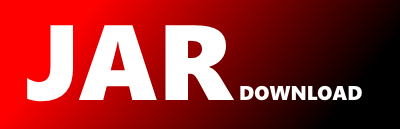
com.itzmeds.adfs.client.response.jwt.ObjectFactory Maven / Gradle / Ivy
Show all versions of adfs-auth-client Show documentation
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.8-b130911.1802
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2018.03.19 at 09:00:41 PM IST
//
package com.itzmeds.adfs.client.response.jwt;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.bind.annotation.adapters.CollapsedStringAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import javax.xml.datatype.XMLGregorianCalendar;
import javax.xml.namespace.QName;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the com.itzmeds.adfs.client.response package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
*
*/
@XmlRegistry
public class ObjectFactory {
private final static QName _KeyType_QNAME = new QName("http://docs.oasis-open.org/ws-sx/ws-trust/200512", "KeyType");
private final static QName _TokenType_QNAME = new QName("http://docs.oasis-open.org/ws-sx/ws-trust/200512", "TokenType");
private final static QName _Address_QNAME = new QName("http://www.w3.org/2005/08/addressing", "Address");
private final static QName _Created_QNAME = new QName("http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-utility-1.0.xsd", "Created");
private final static QName _RequestType_QNAME = new QName("http://docs.oasis-open.org/ws-sx/ws-trust/200512", "RequestType");
private final static QName _Expires_QNAME = new QName("http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-utility-1.0.xsd", "Expires");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: com.itzmeds.adfs.client.response
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link Timestamp }
*
*/
public Timestamp createTimestamp() {
return new Timestamp();
}
/**
* Create an instance of {@link Lifetime }
*
*/
public Lifetime createLifetime() {
return new Lifetime();
}
/**
* Create an instance of {@link BinarySecurityTokenWrapper }
*
*/
public BinarySecurityTokenWrapper createRequestedSecurityToken() {
return new BinarySecurityTokenWrapper();
}
/**
* Create an instance of {@link BinarySecurityToken }
*
*/
public BinarySecurityToken createBinarySecurityToken() {
return new BinarySecurityToken();
}
/**
* Create an instance of {@link RequestSecurityTokenResponseCollection }
*
*/
public RequestSecurityTokenResponseCollection createRequestSecurityTokenResponseCollection() {
return new RequestSecurityTokenResponseCollection();
}
/**
* Create an instance of {@link RequestSecurityTokenResponse }
*
*/
public RequestSecurityTokenResponse createRequestSecurityTokenResponse() {
return new RequestSecurityTokenResponse();
}
/**
* Create an instance of {@link AppliesTo }
*
*/
public AppliesTo createAppliesTo() {
return new AppliesTo();
}
/**
* Create an instance of {@link EndpointReference }
*
*/
public EndpointReference createEndpointReference() {
return new EndpointReference();
}
/**
* Create an instance of {@link Security }
*
*/
public Security createSecurity() {
return new Security();
}
/**
* Create an instance of {@link Header }
*
*/
public Header createHeader() {
return new Header();
}
/**
* Create an instance of {@link Action }
*
*/
public Action createAction() {
return new Action();
}
/**
* Create an instance of {@link Envelope }
*
*/
public Envelope createEnvelope() {
return new Envelope();
}
/**
* Create an instance of {@link Body }
*
*/
public Body createBody() {
return new Body();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/ws-sx/ws-trust/200512", name = "KeyType")
public JAXBElement createKeyType(String value) {
return new JAXBElement(_KeyType_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/ws-sx/ws-trust/200512", name = "TokenType")
public JAXBElement createTokenType(String value) {
return new JAXBElement(_TokenType_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://www.w3.org/2005/08/addressing", name = "Address")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
public JAXBElement createAddress(String value) {
return new JAXBElement(_Address_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link XMLGregorianCalendar }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-utility-1.0.xsd", name = "Created")
public JAXBElement createCreated(XMLGregorianCalendar value) {
return new JAXBElement(_Created_QNAME, XMLGregorianCalendar.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/ws-sx/ws-trust/200512", name = "RequestType")
public JAXBElement createRequestType(String value) {
return new JAXBElement(_RequestType_QNAME, String.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link XMLGregorianCalendar }{@code >}}
*
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-utility-1.0.xsd", name = "Expires")
public JAXBElement createExpires(XMLGregorianCalendar value) {
return new JAXBElement(_Expires_QNAME, XMLGregorianCalendar.class, null, value);
}
}