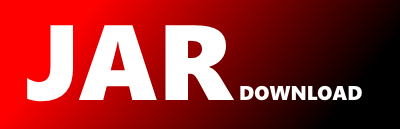
com.github.jackpanz.spring.util.ResultMap Maven / Gradle / Ivy
The newest version!
package com.github.jackpanz.spring.util;
import org.apache.commons.lang3.StringUtils;
import java.io.Serializable;
import java.util.HashMap;
import java.util.Map;
/**
* Created by Administrator on 2016/3/1.
*/
public class ResultMap extends HashMap implements Map, Cloneable, Serializable {
public static int register_timeout = 1001;
public static String DEFAULT_FAIL_MSG = "操作失败";
public static String DEFAULT_SUCCESS_MSG = "操作成功";
private static final long serialVersionUID = -6084968393280233251L;
public static String SET_PAGING_LISTENER = "com.github.jackpanz.spring.util.LayuiPagingListener";
public ResultMap() {
setFail();
}
public ResultMap(boolean isSuccess) {
setActions(isSuccess);
}
public ResultMap(boolean isSuccess, String msg) {
setActions(isSuccess, msg);
}
public ResultMap(boolean isSuccess, String msg, String comd) {
setActions(isSuccess, msg, null, comd, 0, null);
}
public ResultMap(boolean isSuccess, String msg, String comd, int errorCode) {
setActions(isSuccess, msg, null, comd, errorCode, null);
}
public ResultMap(boolean isSuccess, String msg, String comd, int errorCode, Exception e) {
setActions(isSuccess, msg, null, comd, errorCode, e);
}
public ResultMap setActions(boolean action) {
return setActions(action, null, null, null, 0, null);
}
public ResultMap setActions(boolean action, String message) {
return setActions(action, message, null, null, 0, null);
}
public ResultMap setActions(boolean action, Object data) {
return setActions(action, null, data, null, 0, null);
}
public ResultMap setActions(boolean action, String message, String comd) {
return setActions(action, message, null, comd, 0, null);
}
public ResultMap setActions(boolean action, String message, Object data) {
return setActions(action, message, data, null, 0, null);
}
public ResultMap setActions(boolean action, String message, Object data, String comd) {
return setActions(action, message, data, comd, 0, null);
}
public ResultMap setActions(boolean action, String message, Object data, String comd, int errorCode, Exception e) {
if (action) {
setSuccess(message == null ? DEFAULT_SUCCESS_MSG : message, data, comd);
} else {
setFail(message == null ? DEFAULT_FAIL_MSG : message, null, errorCode, e);
}
return this;
}
public boolean getAction() {
return (boolean) get("action");
}
public ResultMap setAction(boolean action) {
put("action", action);
return this;
}
public ResultMap setData(Object data) {
put("data", data);
return this;
}
public ResultMap setSuccess() {
put("action", true);
setMsg(DEFAULT_SUCCESS_MSG);
return this;
}
public ResultMap setSuccess(String msg) {
put("action", true);
setMsg(msg);
return this;
}
public ResultMap setSuccess(Object data) {
put("action", true);
setMsg(DEFAULT_SUCCESS_MSG);
setData(data);
return this;
}
public ResultMap setSuccess(String msg, Object data) {
put("action", true);
setMsg(msg);
setData(data);
return this;
}
public ResultMap setSuccess(String msg, String comd) {
put("action", true);
setMsg(msg);
setComd(comd);
return this;
}
public ResultMap setSuccess(String msg, Object data, String comd) {
put("action", true);
setMsg(msg);
setComd(comd);
setData(data);
return this;
}
public ResultMap setFail() {
setAction(false);
setMsg(DEFAULT_FAIL_MSG);
return this;
}
public ResultMap setFail(String msg) {
setAction(false);
setMsg(msg);
return this;
}
public ResultMap setFail(String msg, int errorCode) {
setAction(false);
setMsg(msg);
setErrorCode(errorCode);
return this;
}
public ResultMap setFail(String msg, Object data, int errorCode, Exception e) {
setAction(false);
setMsg(msg);
setData(data);
setErrorCode(errorCode);
setException(e);
return this;
}
public ResultMap setComd(String comd) {
put("comd", StringUtils.defaultIfBlank(comd, "NONE"));
return this;
}
public ResultMap setMsg(String msg) {
put("msg", msg);
return this;
}
public ResultMap setErrorCode(Integer errorCode) {
put("errorCode", errorCode);
return this;
}
public ResultMap setException(Exception e) {
put("exception", e);
return this;
}
public ResultMap setPageResult(Object page, SetPagingListener setPagingListener) {
setPagingListener.setPageResult(this, page);
return this;
}
public ResultMap setPageResult(Object page) {
try {
SetPagingListener setPagingListener = (SetPagingListener) Class.forName(SET_PAGING_LISTENER).newInstance();
setPageResult(page, setPagingListener);
} catch (InstantiationException e) {
e.printStackTrace();
} catch (IllegalAccessException e) {
e.printStackTrace();
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy