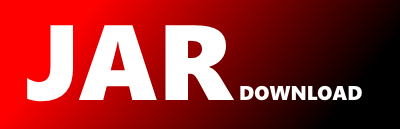
ixa.kaflib.Feature Maven / Gradle / Ivy
package ixa.kaflib;
import java.util.List;
import java.util.ArrayList;
import java.util.HashMap;
import java.io.Serializable;
/** Class for representing features. There are two types of features: properties and categories. */
public class Feature implements Relational, Serializable {
/* Feature's ID (required) */
private String id;
/* Lemma (required) */
private String lemma;
private List> references;
private List externalReferences;
Feature(String id, String lemma, List> references) {
if (references.size() < 1) {
throw new IllegalStateException("Features must contain at least one reference span");
}
if (references.get(0).size() < 1) {
throw new IllegalStateException("Features' reference's spans must contain at least one target");
}
this.id = id;
this.lemma = lemma;
this.references = references;
this.externalReferences = new ArrayList();
}
Feature(Feature feature, HashMap terms) {
this.id = feature.id;
this.lemma = feature.lemma;
/* Copy references */
String id = feature.getId();
this.references = new ArrayList>();
for (Span span : feature.getSpans()) {
/* Copy span */
List targets = span.getTargets();
List copiedTargets = new ArrayList();
for (Term term : targets) {
Term copiedTerm = terms.get(term.getId());
if (copiedTerm == null) {
throw new IllegalStateException("Term not found when copying " + id);
}
copiedTargets.add(copiedTerm);
}
if (span.hasHead()) {
Term copiedHead = terms.get(span.getHead().getId());
this.references.add(new Span(copiedTargets, copiedHead));
}
else {
this.references.add(new Span(copiedTargets));
}
}
/* Copy external references */
this.externalReferences = new ArrayList();
for (ExternalRef externalRef : feature.getExternalRefs()) {
this.externalReferences.add(new ExternalRef(externalRef));
}
}
public boolean isAProperty() {
return this.id.matches("p.*");
}
public boolean isACategory() {
return this.id.matches("c.*");
}
public String getId() {
return this.id;
}
void setId(String id) {
this.id = id;
}
public String getLemma() {
return this.lemma;
}
public void setLemma(String lemma) {
this.lemma = lemma;
}
/** Returns the term targets of the first span. When targets of other spans are needed getReferences() method should be used. */
public List getTerms() {
return this.references.get(0).getTargets();
}
/** Adds a term to the first span. */
public void addTerm(Term term) {
this.references.get(0).addTarget(term);
}
/** Adds a term to the first span. */
public void addTerm(Term term, boolean isHead) {
this.references.get(0).addTarget(term, isHead);
}
public List> getSpans() {
return this.references;
}
public void addSpan(Span span) {
references.add(span);
}
public List getExternalRefs() {
return externalReferences;
}
public void addExternalRef(ExternalRef externalRef) {
externalReferences.add(externalRef);
}
public void addExternalRefs(List externalRefs) {
externalReferences.addAll(externalRefs);
}
public String getSpanStr(Span span) {
String str = "";
for (Term term : span.getTargets()) {
if (!str.isEmpty()) {
str += " ";
}
str += term.getStr();
}
return str;
}
public String getStr() {
return getSpanStr(this.getSpans().get(0));
}
/** Deprecated */
public List> getReferences() {
List> list = new ArrayList>();
for (Span span : this.references) {
list.add(span.getTargets());
}
return list;
}
/** Deprecated */
public void addReference(List span) {
this.references.add(KAFDocument.list2Span(span));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy