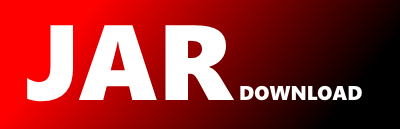
ixa.kaflib.Terminal Maven / Gradle / Ivy
package ixa.kaflib;
import java.util.List;
import java.util.ArrayList;
import java.io.Serializable;
import org.jdom2.Element;
public class Terminal extends TreeNode implements Serializable {
/** The term referenced by this terminal */
private Span span;
Terminal(String id, Span span) {
super(id, false, true);
this.span = span;
}
/** Returns the Span object */
public Span getSpan() {
return this.span;
}
private String getStrValue() {
String str = "";
for (Term term : span.getTargets()) {
if (!str.isEmpty()) {
str += " ";
}
str += term.getStr();
}
return str;
}
public String getStr() {
String strValue = this.getStrValue();
if (strValue.startsWith("-") || strValue.endsWith("-")) {
return strValue.replace("-", "- ");
}
else if (strValue.contains("--")) {
return strValue.replace("--", "-");
}
else {
return strValue;
}
}
public void addChild(TreeNode tn) throws Exception {
throw new Exception("It is not possible to add child nodes to Terminal nodes.");
}
public List getChildren() {
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy