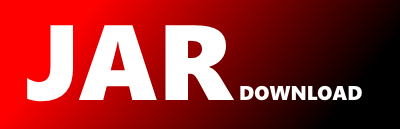
com.github.jaiimageio.plugins.tiff.TIFFImageReadParam Maven / Gradle / Ivy
Show all versions of jai-imageio-core Show documentation
/*
* $RCSfile: TIFFImageReadParam.java,v $
*
*
* Copyright (c) 2005 Sun Microsystems, Inc. All Rights Reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
*
* - Redistribution of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
*
* - Redistribution in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in
* the documentation and/or other materials provided with the
* distribution.
*
* Neither the name of Sun Microsystems, Inc. or the names of
* contributors may be used to endorse or promote products derived
* from this software without specific prior written permission.
*
* This software is provided "AS IS," without a warranty of any
* kind. ALL EXPRESS OR IMPLIED CONDITIONS, REPRESENTATIONS AND
* WARRANTIES, INCLUDING ANY IMPLIED WARRANTY OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE OR NON-INFRINGEMENT, ARE HEREBY
* EXCLUDED. SUN MIDROSYSTEMS, INC. ("SUN") AND ITS LICENSORS SHALL
* NOT BE LIABLE FOR ANY DAMAGES SUFFERED BY LICENSEE AS A RESULT OF
* USING, MODIFYING OR DISTRIBUTING THIS SOFTWARE OR ITS
* DERIVATIVES. IN NO EVENT WILL SUN OR ITS LICENSORS BE LIABLE FOR
* ANY LOST REVENUE, PROFIT OR DATA, OR FOR DIRECT, INDIRECT, SPECIAL,
* CONSEQUENTIAL, INCIDENTAL OR PUNITIVE DAMAGES, HOWEVER CAUSED AND
* REGARDLESS OF THE THEORY OF LIABILITY, ARISING OUT OF THE USE OF OR
* INABILITY TO USE THIS SOFTWARE, EVEN IF SUN HAS BEEN ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGES.
*
* You acknowledge that this software is not designed or intended for
* use in the design, construction, operation or maintenance of any
* nuclear facility.
*
* $Revision: 1.1 $
* $Date: 2005/02/11 05:01:18 $
* $State: Exp $
*/
package com.github.jaiimageio.plugins.tiff;
import java.util.ArrayList;
import java.util.List;
import javax.imageio.ImageReadParam;
/**
* A subclass of {@link ImageReadParam} allowing control over
* the TIFF reading process.
*
* Because TIFF is an extensible format, the reader requires
* information about any tags used by TIFF extensions in order to emit
* meaningful metadata. Also, TIFF extensions may define new
* compression types. Both types of information about extensions may
* be provided by this interface.
*
*
Additional TIFF tags must be organized into
* TIFFTagSet
s. A TIFFTagSet
may be
* provided to the reader by means of the
* addAllowedTagSet
method. By default, the tag sets
* BaselineTIFFTagSet
, FaxTIFFTagSet
,
* EXIFParentTIFFTagSet
, and GeoTIFFTagSet
* are included.
*
*
New TIFF decompressors are handled in a simple fashion. If a
* non-null
TIFFDecompressor
is provided by
* means of the setTIFFDecompressor method, it will override the
* reader's usual choice of decompressor. Thus, to read an image with
* a non-standard compression type, the application should first
* attempt to read the image's metadata and extract the compression
* type. The application may then use its own logic to choose a
* suitable TIFFDecompressor
, instantiate it, and pass it
* to the ImageReadParam
being used. The reader's
* read
method may be called with the
* ImageReadParam
set.
*/
public class TIFFImageReadParam extends ImageReadParam {
List allowedTagSets = new ArrayList(4);
TIFFDecompressor decompressor = null;
TIFFColorConverter colorConverter = null;
/**
* Constructs a TIFFImageReadParam
. Tags defined by
* the TIFFTagSet
s BaselineTIFFTagSet
,
* FaxTIFFTagSet
, EXIFParentTIFFTagSet
, and
* GeoTIFFTagSet
will be supported.
*
* @see BaselineTIFFTagSet
* @see FaxTIFFTagSet
* @see EXIFParentTIFFTagSet
* @see GeoTIFFTagSet
*/
public TIFFImageReadParam() {
addAllowedTagSet(BaselineTIFFTagSet.getInstance());
addAllowedTagSet(FaxTIFFTagSet.getInstance());
addAllowedTagSet(EXIFParentTIFFTagSet.getInstance());
addAllowedTagSet(GeoTIFFTagSet.getInstance());
}
/**
* Adds a TIFFTagSet
object to the list of allowed
* tag sets.
*
* @param tagSet a TIFFTagSet
.
*
* @throws IllegalArgumentException if tagSet
is
* null
.
*/
public void addAllowedTagSet(TIFFTagSet tagSet) {
if (tagSet == null) {
throw new IllegalArgumentException("tagSet == null!");
}
allowedTagSets.add(tagSet);
}
/**
* Removes a TIFFTagSet
object from the list of
* allowed tag sets. Removal is based on the equals
* method of the TIFFTagSet
, which is normally
* defined as reference equality.
*
* @param tagSet a TIFFTagSet
.
*
* @throws IllegalArgumentException if tagSet
is
* null
.
*/
public void removeAllowedTagSet(TIFFTagSet tagSet) {
if (tagSet == null) {
throw new IllegalArgumentException("tagSet == null!");
}
allowedTagSets.remove(tagSet);
}
/**
* Returns a List
containing the allowed
* TIFFTagSet
objects.
*
* @return a List
of TIFFTagSet
s.
*/
public List getAllowedTagSets() {
return allowedTagSets;
}
/**
* Sets the TIFFDecompressor
object to be used by the
* ImageReader
to decode each image strip or tile.
* A value of null
allows the reader to choose its
* own TIFFDecompressor.
*
* @param decompressor the TIFFDecompressor
to be
* used for decoding, or null
to allow the reader to
* choose its own.
*
* @see #getTIFFDecompressor
*/
public void setTIFFDecompressor(TIFFDecompressor decompressor) {
this.decompressor = decompressor;
}
/**
* Returns the TIFFDecompressor
that is currently set
* to be used by the ImageReader
to decode each image
* strip or tile, or null
if none has been set.
*
* @return decompressor the TIFFDecompressor
to be
* used for decoding, or null
if none has been set
* (allowing the reader to choose its own).
*
* @see #setTIFFDecompressor(TIFFDecompressor)
*/
public TIFFDecompressor getTIFFDecompressor() {
return this.decompressor;
}
/**
* Sets the TIFFColorConverter
object for the pixel data
* being read. The data will be converted from the given color
* space to a standard RGB space as it is being read. A value of
* null
disables conversion.
*
* @param colorConverter a TIFFColorConverter
object
* to be used for final color conversion, or null
.
*
* @see #getColorConverter
*/
public void setColorConverter(TIFFColorConverter colorConverter) {
this.colorConverter = colorConverter;
}
/**
* Returns the currently set TIFFColorConverter
object,
* or null
if none is set.
*
* @return the current TIFFColorConverter
object.
*
* @see #setColorConverter(TIFFColorConverter)
*/
public TIFFColorConverter getColorConverter() {
return this.colorConverter;
}
}