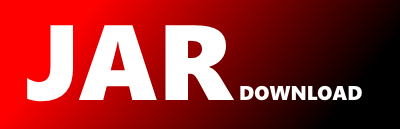
ixa.kaflib.LinkedEntity Maven / Gradle / Ivy
package ixa.kaflib;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
/**
* Linked Entity in the text.
*/
public class LinkedEntity {
/**
* LinkedEntity's ID (required)
*/
private String linkedEntityId;
/**
* LinedEntity's properties
*/
private String resource;
private String reference;
private double confidence;
/**
* Mentions to the same entity (at least one required)
*/
private Span mentions;
LinkedEntity(String linkedEntityId) {
this.linkedEntityId = linkedEntityId;
this.mentions = new Span();
}
LinkedEntity(String linkedEntityId, Span mentions) {
if (mentions.size() < 1) {
throw new IllegalStateException("LinkedEntity must contain at least one reference span");
}
// if (mentions.get(0).size() < 1) {
// throw new IllegalStateException("LinkedEntity' reference's spans must contain at least one target");
// }
this.linkedEntityId = linkedEntityId;
this.mentions = mentions;
}
LinkedEntity(LinkedEntity linkedEntity, HashMap WFs) {
this.linkedEntityId = linkedEntity.linkedEntityId;
this.resource = linkedEntity.resource;
this.reference = linkedEntity.reference;
this.confidence = linkedEntity.confidence;
String id = linkedEntity.getId();
this.mentions = linkedEntity.getWFs();
// for (Span span : linkedEntity.getSpans()) {
// List targets = span.getTargets();
// List copiedTargets = new ArrayList();
// for (WF wf : targets) {
// WF copiedWF = WFs.get(wf.getId());
// if (copiedWF == null) {
// throw new IllegalStateException("Term not found when copying " + id);
// }
// copiedTargets.add(copiedWF);
// }
// if (span.hasHead()) {
// WF copiedHead = WFs.get(span.getHead().getId());
// this.mentions.add(new Span(copiedTargets, copiedHead));
// }
// else {
// this.mentions.add(new Span(copiedTargets));
// }
// }
}
public String getResource() {
return resource;
}
public void setResource(String resource) {
this.resource = resource;
}
public String getReference() {
return reference;
}
public void setReference(String reference) {
this.reference = reference;
}
public double getConfidence() {
return confidence;
}
public void setConfidence(double confidence) {
this.confidence = confidence;
}
public String getId() {
return linkedEntityId;
}
void setId(String id) {
this.linkedEntityId = id;
}
public String getSpanStr() {
String str = "";
for (WF wf : mentions.getTargets()) {
if (!str.isEmpty()) {
str += " ";
}
str += wf.getForm();
}
return str;
}
/**
* Returns the term targets of the first span. When targets of other spans are needed getReferences() method should be used.
*/
public Span getWFs() {
return mentions;
// if (this.mentions.size() > 0) {
// return this.mentions.get(0).getTargets();
// }
// else {
// return null;
// }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy