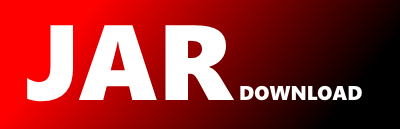
com.github.jaiimageio.plugins.bmp.BMPImageWriteParam Maven / Gradle / Ivy
Show all versions of jai-imageio-core Show documentation
/*
* $RCSfile: BMPImageWriteParam.java,v $
*
*
* Copyright (c) 2005 Sun Microsystems, Inc. All Rights Reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
*
* - Redistribution of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
*
* - Redistribution in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in
* the documentation and/or other materials provided with the
* distribution.
*
* Neither the name of Sun Microsystems, Inc. or the names of
* contributors may be used to endorse or promote products derived
* from this software without specific prior written permission.
*
* This software is provided "AS IS," without a warranty of any
* kind. ALL EXPRESS OR IMPLIED CONDITIONS, REPRESENTATIONS AND
* WARRANTIES, INCLUDING ANY IMPLIED WARRANTY OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE OR NON-INFRINGEMENT, ARE HEREBY
* EXCLUDED. SUN MIDROSYSTEMS, INC. ("SUN") AND ITS LICENSORS SHALL
* NOT BE LIABLE FOR ANY DAMAGES SUFFERED BY LICENSEE AS A RESULT OF
* USING, MODIFYING OR DISTRIBUTING THIS SOFTWARE OR ITS
* DERIVATIVES. IN NO EVENT WILL SUN OR ITS LICENSORS BE LIABLE FOR
* ANY LOST REVENUE, PROFIT OR DATA, OR FOR DIRECT, INDIRECT, SPECIAL,
* CONSEQUENTIAL, INCIDENTAL OR PUNITIVE DAMAGES, HOWEVER CAUSED AND
* REGARDLESS OF THE THEORY OF LIABILITY, ARISING OUT OF THE USE OF OR
* INABILITY TO USE THIS SOFTWARE, EVEN IF SUN HAS BEEN ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGES.
*
* You acknowledge that this software is not designed or intended for
* use in the design, construction, operation or maintenance of any
* nuclear facility.
*
* $Revision: 1.2 $
* $Date: 2006/04/14 21:32:04 $
* $State: Exp $
*/
package com.github.jaiimageio.plugins.bmp;
import java.util.Locale;
import javax.imageio.ImageWriteParam;
import com.github.jaiimageio.impl.plugins.bmp.BMPConstants;
/**
* A subclass of ImageWriteParam
for encoding images in
* the BMP format.
*
* This class allows for the specification of various parameters
* while writing a BMP format image file. By default, the data layout
* is bottom-up, such that the pixels are stored in bottom-up order,
* the first scanline being stored last.
*
*
The particular compression scheme to be used can be specified by using
* the setCompressionType()
method with the appropriate type
* string. The compression scheme specified will be honored if and only if it
* is compatible with the type of image being written. If the specified
* compression scheme is not compatible with the type of image being written
* then the IOException
will be thrown by the BMP image writer.
* If the compression type is not set explicitly then getCompressionType()
* will return null
. In this case the BMP image writer will select
* a compression type that supports encoding of the given image without loss
* of the color resolution.
*
The compression type strings and the image type(s) each supports are
* listed in the following
* table:
*
*
* Compression Types
* Type String Description Image Types
* BI_RGB Uncompressed RLE <= 8-bits/sample
* BI_RLE8 8-bit Run Length Encoding <= 8-bits/sample
* BI_RLE4 4-bit Run Length Encoding <= 4-bits/sample
* BI_BITFIELDS Packed data 16 or 32 bits/sample
* BI_JPEG JPEG encoded grayscale or RGB image
*
*
* When BI_BITFIELDS
is used, if the image encoded has a
* DirectColorModel
, the bit mask in the color model will be
* written into the stream. Otherwise, only 5-5-5 16-bit image or 8-8-8
* 32-bit images are supported.
*
*/
public class BMPImageWriteParam extends ImageWriteParam {
// deprecated version constants
/**
* Constant for BMP version 2.
*
* @deprecated
*/
public static final int VERSION_2 = 0;
/**
* Constant for BMP version 3.
*
* @deprecated
*/
public static final int VERSION_3 = 1;
/**
* Constant for BMP version 4.
*
* @deprecated
*/
public static final int VERSION_4 = 2;
/**
* Constant for BMP version 5.
*
* @deprecated
*/
public static final int VERSION_5 = 3;
private boolean topDown = false;
/**
* Constructs a BMPImageWriteParam
set to use a given
* Locale
and with default values for all parameters.
*
* @param locale a Locale
to be used to localize
* compression type names and quality descriptions, or
* null
.
*/
public BMPImageWriteParam(Locale locale) {
super(locale);
// Set compression types ("BI_RGB" denotes uncompressed).
compressionTypes = BMPConstants.compressionTypeNames;
// Set compression flag.
canWriteCompressed = true;
compressionMode = MODE_COPY_FROM_METADATA;
compressionType = compressionTypes[BMPConstants.BI_RGB];
}
/**
* Constructs an BMPImageWriteParam
object with default
* values for all parameters and a null
Locale
.
*/
public BMPImageWriteParam() {
this(null);
}
/**
* Returns the BMP version to be used. The default is
* VERSION_3
.
*
* @deprecated
* @return the BMP version number.
*/
public int getVersion() {
return VERSION_3;
}
/**
* If set, the data will be written out in a top-down manner, the first
* scanline being written first.
*
* Any compression other than BI_RGB
or
* BI_BITFIELDS
is incompatible with the data being
* written in top-down order. Setting the topDown
argument
* to true
will be honored only when the compression
* type at the time of writing the image is one of the two mentioned
* above. Otherwise, the topDown
setting will be ignored.
*
* @param topDown whether the data are written in top-down order.
*/
public void setTopDown(boolean topDown) {
this.topDown = topDown;
}
/**
* Returns the value of the topDown
parameter.
* The default is false
.
*
* @return whether the data are written in top-down order.
*/
public boolean isTopDown() {
return topDown;
}
// Override superclass implementation to add a new check that compression
// is not being set when image has been specified to be encoded in a top
// down fashion
/**
* Sets the compression type to one of the values indicated by
* getCompressionTypes
. If a value of
* null
is passed in, any previous setting is
* removed.
*
*
The method first invokes
* {@link javax.imageio.ImageWriteParam.#setCompressionType(String)
* setCompressionType()
}
* with the supplied value of compressionType
. Next,
* if {@link #isTopDown()} returns true
and the
* value of compressionType
is incompatible with top-down
* order, {@link #setTopDown(boolean)} is invoked with parameter
* topDown
set to false
. The image will
* then be written in bottom-up order with the specified
* compressionType
.
*
* @param compressionType one of the String
s returned
* by getCompressionTypes
, or null
to
* remove any previous setting.
*
* @exception UnsupportedOperationException if the writer does not
* support compression.
* @exception IllegalStateException if the compression mode is not
* MODE_EXPLICIT
.
* @exception UnsupportedOperationException if there are no
* settable compression types.
* @exception IllegalArgumentException if
* compressionType
is non-null
but is not
* one of the values returned by getCompressionTypes
.
*
* @see #isTopDown
* @see #setTopDown
* @see #getCompressionTypes
* @see #getCompressionType
* @see #unsetCompression
*/
public void setCompressionType(String compressionType) {
super.setCompressionType(compressionType);
if (!(compressionType.equals("BI_RGB")) &&
!(compressionType.equals("BI_BITFIELDS")) && topDown == true) {
topDown = false;
}
}
}