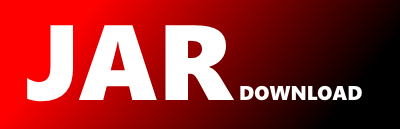
com.maccloud.util.BeanUtils Maven / Gradle / Ivy
//package com.maccloud.util;
//
//import com.maccloud.basic.BasicEntity;
//import com.maccloud.basic.BasicExample;
//import com.maccloud.basic.BasicMapper;
//import org.apache.commons.lang3.math.NumberUtils;
//import org.slf4j.Logger;
//import org.slf4j.LoggerFactory;
//
//import javax.servlet.ServletRequest;
//import java.lang.reflect.InvocationTargetException;
//import java.lang.reflect.Method;
//import java.text.ParseException;
//import java.text.SimpleDateFormat;
//import java.util.*;
//
///**
// * Created by Administrator on 2016/2/22.
// */
//public class BeanUtils {
//
// private final static Logger logger = LoggerFactory.getLogger(BeanUtils.class);
//
// public static final Map, TableProperty> entityInfos = new HashMap, TableProperty>();
//
// public static final Map> names = new HashMap>();
//
// public static final SimpleDateFormat simpleDateFormat1 = new SimpleDateFormat("yyyy-MM-dd");
//
// public static final SimpleDateFormat simpleDateFormat2 = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
//
// public static final String regex1 = "^[0-9]{4}-[0-9]{2}-[0-9]{2}$";
//
// public static final String regex2 = "^[0-9]{4}-[0-9]{2}-[0-9]{2} [0-9]{2}:[0-9]{2}:[0-9]{2}$";
//
//// public static String getColumn(Class basicEntityClazz, String property) {
//// return entityInfos.get(basicEntityClazz).fieldPropertyMap.get(property).column;
//// }
////
//// public static String getColumn(String entity, String property) {
//// return getColumn(getEntity(entity), property);
//// }
//
//// public static String getTable(Class basicEntityClazz) {
//// return entityInfos.get(basicEntityClazz).tableName;
//// }
////
//// public static String getTable(String entity) {
//// return entityInfos.get(getEntity(entity)).tableName;
//// }
//
// public static Object getProperty(Object entity , Method getMethod){
// try {
// return getMethod.invoke(entity,null);
// } catch (IllegalAccessException e) {
// e.printStackTrace();
// } catch (InvocationTargetException e) {
// e.printStackTrace();
// }
// return null;
// }
//
// public static void setProperty(Object entity ,Object value ,Method setMethod){
// try {
// setMethod.invoke(entity,value);
// } catch (IllegalAccessException e) {
// e.printStackTrace();
// } catch (InvocationTargetException e) {
// e.printStackTrace();
// }
// }
//
// public static FieldProperty getPrimaryKey(Class basicEntityClazz) {
// return entityInfos.get(basicEntityClazz).primaryKey;
// }
//
// public static String get(Class basicEntityClazz) {
// return entityInfos.get(basicEntityClazz).primaryKey.column;
// }
//
// public static Class getEntity(String name) {
// return (Class) names.get(name);
// }
//
// public static Class getMapper(Class basicEntityClazz) {
// return entityInfos.get(basicEntityClazz).basicMapperClass;
// }
//
// public static BasicExample getExample(Class basicEntityClazz) throws IllegalAccessException, InstantiationException {
// return entityInfos.get(basicEntityClazz).basicExampleClass.newInstance();
// }
//
// public static int getPropertySize(Class basicEntityClazz) {
// return entityInfos.get(basicEntityClazz).fieldPropertyMap.size();
// }
//
// public static String getFieldGetMethodName(String fildeName) {
// byte[] items = fildeName.getBytes();
// items[0] = (byte) ((char) items[0] - 'a' + 'A');
// return new String(items);
// }
//
// private static String getTableByEntity(String entity) {
// StringBuffer stringBuffer = new StringBuffer();
// for (int i = 0; i < entity.length(); i++) {
// char s = entity.charAt(i);
// if (!Character.isLowerCase(s)) {
// stringBuffer.append("_" + s);
// } else {
// stringBuffer.append(s);
// }
// }
// String name = stringBuffer.toString().toLowerCase();
// return name.startsWith("_view") ? "v" + name : "t" + name;
// }
//
// public static void addClassField(Class entity, String column, String property, Class type,String mapperPackage,String entityPackage,boolean isPrimaryKey) throws NoSuchMethodException, ClassNotFoundException {
// TableProperty tableProperty = entityInfos.get(entity);
// if (tableProperty == null) {
// tableProperty = new TableProperty();
// tableProperty.basicMapperClass = (Class) Class.forName( mapperPackage + "." + entity.getSimpleName() + "Mapper");
// tableProperty.basicExampleClass = (Class) Class.forName( entityPackage + "." + entity.getSimpleName() + "Example");
// tableProperty.tableName = getTableByEntity(entity.getSimpleName());
// entityInfos.put(entity, tableProperty);
// names.put(entity.getSimpleName(), entity);
// }
//
// FieldProperty fieldProperty = new FieldProperty();
//
//
// fieldProperty.setMethod = entity.getMethod("set" + getFieldGetMethodName(property), new Class[]{type});
// fieldProperty.getMethod = entity.getMethod("get" + getFieldGetMethodName(property), null);
// fieldProperty.column = column;
// tableProperty.fieldPropertyMap.put(property, fieldProperty);
//
// if (type == String.class) {
// fieldProperty.type = 1;
// } else if (type == Integer.class) {
// fieldProperty.type = 2;
// } else if (type == Date.class) {
// fieldProperty.type = 3;
// } else if (type == Double.class) {
// fieldProperty.type = 4;
// } else if (type == Float.class ){
// fieldProperty.type = 5;
// }
//
// if( isPrimaryKey ){
// tableProperty.primaryKey = fieldProperty;
// }
//
// }
//
// @Deprecated
// public static void mapPropertyToBeanProperty(Map source, Object to) {
//
// Map classFields = entityInfos.get(to.getClass()).fieldPropertyMap;
// Iterator iterator = source.keySet().iterator();
// while (iterator.hasNext()) {
// String name = iterator.next();
// //System.out.println(" " + name);
// FieldProperty classField = classFields.get(name);
// if( classField == null ){
// continue;
// }
// Method method = classField.setMethod;
// Object value = source.get(name);
// try {
// method.invoke(to, value);
// } catch (Exception e) {
// e.printStackTrace();
// }
// }
//
// }
//
// /**
// * 此方法来解决,页面参数直接绑定object,并且通过mybatis保存到数据库。
// * mybatis更新分两种.
// * 1.根据object更新所有字段
// * 2.根据object非null字段更新。
// * 第二种更新机制同页面没办法绑定,因为好多字段有可能更新为null.所以不考虑.
// *
// * 这办法的逻辑是先把页面参数根据object属性过滤并且取出,null也取出。
// * 让后在DAO里通过selectByPrimaryKey,查出对象在付给属性,最后更新到数据库里。
// *
// * @param servletRequest
// * @param clazz
// * @return
// */
// @Deprecated
// public static Map requestParameterToClazzMap(ServletRequest servletRequest, Class clazz) {
//
// Map parmas = new HashMap();
// Map classFields = entityInfos.get(clazz).fieldPropertyMap;
//
// Enumeration enumeration = servletRequest.getParameterNames();
// while (enumeration.hasMoreElements()) {
// String name = enumeration.nextElement();
// try {
// FieldProperty fieldProperty = classFields.get(name);
// if (fieldProperty == null) {
// continue;
// }
// String value = servletRequest.getParameter(name);
// if (fieldProperty.type == 1) {
// parmas.put(name, StringUtils.isEmpty(value) ? null : value);
// } else if (fieldProperty.type == 2) {
// if (NumberUtils.isNumber(value)) {
// parmas.put(name, NumberUtils.toInt(value, 0));
// } else {
// parmas.put(name, null);
// }
// } else if (fieldProperty.type == 3) {
// parmas.put(name, getDate(value));
// } else if (fieldProperty.type == 4) {
// if (NumberUtils.isNumber(value)) {
// parmas.put(name, NumberUtils.toDouble(value, 0));
// } else {
// parmas.put(name, null);
// }
// } else if (fieldProperty.type == 5 ){
// if (NumberUtils.isNumber(value)) {
// parmas.put(name, NumberUtils.toFloat(value, 0));
// } else {
// parmas.put(name, null);
// }
// }
// } catch (Exception e) {
// logger.debug(clazz.getCanonicalName() + " " + name, e);
// }
// }
// return parmas;
// }
//
// public static Date getDate(String value) {
//
// if (StringUtils.isBlank(value)) {
// return null;
// }
//
// SimpleDateFormat simpleDateFormat = null;
// if (value.matches(regex1)) {
// simpleDateFormat = simpleDateFormat1;
// } else if (value.matches(regex2)) {
// simpleDateFormat = simpleDateFormat2;
// } else {
// return null;
// }
//
// try {
// return simpleDateFormat.parse(value.trim());
// } catch (ParseException e) {
// return null;
// }
//
// }
//
//
//}
//
© 2015 - 2025 Weber Informatics LLC | Privacy Policy