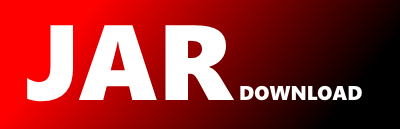
com.itzmeds.adfs.client.response.saml.SignedInfo Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.8-b130911.1802
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2018.03.20 at 12:43:08 PM IST
//
package com.itzmeds.adfs.client.response.saml;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
/**
*
* Java class for anonymous complex type.
*
*
* The following schema fragment specifies the expected content contained within
* this class.
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = { "canonicalizationMethod", "signatureMethod", "reference" })
@XmlRootElement(name = "SignedInfo")
public class SignedInfo {
@XmlElement(name = "CanonicalizationMethod", namespace = "http://www.w3.org/2000/09/xmldsig#", required = true)
protected CanonicalizationMethod canonicalizationMethod;
@XmlElement(name = "SignatureMethod", namespace = "http://www.w3.org/2000/09/xmldsig#", required = true)
protected SignatureMethod signatureMethod;
@XmlElement(name = "Reference", namespace = "http://www.w3.org/2000/09/xmldsig#", required = true)
protected Reference reference;
/**
* Gets the value of the canonicalizationMethod property.
*
* @return possible object is {@link CanonicalizationMethod }
*
*/
public CanonicalizationMethod getCanonicalizationMethod() {
return canonicalizationMethod;
}
/**
* Sets the value of the canonicalizationMethod property.
*
* @param value
* allowed object is {@link CanonicalizationMethod }
*
*/
public void setCanonicalizationMethod(CanonicalizationMethod value) {
this.canonicalizationMethod = value;
}
/**
* Gets the value of the signatureMethod property.
*
* @return possible object is {@link SignatureMethod }
*
*/
public SignatureMethod getSignatureMethod() {
return signatureMethod;
}
/**
* Sets the value of the signatureMethod property.
*
* @param value
* allowed object is {@link SignatureMethod }
*
*/
public void setSignatureMethod(SignatureMethod value) {
this.signatureMethod = value;
}
/**
* Gets the value of the reference property.
*
* @return possible object is {@link Reference }
*
*/
public Reference getReference() {
return reference;
}
/**
* Sets the value of the reference property.
*
* @param value
* allowed object is {@link Reference }
*
*/
public void setReference(Reference value) {
this.reference = value;
}
@Override
public String toString() {
return "SignedInfo [canonicalizationMethod=" + canonicalizationMethod + ", signatureMethod=" + signatureMethod
+ ", reference=" + reference + "]";
}
}