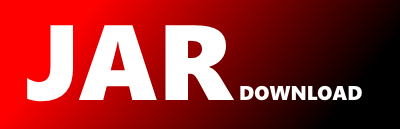
com.github.jackpanz.spring.util.ImageFileLogic Maven / Gradle / Ivy
The newest version!
package com.github.jackpanz.spring.util;
/*
// .::::.
// .::::::::.
// :::::::::::
// ..:::::::::::'
// '::::::::::::'
// .::::::::::
// '::::::::::::::..
// ..::::::::::::.
// ``::::::::::::::::
// ::::``:::::::::' .:::.
// ::::' ':::::' .::::::::.
// .::::' :::: .:::::::'::::.
// .:::' ::::: .:::::::::' ':::::.
// .::' :::::.:::::::::' ':::::.
// .::' ::::::::::::::' ``::::.
// ...::: ::::::::::::' ``::.
// ```` ':. ':::::::::' ::::..
// '.:::::' ':'````..
//
*/
import com.github.jackpanz.spring.initialization.ConstantsConfigurer;
import org.apache.commons.lang3.StringUtils;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.springframework.web.multipart.MultipartFile;
import java.io.IOException;
import java.io.InputStream;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.List;
import java.util.UUID;
/**
* Created by Administrator on 2016/2/23.
*/
public class ImageFileLogic {
protected final Log logger = LogFactory.getLog(getClass());
private static SimpleDateFormat simpleDateFormat = new SimpleDateFormat("yyyyMMdd");
private MultipartFile uploadFile;
private String oldImageName = null;
private String imageName = null;
private boolean isSuccessUpload = false;
public String parentFolder;
public static String type;
public void setSuccessUpload(boolean successUpload) {
isSuccessUpload = successUpload;
}
public boolean getSuccessUpload() {
return isSuccessUpload;
}
public String getImageName() {
return parentFolder + "/" + imageName;
}
/***
* type:用於標誌圖片屬於哪個模块
* */
@Deprecated
public ImageFileLogic(MultipartFile uploadFile, String oldImageName, String parentFolder) {
this.uploadFile = uploadFile;
this.oldImageName = oldImageName;
this.parentFolder = parentFolder;
}
public static void copyOSS(String sourceKey, String destinationKey) {
OSSUtils.copyOSS(sourceKey, destinationKey);
}
public static boolean existObject(String ssoKeyName) {
return OSSUtils.existObject(ssoKeyName);
}
public static void uploadToOSS(String ssoKeyName, InputStream inputStream) {
OSSUtils.uploadToOSS(ssoKeyName, inputStream);
}
public static void deleteFileToOSS(String ssoKeyName) {
OSSUtils.deleteFileToOSS(ssoKeyName);
}
public static void deleteFileToOSS(List ssoKeyNames) {
OSSUtils.deleteFileToOSS(ssoKeyNames);
}
public void deteleOldFile() {
if (isSuccessUpload && StringUtils.isNotEmpty(oldImageName)) {
OSSUtils.deleteFileToOSS(parentFolder + "/" + oldImageName);
}
}
public static String uploadImage(MultipartFile uploadFile) {
if (uploadFile == null || uploadFile.isEmpty()) {
return null;
}
String imageName = null;
if (uploadFile.getContentType().indexOf("jpeg") > -1) {
imageName = "images/" + getNewImageJPGName();
} else if (uploadFile.getOriginalFilename().toLowerCase().indexOf("jpg") > -1) {
imageName = "images/" + getNewImageJPGName();
} else if (uploadFile.getOriginalFilename().toLowerCase().indexOf("png") > -1) {
imageName = "images/" + getNewImagePNGName();
} else if (uploadFile.getContentType().indexOf("png") > -1) {
imageName = "images/" + getNewImagePNGName();
} else if (uploadFile.getContentType().indexOf("mp4") > -1) {
imageName = "videos/" + getNewMp4Name();
}else {
return null;
}
try {
uploadImage(imageName, uploadFile);
return imageName;
} catch (Exception ex) {
ex.printStackTrace();
return null;
}
}
public static void uploadImage(String imageName, MultipartFile uploadFile) {
try {
OSSUtils.uploadToOSS(imageName, uploadFile.getInputStream());
} catch (IOException e) {
e.printStackTrace();
}
}
public boolean copyNewFile() {
try {
if (uploadFile != null && !uploadFile.isEmpty()) {
if (uploadFile.getContentType().indexOf("jpeg") > -1) {
imageName = getNewImageJPGName();
} else if (uploadFile.getContentType().indexOf("png") > -1) {
imageName = getNewImagePNGName();
} else {
return false;
}
uploadToOSS(parentFolder + "/" + imageName, uploadFile.getInputStream());
isSuccessUpload = true;
return true;
}
} catch (Exception e) {
e.printStackTrace();
}
return false;
}
public static String getNewImageJPGName() {
Date now = new Date();
return simpleDateFormat.format(now) + "/" + UUID.randomUUID().toString().replaceAll("-", "") + ".jpg";
}
public static String getNewImagePNGName() {
Date now = new Date();
return simpleDateFormat.format(now) + "/" + UUID.randomUUID().toString().replaceAll("-", "") + ".png";
}
public static String getNewMp4Name() {
Date now = new Date();
return simpleDateFormat.format(now) + "/" + UUID.randomUUID().toString() + ".mp4";
}
/**
* 將臨時文件中的文件複製到正式目錄下
*/
public static String copy(String fileName, String prefix) throws RuntimeException //文件名稱必須以prefix開頭
{
if (StringUtils.isBlank(fileName))
throw new MException("文件名稱不能為空");
if (StringUtils.isBlank(prefix))
throw new MException("請指定文件前綴");
if (ImageFileLogic.existObject(fileName)) {//如果存在該臨時文件則繼續
String odlName = fileName;
try {
fileName = fileName.replace("temp/", prefix);// 更換可臨時文件的名稱
} catch (Exception e) {
throw new MException("您的附件名稱錯誤");
}
String to_object_key = ConstantsConfigurer.image_uload + "/" + fileName;// 正式文件目錄
ImageFileLogic.copyOSS(odlName, to_object_key);// 將臨時文件拷貝為正式文件
ImageFileLogic.deleteFileToOSS(odlName);// 刪除臨時文件
return fileName;
} else
throw new MException("阿裏雲中沒有找到您的附件");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy