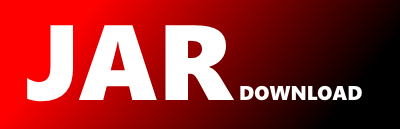
inti.ws.spring.resource.index.IndexConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ws-spring-resource Show documentation
Show all versions of ws-spring-resource Show documentation
An utility library for spring-based web-services for compressing / optimizing JS and CSS resources.
The newest version!
/**
* Copyright 2012 the project-owners
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package inti.ws.spring.resource.index;
import inti.ws.spring.resource.FilteredWebResource;
import inti.ws.spring.resource.config.ConfigParser;
import inti.ws.spring.resource.config.ConfigParserSettings;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import javax.inject.Inject;
import javax.servlet.ServletContext;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.context.annotation.Scope;
import org.springframework.stereotype.Component;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
@Component
@Scope("prototype")
public class IndexConfig implements ConfigParser {
private static final Logger LOGGER = LoggerFactory.getLogger(IndexConfig.class);
private static final Pattern DEPENDENCY_SCANNER = Pattern.compile("\\$(\\w+):(.+)");
public static class IndexBean {
String file;
List dependencies;
Map parameters;
public List getDependencies() {
return dependencies;
}
public void setDependencies(List dependencies) {
this.dependencies = dependencies;
}
public String getFile() {
return file;
}
public void setFile(String file) {
this.file = file;
}
public Map getParameters() {
return parameters;
}
public void setParameters(Map parameters) {
this.parameters = parameters;
}
}
@Inject
ServletContext ctx;
protected ConfigParserSettings settings;
@Override
public Map instanceWebResources(ObjectMapper mapper, JsonNode node) throws Exception {
return parseIndex(mapper.convertValue(node, IndexBean.class));
}
@Override
public void configureWebResources(ObjectMapper mapper, JsonNode node, Map resources,
Map> resourceStack) throws Exception {
IndexBean indexBean = mapper.convertValue(node, IndexBean.class);
Entry entry = resources.entrySet().iterator().next();
FilteredWebResource index = entry.getValue();
Matcher matcher;
String domain;
String resource;
Object value;
if (indexBean.getDependencies() != null) {
for (String dependency : indexBean.getDependencies()) {
matcher = DEPENDENCY_SCANNER.matcher(dependency);
LOGGER.debug("configureWebResources - dependency={}", dependency);
if (matcher.matches()) {
domain = matcher.group(1);
resource = matcher.group(2);
LOGGER.debug("configureWebResources - domain={}, resource={}, resourceStack={}", new Object[] {
domain, resource, resourceStack });
if (resourceStack.containsKey(domain)) {
if (resourceStack.get(domain).containsKey(resource)) {
index.getDependencies().add(resourceStack.get(domain).get(resource));
}
}
}
}
}
if (indexBean.getParameters() != null) {
for (Map.Entry parameter : indexBean.getParameters().entrySet()) {
matcher = DEPENDENCY_SCANNER.matcher(parameter.getValue());
value = "";
if (matcher.matches()) {
domain = matcher.group(1);
resource = matcher.group(2);
if (resourceStack.containsKey(domain)) {
if (resourceStack.get(domain).containsKey(resource)) {
value = resourceStack.get(domain).get(resource);
}
}
} else {
value = parameter.getValue();
}
index.getParameters().put(parameter.getKey(), value);
}
}
LOGGER.debug("configureWebResources - index.getParameters()={}, index.getDependencies()={}",
index.getParameters(), index.getDependencies());
}
protected Map parseIndex(IndexBean indexBean) throws Exception {
FilteredWebResource index;
Map resources = new HashMap<>();
if (indexBean != null) {
index = new FilteredWebResource(ctx, indexBean.getFile(), "/index.html");
resources.put("/index.html", index);
}
return resources;
}
@Override
public ConfigParserSettings getConfigParserSettings() {
return settings;
}
@Override
public void setConfigParserSettings(ConfigParserSettings settings) {
this.settings = settings;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy