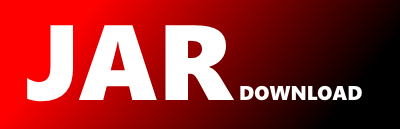
com.ng.gdxutils.model.MoveAlongAction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gdx-utils Show documentation
Show all versions of gdx-utils Show documentation
A java library that used over libGdx game framework.
The newest version!
package com.ng.gdxutils.model;
import com.badlogic.gdx.math.Path;
import com.badlogic.gdx.math.Vector2;
import com.badlogic.gdx.scenes.scene2d.actions.TemporalAction;
import com.badlogic.gdx.utils.Pool;
import com.badlogic.gdx.utils.Pools;
/**
* (c) 2016 Abhishek Aryan
*
* @author Abhishek Aryan
* @since 24-07-2016
*
*/
public class MoveAlongAction extends TemporalAction {
private float x, y;
private boolean rotate;
private Path path;
private Vector2 value = new Vector2();
public Path getPath () {
return path;
}
public void setPath (Path path) {
this.path = path;
}
public boolean isRotate() {
return rotate;
}
public void setRotate (boolean rotate) {
this.rotate = rotate;
}
@Override
protected void begin () {
x = actor.getX();
y = actor.getY();
}
@Override
protected void update (float percent) {
path.valueAt(value, percent);
actor.setPosition(x + value.x, y + value.y);
if (rotate) {
actor.setRotation(path.derivativeAt(value, percent).angle());
}
}
@Override
public void reset () {
super.reset();
path = null;
}
/**Returns a new or pooled MoveAlongAction with the given path and a duration of {@code 1.0}. */
public static MoveAlongAction obtain(Path path) {
return obtain(path, 1.0f);
}
/**Returns a new or pooled MoveAlongAction with the given path and duration. */
public static MoveAlongAction obtain(Path path, float duration) {
Pool pool = Pools.get(MoveAlongAction.class);
MoveAlongAction action = pool.obtain();
action.setDuration(duration);
action.setPath(path);
action.setPool(pool);
return action;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy