_REQ = (c, req) -> c == 2;
public static final BiPredicate THIRD_REQ = (c, req) -> c == 3;
public static final BiPredicate FORTH_REQ = (c, req) -> c == 4;
public static final IntFunction> REQ_GT =
lowerLimit -> (c, req) -> c > lowerLimit;
public static final IntFunction> REQ_GET =
lowerLimit -> (c, req) -> c >= lowerLimit;
public static final IntFunction> REQ_LT =
upperLimit -> (c, req) -> c < upperLimit;
public static final IntFunction> REQ_LET =
upperLimit -> (c, req) -> c <= upperLimit;
final BiPredicate predicate;
IntFunction>> headers;
IntFunction>> code;
IntFunction>> body;
private MockReqResp(final BiPredicate predicate) {
this.predicate = predicate;
this.headers = MockHeadersResp.EMPTY;
this.code = MockStatusCodeResp._200;
this.body = MockBodyResp.EMPTY;
}
public static MockReqResp when(final BiPredicate predicate) {
return new MockReqResp(predicate);
}
public MockReqResp setHeadersResp(final IntFunction>> headersResp) {
this.headers = Objects.requireNonNull(headersResp);
return this;
}
public MockReqResp setBodyResp(final IntFunction>> bodyResp) {
this.body = Objects.requireNonNull(bodyResp);
return this;
}
public MockReqResp setStatusCodeResp(final IntFunction>> statusCodeResp) {
this.code = Objects.requireNonNull(statusCodeResp);
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy