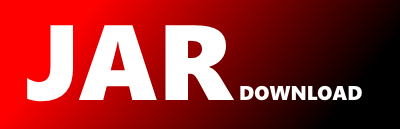
inti.util.Base64OutputByteBuffer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of utils Show documentation
Show all versions of utils Show documentation
An utility library for Inti.
The newest version!
/**
* Copyright 2012 the project-owners
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package inti.util;
import java.io.IOException;
import java.io.OutputStream;
import java.nio.ByteBuffer;
/**
* A fast base64 encoding-OutputStream - not thread-safe.
* Basic Usage:
*
* Base64OutputByteBuffer buffer = new Base64OutputByteBuffer(
* Base64OutputByteBuffer.DEFAULT_ALPHABET,
* Base64OutputByteBuffer.DEFAULT_PADDING,
* Base64OutputByteBuffer.DEFAULT_BUFFER_SIZE);
* ...
* buffer.reset();
* ...
* buffer.write(myData); // x times
* ...
* buffer.flush();
* ...
* ByteBuffer dest = buffer.getByteBuffer();
* ...
*
*/
public class Base64OutputByteBuffer extends OutputStream {
/**
* 64K buffer.
*/
public static final int DEFAULT_BUFFER_SIZE = 65536;
/**
* Default Base64 alphabet (+, /).
*/
public static final String DEFAULT_ALPHABET = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/";
/**
* URL-safe Base64 alphabet (-, _).
*/
public static final String BASE64_URL_ALPHABET = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789-_";
/**
* Default Base64 padding sign (=).
*/
public static final char DEFAULT_PADDING = '=';
/**
* encoding alphabet.
*/
protected char[] alphabet = DEFAULT_ALPHABET.toCharArray();
/**
* Padding sign.
*/
protected byte padding = DEFAULT_PADDING;
/**
* Buffer for the encoded data.
*/
protected ByteBuffer outputBuffer;
/**
* Transfer-buffer for encoding.
*/
protected ByteBuffer inputBuffer = ByteBuffer.wrap(new byte[3]);
public Base64OutputByteBuffer(String alphabet, char padding, int bufferSize) {
if (bufferSize % 4 != 0) {
throw new IllegalArgumentException("bufferSize needs to be restless dividable through 4");
}
this.alphabet = alphabet.toCharArray();
this.padding = (byte) padding;
outputBuffer = ByteBuffer.wrap(new byte[bufferSize]);
}
public ByteBuffer getByteBuffer() {
return outputBuffer;
}
/**
* Clears input- and output-Buffer
*/
public void reset() {
outputBuffer.clear();
inputBuffer.clear();
}
/**
* Fills the input-buffer and eventually encodes it.
*/
@Override
public void write(int b) throws IOException {
inputBuffer.put((byte) b);
if (inputBuffer.position() == inputBuffer.limit()) {
encode();
}
}
/**
* Fills the input-buffer and eventually encodes it.
*/
@Override
public void write(byte[] data, int offset, int length) throws IOException {
int currentOffset = offset, rest, remainingLength = length;
if (inputBuffer.position() != 0) {
for (int i = 0; i < length; i++) {
inputBuffer.put(data[currentOffset++]);
remainingLength--;
if (inputBuffer.position() == inputBuffer.limit()) {
encode();
break;
}
}
}
rest = remainingLength % 3;
remainingLength -= rest;
if (remainingLength >= 3) {
for (int i = 0; i < remainingLength; i += 3, currentOffset += 3) {
inputBuffer.put(data, currentOffset, 3);
encode();
}
}
while (rest-- > 0) {
inputBuffer.put(data[currentOffset++]);
if (inputBuffer.position() == inputBuffer.limit()) {
encode();
}
}
}
/**
* encodes and clears the input buffer.
*/
protected void encode() throws IOException {
int index;
index = (inputBuffer.get(0) & 0xfc) >> 2;
outputBuffer.put((byte) alphabet[index]);
index = (inputBuffer.get(0) & 0x03) << 4 | (inputBuffer.get(1) & 0xf0) >> 4;
outputBuffer.put((byte) alphabet[index]);
index = (inputBuffer.get(1) & 0x0f) << 2 | (inputBuffer.get(2) & 0xc0) >> 6;
outputBuffer.put((byte) alphabet[index]);
index = inputBuffer.get(2) & 0x3f;
outputBuffer.put((byte) alphabet[index]);
inputBuffer.clear();
}
@Override
public void flush() throws IOException {
int index;
if (inputBuffer.position() == 1) {
index = (inputBuffer.get(0) & 0xfc) >> 2;
outputBuffer.put((byte) alphabet[index]);
index = (inputBuffer.get(0) & 0x03) << 4;
outputBuffer.put((byte) alphabet[index]);
outputBuffer.put(padding);
outputBuffer.put(padding);
} else if (inputBuffer.position() == 2) {
index = (inputBuffer.get(0) & 0xfc) >> 2;
outputBuffer.put((byte) alphabet[index]);
index = (inputBuffer.get(0) & 0x03) << 4 | (inputBuffer.get(1) & 0xf0) >> 4;
outputBuffer.put((byte) alphabet[index]);
index = (inputBuffer.get(1) & 0x0f) << 2;
outputBuffer.put((byte) alphabet[index]);
outputBuffer.put(padding);
}
inputBuffer.clear();
outputBuffer.flip();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy