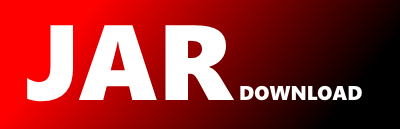
scalacss.internal.CanIUse.scala Maven / Gradle / Ivy
The newest version!
package scalacss.internal
////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
//
// NOTICE: This file is generated by misc/caniuse.scala
//
////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
import japgolly.univeq.UnivEq
object CanIUse {
type VerStr = String
type Subject = Map[Agent, Set[Support]]
sealed trait Support
object Support {
case object Unsupported extends Support
case object Unknown extends Support
case object Partial extends Support
case object Full extends Support
case object PartialX extends Support
case object FullX extends Support
implicit def univEq: UnivEq[Support] = UnivEq.derive
}
sealed abstract class Prefix(val name: String) {
val prefix = "-" + name + "-"
}
object Prefix {
case object moz extends Prefix("moz")
case object ms extends Prefix("ms")
case object o extends Prefix("o")
case object webkit extends Prefix("webkit")
implicit def univEq: UnivEq[Prefix] = UnivEq.derive
implicit val ordering: Ordering[Prefix] = Ordering.by(_.name)
val values = NonEmptyVector[Prefix](moz, ms, o, webkit)
}
import Prefix._
final case class Agent(prefix: Prefix, prefixExceptions: Map[VerStr, Prefix])
object Agent {
val AndroidBrowser = Agent(webkit, Map.empty)
val AndroidChrome = Agent(webkit, Map.empty)
val AndroidFirefox = Agent(moz , Map.empty)
val AndroidUC = Agent(webkit, Map("11.4" -> webkit))
val BaiduBrowser = Agent(webkit, Map.empty)
val BlackberryBrowser = Agent(webkit, Map.empty)
val Chrome = Agent(webkit, Map.empty)
val Edge = Agent(ms , Map.empty)
val Firefox = Agent(moz , Map.empty)
val IE = Agent(ms , Map.empty)
val IEMobile = Agent(ms , Map.empty)
val IOSSafari = Agent(webkit, Map.empty)
val Opera = Agent(webkit, Map("9,9.5-9.6,10.0-10.1,10.5,10.6,11,11.1,11.5,11.6,12,12.1" -> o))
val OperaMini = Agent(o , Map.empty)
val OperaMobile = Agent(o , Map("37" -> webkit))
val QQBrowser = Agent(webkit, Map.empty)
val Safari = Agent(webkit, Map.empty)
val Samsung = Agent(webkit, Map.empty)
implicit def univEq: UnivEq[Agent] = UnivEq.derive
val values = NonEmptyVector[Agent](AndroidBrowser, AndroidChrome, AndroidFirefox, AndroidUC, BaiduBrowser, BlackberryBrowser, Chrome, Edge, Firefox, IE, IEMobile, IOSSafari, Opera, OperaMini, OperaMobile, QQBrowser, Safari, Samsung)
}
import Agent._
import Support._
/**
* CSS Animation
*
* Complex method of animating certain properties of an element
*
* https://www.w3.org/TR/css3-animations/
*/
val animation: Subject = Map(
AndroidBrowser -> Set(PartialX, FullX, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(FullX),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(FullX),
Chrome -> Set(FullX, Full),
Edge -> Set(Full),
Firefox -> Set(Unsupported, FullX, Full),
IE -> Set(Unsupported, Full),
IEMobile -> Set(Full),
IOSSafari -> Set(PartialX, FullX, Full),
Opera -> Set(Unsupported, FullX, Full),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported, Full),
QQBrowser -> Set(FullX),
Safari -> Set(Unsupported, PartialX, FullX, Full),
Samsung -> Set(Full))
/**
* CSS Appearance
*
* The `appearance` property defines how elements (particularly form controls) appear by default. By setting the value to `none` the default appearance can be entirely redefined using other CSS properties.
*
* https://drafts.csswg.org/css-ui-4/#appearance-switching
*/
def appearance: Subject = Map(
AndroidBrowser -> Set(PartialX),
AndroidChrome -> Set(PartialX),
AndroidFirefox -> Set(PartialX),
AndroidUC -> Set(PartialX),
BaiduBrowser -> Set(PartialX),
BlackberryBrowser -> Set(PartialX),
Chrome -> Set(PartialX),
Edge -> Set(Partial),
Firefox -> Set(PartialX),
IE -> Set(Unsupported),
IEMobile -> Set(Unsupported, Partial),
IOSSafari -> Set(PartialX),
Opera -> Set(Unsupported, PartialX),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported, PartialX),
QQBrowser -> Set(PartialX),
Safari -> Set(PartialX),
Samsung -> Set(PartialX))
/**
* CSS background-attachment
*
* Method of defining how a background image is attached to a scrollable element. Values include `scroll` (default), `fixed` and `local`.
*
* https://www.w3.org/TR/css3-background/#the-background-attachment
*/
def backgroundAttachment: Subject = Map(
AndroidBrowser -> Set(Unsupported, Partial),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Partial),
BaiduBrowser -> Set(Partial),
BlackberryBrowser -> Set(Partial),
Chrome -> Set(Full),
Edge -> Set(Full),
Firefox -> Set(Partial, Full),
IE -> Set(Partial, Full),
IEMobile -> Set(Full),
IOSSafari -> Set(Unsupported, Partial),
Opera -> Set(Partial, Full),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Partial, Full),
QQBrowser -> Set(Full),
Safari -> Set(Partial, Full),
Samsung -> Set(Unsupported, Partial))
/**
* CSS3 Background-image options
*
* New properties to affect background images, including background-clip, background-origin and background-size
*
* https://www.w3.org/TR/css3-background/#backgrounds
*/
def backgroundImgOpts: Subject = Map(
AndroidBrowser -> Set(PartialX, Partial, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Full),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(Full),
Chrome -> Set(Partial, Full),
Edge -> Set(Full),
Firefox -> Set(Unsupported, PartialX, Full),
IE -> Set(Unsupported, Full),
IEMobile -> Set(Full),
IOSSafari -> Set(Partial, Full),
Opera -> Set(Unsupported, PartialX, Full),
OperaMini -> Set(Partial),
OperaMobile -> Set(Full),
QQBrowser -> Set(Full),
Safari -> Set(Partial, Full),
Samsung -> Set(Full))
/**
* CSS background-position edge offsets
*
* Allows CSS background images to be positioned relative to the specified edge using the 3 to 4 value syntax. For example: `background-position: right 5px bottom 5px;` for positioning 5px from the bottom-right corner.
*
* https://www.w3.org/TR/css3-background/#background-position
*/
def backgroundOffsets: Subject = Map(
AndroidBrowser -> Set(Unsupported, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Full),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(Unsupported, Full),
Chrome -> Set(Unsupported, Full),
Edge -> Set(Full),
Firefox -> Set(Unsupported, Full),
IE -> Set(Unsupported, Full),
IEMobile -> Set(Full),
IOSSafari -> Set(Unsupported, Full),
Opera -> Set(Unsupported, Full),
OperaMini -> Set(Full),
OperaMobile -> Set(Unsupported, Full),
QQBrowser -> Set(Full),
Safari -> Set(Unsupported, Full),
Samsung -> Set(Full))
/**
* CSS background-repeat round and space
*
* Allows CSS background images to be repeated without clipping.
*
* https://www.w3.org/TR/css3-background/#the-background-repeat
*/
def backgroundRepeatRoundSpace: Subject = Map(
AndroidBrowser -> Set(Unsupported, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Full),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(Unsupported, Full),
Chrome -> Set(Unsupported, Full),
Edge -> Set(Full),
Firefox -> Set(Unsupported, Full),
IE -> Set(Unsupported, Partial, Full),
IEMobile -> Set(Full),
IOSSafari -> Set(Unsupported, Full),
Opera -> Set(Unsupported, Full),
OperaMini -> Set(Full),
OperaMobile -> Set(Unsupported, Full),
QQBrowser -> Set(Full),
Safari -> Set(Unsupported, Full),
Samsung -> Set(Full))
/**
* CSS background-blend-mode
*
* Allows blending between CSS background images, gradients, and colors.
*
* https://www.w3.org/TR/compositing-1/#propdef-background-blend-mode
*/
def backgroundblendmode: Subject = Map(
AndroidBrowser -> Set(Unsupported, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Full),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(Unsupported),
Chrome -> Set(Unsupported, Full, Partial),
Edge -> Set(Unsupported),
Firefox -> Set(Unsupported, Full),
IE -> Set(Unsupported),
IEMobile -> Set(Unsupported),
IOSSafari -> Set(Unsupported, Partial),
Opera -> Set(Unsupported, Full, Partial),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported, Partial),
QQBrowser -> Set(Full),
Safari -> Set(Unsupported, Partial),
Samsung -> Set(Full))
/**
* CSS3 Border images
*
* Method of using images for borders
*
* https://www.w3.org/TR/css3-background/#border-images
*/
val borderImage: Subject = Map(
AndroidBrowser -> Set(PartialX, Partial),
AndroidChrome -> Set(Partial),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Partial),
BaiduBrowser -> Set(Partial),
BlackberryBrowser -> Set(Partial),
Chrome -> Set(PartialX, Partial),
Edge -> Set(Full),
Firefox -> Set(Unsupported, PartialX, Partial, Full),
IE -> Set(Unsupported, Full),
IEMobile -> Set(Unsupported, Full),
IOSSafari -> Set(PartialX, Partial, Full),
Opera -> Set(Unsupported, Partial, PartialX),
OperaMini -> Set(PartialX),
OperaMobile -> Set(Unsupported, PartialX, Partial),
QQBrowser -> Set(Partial),
Safari -> Set(PartialX, Partial, Full),
Samsung -> Set(Partial))
/**
* CSS3 Border-radius (rounded corners)
*
* Method of making the border corners round. Covers support for the shorthand `border-radius` as well as the long-hand properties (e.g. `border-top-left-radius`)
*
* https://www.w3.org/TR/css3-background/#the-border-radius
*/
val borderRadius: Subject = Map(
AndroidBrowser -> Set(FullX, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Full),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(Full),
Chrome -> Set(FullX, Full),
Edge -> Set(Full),
Firefox -> Set(PartialX, FullX, Full),
IE -> Set(Unsupported, Full),
IEMobile -> Set(Full),
IOSSafari -> Set(FullX, Full),
Opera -> Set(Unsupported, Full),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported, Full),
QQBrowser -> Set(Full),
Safari -> Set(FullX, Full),
Samsung -> Set(Full))
/**
* CSS box-decoration-break
*
* Controls whether the box's margins, borders, padding, and other decorations wrap the broken edges of the box fragments (when the box is split by a break (page/column/region/line).
*
* https://www.w3.org/TR/css3-break/#break-decoration
*/
def boxdecorationbreak: Subject = Map(
AndroidBrowser -> Set(Unsupported, PartialX),
AndroidChrome -> Set(PartialX),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Full),
BaiduBrowser -> Set(PartialX),
BlackberryBrowser -> Set(Unsupported, PartialX),
Chrome -> Set(Unsupported, PartialX),
Edge -> Set(Unsupported),
Firefox -> Set(Unsupported, Full),
IE -> Set(Unsupported),
IEMobile -> Set(Unsupported),
IOSSafari -> Set(Unsupported, PartialX),
Opera -> Set(Unsupported, Full, PartialX),
OperaMini -> Set(Partial),
OperaMobile -> Set(Unsupported, Full, PartialX),
QQBrowser -> Set(PartialX),
Safari -> Set(Unsupported, PartialX),
Samsung -> Set(PartialX))
/**
* CSS3 Box-shadow
*
* Method of displaying an inner or outer shadow effect to elements
*
* https://www.w3.org/TR/css3-background/#box-shadow
*/
def boxshadow: Subject = Map(
AndroidBrowser -> Set(PartialX, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Full),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(FullX, Full),
Chrome -> Set(FullX, Full),
Edge -> Set(Full),
Firefox -> Set(Unsupported, FullX, Full),
IE -> Set(Unsupported, Full),
IEMobile -> Set(Full),
IOSSafari -> Set(PartialX, FullX, Full),
Opera -> Set(Unsupported, Full),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported, Full),
QQBrowser -> Set(Full),
Safari -> Set(PartialX, FullX, Full),
Samsung -> Set(Full))
/**
* calc() as CSS unit value
*
* Method of allowing calculated values for length units, i.e. `width: calc(100% - 3em)`
*
* https://www.w3.org/TR/css3-values/#calc
*/
def calc: Subject = Map(
AndroidBrowser -> Set(Unsupported, Partial, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Full),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(Unsupported, Full),
Chrome -> Set(Unsupported, FullX, Full),
Edge -> Set(Full),
Firefox -> Set(Unsupported, FullX, Full),
IE -> Set(Unsupported, Partial, Full),
IEMobile -> Set(Full),
IOSSafari -> Set(Unsupported, FullX, Full),
Opera -> Set(Unsupported, Full),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported, Full),
QQBrowser -> Set(Full),
Safari -> Set(Unsupported, FullX, Full),
Samsung -> Set(Full))
/**
* CSS Canvas Drawings
*
* Method of using HTML5 Canvas as a background image. Not currently part of any specification.
*
* http://webkit.org/blog/176/css-canvas-drawing/
*/
def canvas: Subject = Map(
AndroidBrowser -> Set(FullX, Unsupported),
AndroidChrome -> Set(Unsupported),
AndroidFirefox -> Set(Unsupported),
AndroidUC -> Set(FullX),
BaiduBrowser -> Set(Unsupported),
BlackberryBrowser -> Set(FullX),
Chrome -> Set(FullX, Unsupported),
Edge -> Set(Unsupported),
Firefox -> Set(Unsupported, Unknown),
IE -> Set(Unsupported),
IEMobile -> Set(Unsupported),
IOSSafari -> Set(FullX),
Opera -> Set(Unsupported, FullX),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported),
QQBrowser -> Set(FullX),
Safari -> Set(Unsupported, FullX),
Samsung -> Set(FullX, Unsupported))
/**
* CSS caret-color
*
* The `caret-color` property allows the color to be set of the caret (blinking text insertion pointer) in an editable text area.
*
* https://www.w3.org/TR/css-ui-3/#caret-color
*/
def caretColor: Subject = Map(
AndroidBrowser -> Set(Unsupported, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Unsupported),
BaiduBrowser -> Set(Unsupported),
BlackberryBrowser -> Set(Unsupported),
Chrome -> Set(Unsupported, Full),
Edge -> Set(Unsupported),
Firefox -> Set(Unsupported, Full),
IE -> Set(Unsupported),
IEMobile -> Set(Unsupported),
IOSSafari -> Set(Unsupported),
Opera -> Set(Unsupported, Full),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported),
QQBrowser -> Set(Unsupported),
Safari -> Set(Unsupported, Full),
Samsung -> Set(Unsupported))
/**
* ch (character) unit
*
* Unit representing the width of the character "0" in the current font, of particular use in combination with monospace fonts.
*
* https://www.w3.org/TR/css3-values/#ch
*/
def chUnit: Subject = Map(
AndroidBrowser -> Set(Unsupported, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Full),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(Unsupported, Full),
Chrome -> Set(Unsupported, Full),
Edge -> Set(Full),
Firefox -> Set(Full),
IE -> Set(Unsupported, Partial),
IEMobile -> Set(Full),
IOSSafari -> Set(Unsupported, Full),
Opera -> Set(Unsupported, Full),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported, Full),
QQBrowser -> Set(Full),
Safari -> Set(Unsupported, Full),
Samsung -> Set(Full))
/**
* CSS clip-path property (for HTML)
*
* Method of defining the visible region of an HTML element using SVG or a shape definition.
*
* https://www.w3.org/TR/css-masking-1/#the-clip-path
*/
def clipPath: Subject = Map(
AndroidBrowser -> Set(Unsupported, PartialX, Partial),
AndroidChrome -> Set(Partial),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(PartialX),
BaiduBrowser -> Set(Partial),
BlackberryBrowser -> Set(Unsupported),
Chrome -> Set(Unsupported, PartialX, Partial),
Edge -> Set(Unsupported),
Firefox -> Set(Unsupported, Partial, Full),
IE -> Set(Unsupported),
IEMobile -> Set(Unsupported),
IOSSafari -> Set(Unsupported, PartialX),
Opera -> Set(Unsupported, PartialX, Partial),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported, PartialX),
QQBrowser -> Set(PartialX),
Safari -> Set(Unsupported, PartialX),
Samsung -> Set(PartialX))
/**
* CSS Counters
*
* Method of controlling number values in generated content, using the `counter-reset` and `counter-increment` properties.
*
* https://www.w3.org/TR/CSS21/generate.html#counters
*/
val counters: Subject = Map(
AndroidBrowser -> Set(Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Full),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(Full),
Chrome -> Set(Full),
Edge -> Set(Full),
Firefox -> Set(Full),
IE -> Set(Unsupported, Full),
IEMobile -> Set(Full),
IOSSafari -> Set(Full),
Opera -> Set(Full),
OperaMini -> Set(Full),
OperaMobile -> Set(Full),
QQBrowser -> Set(Full),
Safari -> Set(Full),
Samsung -> Set(Full))
/**
* Crisp edges/pixelated images
*
* Scales images with an algorithm that preserves edges and contrast, without smoothing colors or introducing blur. This is intended for images such as pixel art. Official values that accomplish this for the `image-rendering` property are `crisp-edges` and `pixelated`.
*
* https://drafts.csswg.org/css-images-3/#valdef-image-rendering-crisp-edges
*/
def crispEdges: Subject = Map(
AndroidBrowser -> Set(Unsupported, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(FullX),
AndroidUC -> Set(PartialX),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(Unsupported, PartialX),
Chrome -> Set(Unsupported, Full),
Edge -> Set(Unsupported),
Firefox -> Set(Unsupported, FullX),
IE -> Set(Unsupported, PartialX),
IEMobile -> Set(PartialX),
IOSSafari -> Set(Unsupported, PartialX, Full),
Opera -> Set(Unsupported, FullX, Full),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported, FullX, Full),
QQBrowser -> Set(Unsupported),
Safari -> Set(Unsupported, PartialX, Full),
Samsung -> Set(Full))
/**
* CSS3 Box-sizing
*
* Method of specifying whether or not an element's borders and padding should be included in size units
*
* https://www.w3.org/TR/css3-ui/#box-sizing
*/
def css3Boxsizing: Subject = Map(
AndroidBrowser -> Set(FullX, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Full),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(FullX, Full),
Chrome -> Set(FullX, Full),
Edge -> Set(Full),
Firefox -> Set(FullX, Full),
IE -> Set(Unsupported, Full),
IEMobile -> Set(Full),
IOSSafari -> Set(FullX, Full),
Opera -> Set(Unsupported, Full),
OperaMini -> Set(Full),
OperaMobile -> Set(Full),
QQBrowser -> Set(Full),
Safari -> Set(FullX, Full),
Samsung -> Set(Full))
/**
* CSS3 Cursors: zoom-in & zoom-out
*
* Support for `zoom-in`, `zoom-out` values for the CSS3 `cursor` property.
*
* https://www.w3.org/TR/css3-ui/#cursor
*/
def css3CursorsNewer: Subject = Map(
AndroidBrowser -> Set(Unsupported),
AndroidChrome -> Set(Unsupported),
AndroidFirefox -> Set(Unsupported),
AndroidUC -> Set(Unsupported),
BaiduBrowser -> Set(Unsupported),
BlackberryBrowser -> Set(FullX),
Chrome -> Set(FullX, Full),
Edge -> Set(Full),
Firefox -> Set(FullX, Full),
IE -> Set(Unsupported),
IEMobile -> Set(Unsupported),
IOSSafari -> Set(Unsupported),
Opera -> Set(Unsupported, Full, FullX),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported),
QQBrowser -> Set(Unsupported),
Safari -> Set(FullX, Full),
Samsung -> Set(Unsupported))
/**
* CSS3 tab-size
*
* Method of customizing the width of the tab character. Only effective using 'white-space: pre' or 'white-space: pre-wrap'.
*
* https://www.w3.org/TR/css3-text/#tab-size
*/
def css3Tabsize: Subject = Map(
AndroidBrowser -> Set(Unsupported, Partial, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(FullX),
AndroidUC -> Set(Full),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(Partial),
Chrome -> Set(Unsupported, Partial, Full),
Edge -> Set(Unsupported),
Firefox -> Set(Unsupported, PartialX, FullX),
IE -> Set(Unsupported),
IEMobile -> Set(Unsupported),
IOSSafari -> Set(Unsupported, Partial),
Opera -> Set(Unsupported, PartialX, Partial, Full),
OperaMini -> Set(PartialX),
OperaMobile -> Set(Unsupported, PartialX, Full),
QQBrowser -> Set(Full),
Safari -> Set(Unsupported, Partial),
Samsung -> Set(Full))
/**
* CSS Device Adaptation
*
* A standard way to override the size of viewport in web page using the `@viewport` rule, standardizing and replacing Apple's own popular `<meta>` viewport implementation.
*
* https://www.w3.org/TR/css-device-adapt/
*/
def deviceadaptation: Subject = Map(
AndroidBrowser -> Set(Unsupported),
AndroidChrome -> Set(Unsupported),
AndroidFirefox -> Set(Unsupported),
AndroidUC -> Set(Unsupported),
BaiduBrowser -> Set(Unsupported),
BlackberryBrowser -> Set(Unsupported),
Chrome -> Set(Unsupported),
Edge -> Set(PartialX),
Firefox -> Set(Unsupported),
IE -> Set(Unsupported, PartialX),
IEMobile -> Set(PartialX),
IOSSafari -> Set(Unsupported),
Opera -> Set(Unsupported),
OperaMini -> Set(PartialX),
OperaMobile -> Set(Unsupported, PartialX),
QQBrowser -> Set(Unsupported),
Safari -> Set(Unsupported),
Samsung -> Set(Unsupported))
/**
* CSS display: contents
*
* `display: contents` causes an element's children to appear as if they were direct children of the element's parent, ignoring the element itself. This can be useful when a wrapper element should be ignored when using CSS grid or similar layout techniques.
*
* https://drafts.csswg.org/css-display/
*/
def displayContents: Subject = Map(
AndroidBrowser -> Set(Unsupported),
AndroidChrome -> Set(Unsupported),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Unsupported),
BaiduBrowser -> Set(Unsupported),
BlackberryBrowser -> Set(Unsupported),
Chrome -> Set(Unsupported),
Edge -> Set(Unsupported),
Firefox -> Set(Unsupported, Full),
IE -> Set(Unsupported),
IEMobile -> Set(Unsupported),
IOSSafari -> Set(Unsupported),
Opera -> Set(Unsupported),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported),
QQBrowser -> Set(Unsupported),
Safari -> Set(Unsupported, Full),
Samsung -> Set(Unsupported))
/**
* CSS Feature Queries
*
* CSS Feature Queries allow authors to condition rules based on whether particular property declarations are supported in CSS using the @supports at rule.
*
* https://www.w3.org/TR/css3-conditional/#at-supports
*/
def featurequeries: Subject = Map(
AndroidBrowser -> Set(Unsupported, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Full),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(Unsupported),
Chrome -> Set(Unsupported, Full),
Edge -> Set(Full),
Firefox -> Set(Unsupported, Full),
IE -> Set(Unsupported),
IEMobile -> Set(Unsupported),
IOSSafari -> Set(Unsupported, Full),
Opera -> Set(Unsupported, Full),
OperaMini -> Set(Full),
OperaMobile -> Set(Unsupported, Full),
QQBrowser -> Set(Full),
Safari -> Set(Unsupported, Full),
Samsung -> Set(Full))
/**
* CSS Filter Effects
*
* Method of applying filter effects (like blur, grayscale, brightness, contrast and hue) to elements, previously only possible by using SVG.
*
* https://www.w3.org/TR/filter-effects-1/
*/
def filters: Subject = Map(
AndroidBrowser -> Set(Unsupported, FullX, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(FullX),
BaiduBrowser -> Set(FullX),
BlackberryBrowser -> Set(Unsupported, FullX),
Chrome -> Set(Unsupported, FullX, Full),
Edge -> Set(Unsupported, Partial),
Firefox -> Set(Unsupported, Partial, Full),
IE -> Set(Unsupported),
IEMobile -> Set(Unsupported),
IOSSafari -> Set(Unsupported, FullX, Full),
Opera -> Set(Unsupported, FullX, Full),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported, FullX),
QQBrowser -> Set(FullX),
Safari -> Set(Unsupported, FullX, Full),
Samsung -> Set(FullX))
/**
* CSS position:fixed
*
* Method of keeping an element in a fixed location regardless of scroll position
*
* https://www.w3.org/TR/CSS21/visuren.html#fixed-positioning
*/
def fixed: Subject = Map(
AndroidBrowser -> Set(Partial, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Full),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(Full),
Chrome -> Set(Full),
Edge -> Set(Full),
Firefox -> Set(Full),
IE -> Set(Unsupported, Full),
IEMobile -> Set(Full),
IOSSafari -> Set(Unsupported, Partial, Full),
Opera -> Set(Full),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Full),
QQBrowser -> Set(Full),
Safari -> Set(Full),
Samsung -> Set(Full))
/**
* CSS Flexible Box Layout Module
*
* Method of positioning elements in horizontal or vertical stacks. Support includes all properties prefixed with `flex`, as well as `display: flex`, `display: inline-flex`, `align-content`, `align-items`, `align-self`, `justify-content` and `order`.
*
* https://www.w3.org/TR/css3-flexbox/
*/
val flexbox: Subject = Map(
AndroidBrowser -> Set(PartialX, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(PartialX),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(PartialX, Full),
Chrome -> Set(PartialX, FullX, Full),
Edge -> Set(Full),
Firefox -> Set(PartialX, Partial, Full),
IE -> Set(Unsupported, PartialX, Partial),
IEMobile -> Set(PartialX, Full),
IOSSafari -> Set(PartialX, FullX, Full),
Opera -> Set(Unsupported, Full, FullX),
OperaMini -> Set(Full),
OperaMobile -> Set(Unsupported, Full),
QQBrowser -> Set(Full),
Safari -> Set(PartialX, FullX, Full),
Samsung -> Set(Full))
/**
* display: flow-root
*
* The element generates a block container box, and lays out its contents using flow layout. It always establishes a new block formatting context for its contents. The result is the same as the "clearfix" hack.
*
* https://www.w3.org/TR/css-display-3/#valdef-display-flow-root
*/
def flowRoot: Subject = Map(
AndroidBrowser -> Set(Unsupported, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Unsupported),
BaiduBrowser -> Set(Unsupported),
BlackberryBrowser -> Set(Unsupported),
Chrome -> Set(Unsupported, Full),
Edge -> Set(Unsupported),
Firefox -> Set(Unsupported, Full),
IE -> Set(Unsupported),
IEMobile -> Set(Unsupported),
IOSSafari -> Set(Unsupported),
Opera -> Set(Unsupported, Full),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported),
QQBrowser -> Set(Unsupported),
Safari -> Set(Unsupported),
Samsung -> Set(Unsupported))
/**
* system-ui value for font-family
*
* Value for `font-family` that represents the default user interface font.
*
* https://drafts.csswg.org/css-fonts-4/#system-ui-def
*/
def fontFamilySystemUi: Subject = Map(
AndroidBrowser -> Set(Unsupported, Full),
AndroidChrome -> Set(Unsupported),
AndroidFirefox -> Set(Unsupported),
AndroidUC -> Set(Full),
BaiduBrowser -> Set(Unsupported),
BlackberryBrowser -> Set(Unsupported, Unknown),
Chrome -> Set(Unsupported, Full),
Edge -> Set(Unsupported),
Firefox -> Set(Unsupported, Partial),
IE -> Set(Unsupported),
IEMobile -> Set(Unsupported),
IOSSafari -> Set(Unsupported, Partial, Full),
Opera -> Set(Unsupported, Full),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported, Full),
QQBrowser -> Set(Full),
Safari -> Set(Unsupported, Unknown, Partial, Full),
Samsung -> Set(Unsupported, Full))
/**
* CSS font-feature-settings
*
* Method of applying advanced typographic and language-specific font features to supported OpenType fonts.
*
* http://w3.org/TR/css3-fonts/#font-rend-props
*/
def fontFeature: Subject = Map(
AndroidBrowser -> Set(Unsupported, FullX, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(FullX),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(Unsupported, FullX),
Chrome -> Set(Unsupported, PartialX, FullX, Full),
Edge -> Set(Full),
Firefox -> Set(Unsupported, PartialX, FullX, Full),
IE -> Set(Unsupported, Full),
IEMobile -> Set(Unsupported),
IOSSafari -> Set(Partial, Unsupported, Full),
Opera -> Set(Unsupported, FullX, Full),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported, Full),
QQBrowser -> Set(Full),
Safari -> Set(Unsupported, Partial, Full),
Samsung -> Set(FullX, Full))
/**
* CSS3 font-kerning
*
* Controls the usage of the kerning information (spacing between letters) stored in the font. Note that this only affects OpenType fonts with kerning information, it has no effect on other fonts.
*
* https://www.w3.org/TR/css3-fonts/#font-kerning-prop
*/
def fontKerning: Subject = Map(
AndroidBrowser -> Set(Unsupported, FullX, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Full),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(Unsupported, FullX),
Chrome -> Set(Unsupported, FullX, Full),
Edge -> Set(Unsupported),
Firefox -> Set(Unsupported, Full),
IE -> Set(Unsupported),
IEMobile -> Set(Unsupported),
IOSSafari -> Set(Unsupported, FullX),
Opera -> Set(Unsupported, FullX, Full),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported, Full),
QQBrowser -> Set(Full),
Safari -> Set(Unsupported, FullX, Full),
Samsung -> Set(Full))
/**
* CSS font-size-adjust
*
* Method of adjusting the font size in a matter that relates to the height of lowercase vs. uppercase letters. This makes it easier to set the size of fallback fonts.
*
* https://www.w3.org/TR/css-fonts-3/#font-size-adjust-prop
*/
def fontSizeAdjust: Subject = Map(
AndroidBrowser -> Set(Unsupported),
AndroidChrome -> Set(Unsupported),
AndroidFirefox -> Set(Unsupported),
AndroidUC -> Set(Unsupported),
BaiduBrowser -> Set(Unsupported),
BlackberryBrowser -> Set(Unsupported),
Chrome -> Set(Unsupported),
Edge -> Set(Unsupported),
Firefox -> Set(Unsupported, Full),
IE -> Set(Unsupported),
IEMobile -> Set(Unsupported),
IOSSafari -> Set(Unsupported),
Opera -> Set(Unsupported),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported),
QQBrowser -> Set(Unsupported),
Safari -> Set(Unsupported),
Samsung -> Set(Unsupported))
/**
* CSS font-stretch
*
* If a font has multiple types of variations based on the width of characters, the `font-stretch` property allows the appropriate one to be selected. The property in itself does not cause the browser to stretch to a font.
*
* https://www.w3.org/TR/css-fonts-3/#font-stretch-prop
*/
def fontStretch: Subject = Map(
AndroidBrowser -> Set(Unsupported, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Unsupported),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(Unsupported),
Chrome -> Set(Unsupported, Full),
Edge -> Set(Full),
Firefox -> Set(Unsupported, Full),
IE -> Set(Unsupported, Full),
IEMobile -> Set(Full),
IOSSafari -> Set(Unsupported),
Opera -> Set(Unsupported, Full),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported, Full),
QQBrowser -> Set(Unsupported),
Safari -> Set(Unsupported, Full),
Samsung -> Set(Unsupported, Full))
/**
* CSS Generated content for pseudo-elements
*
* Method of displaying text or images before or after the given element's contents using the ::before and ::after pseudo-elements. All browsers with support also support the `attr()` notation in the `content` property.
*
* https://www.w3.org/TR/CSS21/generate.html
*/
def gencontent: Subject = Map(
AndroidBrowser -> Set(Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Full),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(Full),
Chrome -> Set(Full),
Edge -> Set(Full),
Firefox -> Set(Full),
IE -> Set(Unsupported, Partial, Full),
IEMobile -> Set(Full),
IOSSafari -> Set(Full),
Opera -> Set(Full),
OperaMini -> Set(Full),
OperaMobile -> Set(Full),
QQBrowser -> Set(Full),
Safari -> Set(Full),
Samsung -> Set(Full))
/**
* CSS Gradients
*
* Method of defining a linear or radial color gradient as a CSS image.
*
* https://www.w3.org/TR/css3-images/
*/
def gradients: Subject = Map(
AndroidBrowser -> Set(PartialX, FullX, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Full),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(PartialX, Full),
Chrome -> Set(PartialX, FullX, Full),
Edge -> Set(Full),
Firefox -> Set(Unsupported, FullX, Full),
IE -> Set(Unsupported, Full),
IEMobile -> Set(Full),
IOSSafari -> Set(PartialX, FullX, Full),
Opera -> Set(Unsupported, PartialX, FullX, Full),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported, PartialX, FullX, Full),
QQBrowser -> Set(Full),
Safari -> Set(Unsupported, PartialX, FullX, Full),
Samsung -> Set(Full))
/**
* CSS Grid Layout
*
* Method of using a grid concept to lay out content, providing a mechanism for authors to divide available space for layout into columns and rows using a set of predictable sizing behaviors. Includes support for all `grid-*` properties and the `fr` unit.
*
* https://www.w3.org/TR/css3-grid-layout/
*/
val grid: Subject = Map(
AndroidBrowser -> Set(Unsupported, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Unsupported),
BaiduBrowser -> Set(Unsupported),
BlackberryBrowser -> Set(Unsupported),
Chrome -> Set(Unsupported, Full),
Edge -> Set(PartialX, Full),
Firefox -> Set(Unsupported, Full),
IE -> Set(Unsupported, PartialX),
IEMobile -> Set(PartialX),
IOSSafari -> Set(Unsupported, Full),
Opera -> Set(Unsupported, Full),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported),
QQBrowser -> Set(Unsupported),
Safari -> Set(Unsupported, Full),
Samsung -> Set(Unsupported, Full))
/**
* CSS hanging-punctuation
*
* Allows some punctuation characters from start (or the end) of text elements to be placed "outside" of the box in order to preserve the reading flow.
*
* https://drafts.csswg.org/css-text-3/#hanging-punctuation-property
*/
def hangingPunctuation: Subject = Map(
AndroidBrowser -> Set(Unsupported),
AndroidChrome -> Set(Unsupported),
AndroidFirefox -> Set(Unsupported),
AndroidUC -> Set(Unsupported),
BaiduBrowser -> Set(Unsupported),
BlackberryBrowser -> Set(Unsupported),
Chrome -> Set(Unsupported),
Edge -> Set(Unsupported, Unknown),
Firefox -> Set(Unsupported),
IE -> Set(Unsupported),
IEMobile -> Set(Unsupported),
IOSSafari -> Set(Unsupported, Full),
Opera -> Set(Unsupported),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported),
QQBrowser -> Set(Unsupported),
Safari -> Set(Unsupported, Full),
Samsung -> Set(Unsupported))
/**
* CSS Hyphenation
*
* Method of controlling when words at the end of lines should be hyphenated using the "hyphens" property.
*
* https://www.w3.org/TR/css3-text/#hyphenation
*/
def hyphens: Subject = Map(
AndroidBrowser -> Set(Unsupported, Partial),
AndroidChrome -> Set(Partial),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(PartialX),
BaiduBrowser -> Set(Partial),
BlackberryBrowser -> Set(Unsupported),
Chrome -> Set(Unsupported, Partial),
Edge -> Set(FullX),
Firefox -> Set(Unsupported, FullX, Full),
IE -> Set(Unsupported, FullX),
IEMobile -> Set(Unsupported),
IOSSafari -> Set(Unsupported, FullX),
Opera -> Set(Unsupported, Partial),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported),
QQBrowser -> Set(Unsupported),
Safari -> Set(Unsupported, FullX),
Samsung -> Set(Unsupported, Partial, Full))
/**
* CSS3 image-orientation
*
* CSS property used generally to fix the intended orientation of an image. This can be done using 90 degree increments or based on the image's EXIF data using the "from-image" value.
*
* https://www.w3.org/TR/css3-images/#image-orientation
*/
def imageOrientation: Subject = Map(
AndroidBrowser -> Set(Unsupported),
AndroidChrome -> Set(Unsupported),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Unsupported),
BaiduBrowser -> Set(Unsupported),
BlackberryBrowser -> Set(Unsupported),
Chrome -> Set(Unsupported),
Edge -> Set(Unsupported),
Firefox -> Set(Unsupported, Full),
IE -> Set(Unsupported),
IEMobile -> Set(Unsupported),
IOSSafari -> Set(Partial),
Opera -> Set(Unsupported),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported),
QQBrowser -> Set(Unsupported),
Safari -> Set(Unsupported),
Samsung -> Set(Unsupported))
/**
* CSS image-set
*
* Method of letting the browser pick the most appropriate CSS background image from a given set, primarily for high PPI screens.
*
* https://drafts.csswg.org/css-images-4/#image-set-notation
*/
def imageSet: Subject = Map(
AndroidBrowser -> Set(Unsupported, FullX),
AndroidChrome -> Set(FullX),
AndroidFirefox -> Set(Unsupported),
AndroidUC -> Set(FullX),
BaiduBrowser -> Set(FullX),
BlackberryBrowser -> Set(Unsupported, FullX),
Chrome -> Set(Unsupported, FullX),
Edge -> Set(Unsupported),
Firefox -> Set(Unsupported),
IE -> Set(Unsupported),
IEMobile -> Set(Unsupported),
IOSSafari -> Set(Unsupported, FullX),
Opera -> Set(Unsupported, FullX),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported, FullX),
QQBrowser -> Set(FullX),
Safari -> Set(Unsupported, FullX),
Samsung -> Set(FullX))
/**
* CSS Initial Letter
*
* Method of creating an enlarged cap, including a drop or raised cap, in a robust way.
*
* https://www.w3.org/TR/css-inline/#initial-letter-styling
*/
def initialLetter: Subject = Map(
AndroidBrowser -> Set(Unsupported),
AndroidChrome -> Set(Unsupported),
AndroidFirefox -> Set(Unsupported),
AndroidUC -> Set(Unsupported),
BaiduBrowser -> Set(Unsupported),
BlackberryBrowser -> Set(Unsupported),
Chrome -> Set(Unsupported),
Edge -> Set(Unsupported),
Firefox -> Set(Unsupported),
IE -> Set(Unsupported),
IEMobile -> Set(Unsupported),
IOSSafari -> Set(Unsupported, PartialX),
Opera -> Set(Unsupported),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported),
QQBrowser -> Set(Unsupported),
Safari -> Set(Unsupported, Partial, PartialX),
Samsung -> Set(Unsupported))
/**
* Intrinsic & Extrinsic Sizing
*
* Allows for the heights and widths to be specified in intrinsic values using the `max-content`, `min-content`, `fit-content` and `stretch` (formerly `fill`) properties.
*
* https://www.w3.org/TR/css3-sizing/
*/
def intrinsicWidth: Subject = Map(
AndroidBrowser -> Set(Unsupported, FullX, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(PartialX),
AndroidUC -> Set(FullX),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(Unsupported, FullX),
Chrome -> Set(Unsupported, FullX, Full),
Edge -> Set(Unsupported),
Firefox -> Set(Unsupported, PartialX),
IE -> Set(Unsupported),
IEMobile -> Set(Unsupported),
IOSSafari -> Set(Unsupported, PartialX),
Opera -> Set(Unsupported, FullX, Full),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported, Full),
QQBrowser -> Set(FullX),
Safari -> Set(Unsupported, PartialX, Partial),
Samsung -> Set(FullX, Full))
/**
* letter-spacing CSS property
*
* Controls spacing between characters of text (i.e. "tracking" in typographical terms). Not to be confused with kerning.
*
* https://www.w3.org/TR/CSS2/text.html#propdef-letter-spacing
*/
def letterSpacing: Subject = Map(
AndroidBrowser -> Set(Unknown, Partial, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Full),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(Partial),
Chrome -> Set(Partial, Full),
Edge -> Set(Full),
Firefox -> Set(Full),
IE -> Set(Unknown, Partial, Full),
IEMobile -> Set(Full),
IOSSafari -> Set(Unknown, Full),
Opera -> Set(Unknown, Partial, Full),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Partial, Full),
QQBrowser -> Set(Full),
Safari -> Set(Unknown, Partial, Full),
Samsung -> Set(Full))
/**
* CSS Logical Properties
*
* Use start/end properties that depend on LTR or RTL writing direction instead of left/right
*
* https://www.w3.org/TR/css-logical-1/
*/
def logicalProps: Subject = Map(
AndroidBrowser -> Set(PartialX),
AndroidChrome -> Set(PartialX),
AndroidFirefox -> Set(PartialX),
AndroidUC -> Set(PartialX),
BaiduBrowser -> Set(PartialX),
BlackberryBrowser -> Set(PartialX),
Chrome -> Set(PartialX),
Edge -> Set(Unsupported),
Firefox -> Set(Unsupported, PartialX, Full),
IE -> Set(Unsupported),
IEMobile -> Set(Unsupported),
IOSSafari -> Set(PartialX),
Opera -> Set(Unsupported, PartialX),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported, PartialX),
QQBrowser -> Set(PartialX),
Safari -> Set(PartialX),
Samsung -> Set(PartialX))
/**
* CSS Masks
*
* Method of displaying part of an element, using a selected image as a mask
*
* https://www.w3.org/TR/css-masking-1/
*/
val masks: Subject = Map(
AndroidBrowser -> Set(PartialX),
AndroidChrome -> Set(PartialX),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(PartialX),
BaiduBrowser -> Set(PartialX),
BlackberryBrowser -> Set(PartialX),
Chrome -> Set(PartialX),
Edge -> Set(Unsupported),
Firefox -> Set(Unsupported, Partial, Full),
IE -> Set(Unsupported),
IEMobile -> Set(Unsupported),
IOSSafari -> Set(PartialX),
Opera -> Set(Unsupported, PartialX),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported, PartialX),
QQBrowser -> Set(PartialX),
Safari -> Set(Unsupported, PartialX),
Samsung -> Set(PartialX))
/**
* Media Queries: resolution feature
*
* Allows a media query to be set based on the device pixels used per CSS unit. While the standard uses `min`/`max-resolution` for this, some browsers support the older non-standard `device-pixel-ratio` media query.
*
* https://www.w3.org/TR/css3-mediaqueries/#resolution
*/
def mediaResolution: Subject = Map(
AndroidBrowser -> Set(Unknown, PartialX, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Full),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(PartialX),
Chrome -> Set(PartialX, Full),
Edge -> Set(Full),
Firefox -> Set(Unsupported, Partial, Full),
IE -> Set(Unsupported, Partial),
IEMobile -> Set(Partial),
IOSSafari -> Set(Unknown, PartialX),
Opera -> Set(Unsupported, PartialX, Full),
OperaMini -> Set(Partial),
OperaMobile -> Set(PartialX, Full),
QQBrowser -> Set(Full),
Safari -> Set(Unsupported, PartialX),
Samsung -> Set(Full))
/**
* CSS3 Multiple column layout
*
* Method of flowing information in multiple columns
*
* https://www.w3.org/TR/css3-multicol/
*/
val multicolumn: Subject = Map(
AndroidBrowser -> Set(PartialX, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Partial),
AndroidUC -> Set(PartialX),
BaiduBrowser -> Set(Partial),
BlackberryBrowser -> Set(PartialX),
Chrome -> Set(PartialX, Full),
Edge -> Set(Full),
Firefox -> Set(PartialX, Partial),
IE -> Set(Unsupported, Full),
IEMobile -> Set(Full),
IOSSafari -> Set(PartialX, Partial, Full),
Opera -> Set(Unsupported, Full, PartialX),
OperaMini -> Set(Full),
OperaMobile -> Set(Unsupported, Full, Partial),
QQBrowser -> Set(Partial),
Safari -> Set(PartialX, Partial, Full),
Samsung -> Set(PartialX, Full))
/**
* CSS3 object-fit/object-position
*
* Method of specifying how an object (image or video) should fit inside its box. object-fit options include "contain" (fit according to aspect ratio), "fill" (stretches object to fill) and "cover" (overflows box but maintains ratio), where object-position allows the object to be repositioned like background-image does.
*
* https://www.w3.org/TR/css3-images/
*/
def objectFit: Subject = Map(
AndroidBrowser -> Set(Unsupported, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Full),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(Unsupported),
Chrome -> Set(Unsupported, Full),
Edge -> Set(Unsupported, Partial),
Firefox -> Set(Unsupported, Full),
IE -> Set(Unsupported),
IEMobile -> Set(Unsupported),
IOSSafari -> Set(Unsupported, Partial, Full),
Opera -> Set(Unsupported, FullX, Full),
OperaMini -> Set(FullX),
OperaMobile -> Set(Unsupported, FullX, Full),
QQBrowser -> Set(Full),
Safari -> Set(Unsupported, Partial, Full),
Samsung -> Set(Full))
/**
* CSS3 Opacity
*
* Method of setting the transparency level of an element
*
* https://www.w3.org/TR/css3-color/
*/
def opacity: Subject = Map(
AndroidBrowser -> Set(Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Full),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(Full),
Chrome -> Set(Full),
Edge -> Set(Full),
Firefox -> Set(Full),
IE -> Set(Partial, Full),
IEMobile -> Set(Full),
IOSSafari -> Set(Full),
Opera -> Set(Full),
OperaMini -> Set(Full),
OperaMobile -> Set(Full),
QQBrowser -> Set(Full),
Safari -> Set(Full),
Samsung -> Set(Full))
/**
* CSS outline properties
*
* The CSS outline properties draw a border around an element that does not affect layout, making it ideal for highlighting. This covers the `outline` shorthand, as well as `outline-width`, `outline-style`, `outline-color` and `outline-offset`.
*
* https://drafts.csswg.org/css-ui/
*/
val outline: Subject = Map(
AndroidBrowser -> Set(Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Full),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(Full),
Chrome -> Set(Full),
Edge -> Set(Partial, Full),
Firefox -> Set(Full),
IE -> Set(Unsupported, Partial),
IEMobile -> Set(Partial),
IOSSafari -> Set(Full),
Opera -> Set(Partial, Full),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Partial, Full),
QQBrowser -> Set(Full),
Safari -> Set(Full),
Samsung -> Set(Full))
/**
* CSS overflow-anchor (Scroll Anchoring)
*
* Changes in DOM elements above the visible region of a scrolling box can result in the page moving while the user is in the middle of consuming the content.
By default, the value of `overflow-anchor` is `auto`, it can mitigate this jarring user experience by keeping track of the position of an anchor node and adjusting the scroll offset accordingly
*
* https://wicg.github.io/ScrollAnchoring/
*/
def overflowAnchor: Subject = Map(
AndroidBrowser -> Set(Unsupported, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Unsupported),
AndroidUC -> Set(Unsupported),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(Unsupported),
Chrome -> Set(Unsupported, Full),
Edge -> Set(Unsupported),
Firefox -> Set(Unsupported),
IE -> Set(Unsupported),
IEMobile -> Set(Unsupported),
IOSSafari -> Set(Unsupported),
Opera -> Set(Unsupported, Full),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported),
QQBrowser -> Set(Unsupported),
Safari -> Set(Unsupported),
Samsung -> Set(Unsupported, Full))
/**
* CSS overscroll-behavior
*
* CSS property to control the behavior when the scroll position of a scroll container reaches the edge of the scrollport.
*
* https://wicg.github.io/overscroll-behavior/
*/
def overscrollBehavior: Subject = Map(
AndroidBrowser -> Set(Unsupported),
AndroidChrome -> Set(Partial),
AndroidFirefox -> Set(Unsupported),
AndroidUC -> Set(Unsupported),
BaiduBrowser -> Set(Unsupported),
BlackberryBrowser -> Set(Unsupported),
Chrome -> Set(Unsupported, Partial, Full),
Edge -> Set(Partial),
Firefox -> Set(Unsupported, Full),
IE -> Set(Unsupported, Partial),
IEMobile -> Set(Partial),
IOSSafari -> Set(Unsupported),
Opera -> Set(Unsupported, Partial),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported),
QQBrowser -> Set(Unsupported),
Safari -> Set(Unsupported),
Samsung -> Set(Unsupported))
/**
* CSS page-break properties
*
* Properties to control the way elements are broken across (printed) pages.
*
* https://www.w3.org/TR/CSS2/page.html#page-breaks
*/
def pageBreak: Subject = Map(
AndroidBrowser -> Set(Partial),
AndroidChrome -> Set(Partial),
AndroidFirefox -> Set(Partial),
AndroidUC -> Set(Partial),
BaiduBrowser -> Set(Partial),
BlackberryBrowser -> Set(Partial),
Chrome -> Set(Partial),
Edge -> Set(Partial),
Firefox -> Set(Partial),
IE -> Set(Partial),
IEMobile -> Set(Partial),
IOSSafari -> Set(Partial),
Opera -> Set(Unknown, Full, Partial),
OperaMini -> Set(Full),
OperaMobile -> Set(Full, Partial),
QQBrowser -> Set(Partial),
Safari -> Set(Partial),
Samsung -> Set(Partial))
/**
* CSS Reflections
*
* Method of displaying a reflection of an element
*
* http://webkit.org/blog/182/css-reflections/
*/
def reflections: Subject = Map(
AndroidBrowser -> Set(FullX),
AndroidChrome -> Set(FullX),
AndroidFirefox -> Set(Unsupported),
AndroidUC -> Set(Unsupported),
BaiduBrowser -> Set(FullX),
BlackberryBrowser -> Set(FullX),
Chrome -> Set(FullX),
Edge -> Set(Unsupported),
Firefox -> Set(Unsupported),
IE -> Set(Unsupported),
IEMobile -> Set(Unsupported),
IOSSafari -> Set(FullX),
Opera -> Set(Unsupported, FullX),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported, FullX),
QQBrowser -> Set(FullX),
Safari -> Set(Unsupported, FullX),
Samsung -> Set(FullX))
/**
* CSS Regions
*
* Method of flowing content into multiple elements.
*
* https://www.w3.org/TR/css3-regions/
*/
def regions: Subject = Map(
AndroidBrowser -> Set(Unsupported),
AndroidChrome -> Set(Unsupported),
AndroidFirefox -> Set(Unsupported),
AndroidUC -> Set(Unsupported),
BaiduBrowser -> Set(Unsupported),
BlackberryBrowser -> Set(Unsupported),
Chrome -> Set(Unsupported, PartialX),
Edge -> Set(PartialX),
Firefox -> Set(Unsupported),
IE -> Set(Unsupported, PartialX),
IEMobile -> Set(PartialX),
IOSSafari -> Set(Unsupported, FullX),
Opera -> Set(Unsupported),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported),
QQBrowser -> Set(Unsupported),
Safari -> Set(Unsupported, FullX),
Samsung -> Set(Unsupported))
/**
* CSS Repeating Gradients
*
* Method of defining a repeating linear or radial color gradient as a CSS image.
*
* https://www.w3.org/TR/css3-images/#repeating-gradients
*/
def repeatingGradients: Subject = Map(
AndroidBrowser -> Set(Unsupported, FullX, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Full),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(Unsupported, Full),
Chrome -> Set(Unsupported, FullX, Full),
Edge -> Set(Full),
Firefox -> Set(Unsupported, FullX, Full),
IE -> Set(Unsupported, Full),
IEMobile -> Set(Full),
IOSSafari -> Set(Unsupported, FullX, Full),
Opera -> Set(Unsupported, PartialX, FullX, Full),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported, PartialX, FullX, Full),
QQBrowser -> Set(Full),
Safari -> Set(Unsupported, FullX, Full),
Samsung -> Set(Full))
/**
* CSS resize property
*
* Method of allowing an element to be resized by the user, with options to limit to a given direction.
*
* https://www.w3.org/TR/css3-ui/#resize
*/
def resize: Subject = Map(
AndroidBrowser -> Set(Unsupported, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Full),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(Unsupported),
Chrome -> Set(Full),
Edge -> Set(Unsupported),
Firefox -> Set(Unsupported, FullX, Full),
IE -> Set(Unsupported),
IEMobile -> Set(Unsupported),
IOSSafari -> Set(Unsupported),
Opera -> Set(Unsupported, Partial, Full),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported, Full),
QQBrowser -> Set(Full),
Safari -> Set(Unsupported, Full),
Samsung -> Set(Unsupported, Full))
/**
* display: run-in
*
* If the run-in box contains a block box, same as block. If a block box follows the run-in box, the run-in box becomes the first inline box of the block box. If an inline box follows, the run-in box becomes a block box.
*
* https://drafts.csswg.org/css-display/#valdef-display-run-in
*/
def runIn: Subject = Map(
AndroidBrowser -> Set(Full, Unsupported),
AndroidChrome -> Set(Unsupported),
AndroidFirefox -> Set(Unsupported),
AndroidUC -> Set(Unsupported),
BaiduBrowser -> Set(Unsupported),
BlackberryBrowser -> Set(Unknown),
Chrome -> Set(Full, Unsupported),
Edge -> Set(Unsupported),
Firefox -> Set(Unsupported),
IE -> Set(Unsupported, Full),
IEMobile -> Set(Full),
IOSSafari -> Set(Full, Unsupported),
Opera -> Set(Full, Unsupported),
OperaMini -> Set(Full),
OperaMobile -> Set(Full, Unsupported),
QQBrowser -> Set(Unsupported),
Safari -> Set(Full, Unknown, Unsupported),
Samsung -> Set(Unsupported))
/**
* CSSOM Scroll-behavior
*
* Method of specifying the scrolling behavior for a scrolling box, when scrolling happens due to navigation or CSSOM scrolling APIs.
*
* https://drafts.csswg.org/cssom-view/#propdef-scroll-behavior
*/
def scrollBehavior: Subject = Map(
AndroidBrowser -> Set(Unsupported),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Unsupported),
AndroidUC -> Set(Unsupported),
BaiduBrowser -> Set(Unsupported),
BlackberryBrowser -> Set(Unsupported),
Chrome -> Set(Unsupported, Full),
Edge -> Set(Unsupported),
Firefox -> Set(Unsupported, Full),
IE -> Set(Unsupported),
IEMobile -> Set(Unsupported),
IOSSafari -> Set(Unsupported),
Opera -> Set(Unsupported, Full),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported),
QQBrowser -> Set(Unsupported),
Safari -> Set(Unsupported),
Samsung -> Set(Unsupported))
/**
* CSS 2.1 selectors
*
* Basic CSS selectors including: `*` (universal selector), `>` (child selector), `:first-child`, `:link`, `:visited`, `:active`, `:hover`, `:focus`, `:lang()`, `+` (adjacent sibling selector), `[attr]`, `[attr="val"]`, `[attr~="val"]`, `[attr|="bar"]`, `.foo` (class selector), `#foo` (id selector)
*
* https://www.w3.org/TR/CSS21/selector.html
*/
def sel2: Subject = Map(
AndroidBrowser -> Set(Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Full),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(Full),
Chrome -> Set(Full),
Edge -> Set(Full),
Firefox -> Set(Full),
IE -> Set(Unsupported, Full),
IEMobile -> Set(Full),
IOSSafari -> Set(Full),
Opera -> Set(Full),
OperaMini -> Set(Full),
OperaMobile -> Set(Full),
QQBrowser -> Set(Full),
Safari -> Set(Full),
Samsung -> Set(Full))
/**
* CSS3 selectors
*
* Advanced element selection using selectors including: `[foo^="bar"]`, `[foo$="bar"]`, `[foo*="bar"]`, `:root`, `:nth-child()`, `:nth-last-child()`, `nth-of-type`, `nth-last-of-type()`, `:last-child`, `:first-of-type`, `:last-of-type`, `:only-child`, `:only-of-type`, `:empty`, `:target`, `:enabled`, `:disabled`, `:checked`, `:not()`, `~` (general sibling)
*
* https://www.w3.org/TR/css3-selectors/
*/
def sel3: Subject = Map(
AndroidBrowser -> Set(Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Full),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(Full),
Chrome -> Set(Full),
Edge -> Set(Full),
Firefox -> Set(Unsupported, Full),
IE -> Set(Unsupported, Partial, Full),
IEMobile -> Set(Full),
IOSSafari -> Set(Full),
Opera -> Set(Unsupported, Full),
OperaMini -> Set(Full),
OperaMobile -> Set(Full),
QQBrowser -> Set(Full),
Safari -> Set(Unsupported, Full),
Samsung -> Set(Full))
/**
* ::selection CSS pseudo-element
*
* The ::selection CSS pseudo-element applies rules to the portion of a document that has been highlighted (e.g., selected with the mouse or another pointing device) by the user.
*
* https://www.w3.org/TR/css-pseudo-4/#selectordef-selection
*/
def selection: Subject = Map(
AndroidBrowser -> Set(Unsupported, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(FullX),
AndroidUC -> Set(Unsupported),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(Unsupported, Full),
Chrome -> Set(Full),
Edge -> Set(Full),
Firefox -> Set(FullX),
IE -> Set(Unsupported, Full),
IEMobile -> Set(Full),
IOSSafari -> Set(Unsupported),
Opera -> Set(Unsupported, Full),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unknown, Full),
QQBrowser -> Set(Full),
Safari -> Set(Full),
Samsung -> Set(Full))
/**
* CSS Shapes Level 1
*
* Allows geometric shapes to be set in CSS to define an area for text to flow around.
*
* https://www.w3.org/TR/css-shapes/
*/
val shapes: Subject = Map(
AndroidBrowser -> Set(Unsupported, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Unsupported),
AndroidUC -> Set(Full),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(Unsupported),
Chrome -> Set(Unsupported, Full),
Edge -> Set(Unsupported),
Firefox -> Set(Unsupported),
IE -> Set(Unsupported),
IEMobile -> Set(Unsupported),
IOSSafari -> Set(Unsupported, FullX, Full),
Opera -> Set(Unsupported, Full),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported, Full),
QQBrowser -> Set(Full),
Safari -> Set(Unsupported, FullX, Full),
Samsung -> Set(Full))
/**
* CSS position:sticky
*
* Keeps elements positioned as "fixed" or "relative" depending on how it appears in the viewport. As a result the element is "stuck" when necessary while scrolling.
*
* https://drafts.csswg.org/css-position/#sticky-pos
*/
def sticky: Subject = Map(
AndroidBrowser -> Set(Unsupported, Partial),
AndroidChrome -> Set(Partial),
AndroidFirefox -> Set(Partial),
AndroidUC -> Set(Unsupported),
BaiduBrowser -> Set(Unsupported),
BlackberryBrowser -> Set(Unsupported),
Chrome -> Set(Unsupported, Partial, Full),
Edge -> Set(Unsupported, Full),
Firefox -> Set(Unsupported, Partial, Full),
IE -> Set(Unsupported),
IEMobile -> Set(Unsupported),
IOSSafari -> Set(Unsupported, PartialX, FullX),
Opera -> Set(Unsupported, Partial),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported),
QQBrowser -> Set(Unsupported),
Safari -> Set(Unsupported, PartialX, FullX),
Samsung -> Set(Unsupported, Full))
/**
* CSS3 text-align-last
*
* CSS property to describe how the last line of a block or a line right before a forced line break when `text-align` is `justify`.
*
* https://www.w3.org/TR/css3-text/#text-align-last-property
*/
def textAlignLast: Subject = Map(
AndroidBrowser -> Set(Unsupported, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Unsupported),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(Unsupported),
Chrome -> Set(Unsupported, Full),
Edge -> Set(Partial),
Firefox -> Set(Unsupported, FullX, Full),
IE -> Set(Partial),
IEMobile -> Set(Partial),
IOSSafari -> Set(Unsupported),
Opera -> Set(Unsupported, Full),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported),
QQBrowser -> Set(Unsupported),
Safari -> Set(Unsupported),
Samsung -> Set(Unsupported, Full))
/**
* text-decoration styling
*
* Method of defining the type, style and color of lines in the text-decoration property. These can be defined as shorthand (e.g. `text-decoration: line-through dashed blue`) or as single properties (e.g. `text-decoration-color: blue`)
*
* https://www.w3.org/TR/css-text-decor-3/#line-decoration
*/
val textDecoration: Subject = Map(
AndroidBrowser -> Set(Unsupported, Full),
AndroidChrome -> Set(Partial),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Unsupported),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(Unsupported),
Chrome -> Set(Unsupported, Partial),
Edge -> Set(Unsupported),
Firefox -> Set(Unsupported, PartialX, Partial),
IE -> Set(Unsupported),
IEMobile -> Set(Unsupported),
IOSSafari -> Set(Unsupported, PartialX),
Opera -> Set(Unsupported, Partial),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported),
QQBrowser -> Set(Unsupported),
Safari -> Set(Unsupported, PartialX),
Samsung -> Set(Unsupported))
/**
* text-emphasis styling
*
* Method of using small symbols next to each glyph to emphasize a run of text, commonly used in East Asian languages. The `text-emphasis` shorthand, and its `text-emphasis-style` and `text-emphasis-color` longhands, can be used to apply marks to the text. The `text-emphasis-position` property, which inherits separately, allows setting the emphasis marks' position with respect to the text.
*
* https://drafts.csswg.org/css-text-decor-3/#text-emphasis-property
*/
def textEmphasis: Subject = Map(
AndroidBrowser -> Set(Unsupported, PartialX),
AndroidChrome -> Set(PartialX),
AndroidFirefox -> Set(Unsupported),
AndroidUC -> Set(PartialX),
BaiduBrowser -> Set(PartialX),
BlackberryBrowser -> Set(Unsupported, PartialX),
Chrome -> Set(Unsupported, PartialX),
Edge -> Set(Unsupported),
Firefox -> Set(Unsupported, Full),
IE -> Set(Unsupported),
IEMobile -> Set(Unsupported),
IOSSafari -> Set(Unsupported, Full),
Opera -> Set(Unsupported, PartialX),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported, PartialX),
QQBrowser -> Set(PartialX),
Safari -> Set(Unsupported, PartialX, Full),
Samsung -> Set(PartialX))
/**
* CSS text-indent
*
* The `text-indent` property applies indentation to lines of inline content in a block.
*
* https://drafts.csswg.org/css-text-3/#text-indent-property
*/
def textIndent: Subject = Map(
AndroidBrowser -> Set(Partial),
AndroidChrome -> Set(Partial),
AndroidFirefox -> Set(Partial),
AndroidUC -> Set(Partial),
BaiduBrowser -> Set(Partial),
BlackberryBrowser -> Set(Partial),
Chrome -> Set(Partial),
Edge -> Set(Partial),
Firefox -> Set(Partial),
IE -> Set(Partial),
IEMobile -> Set(Partial),
IOSSafari -> Set(Partial),
Opera -> Set(Partial),
OperaMini -> Set(Partial),
OperaMobile -> Set(Partial),
QQBrowser -> Set(Partial),
Safari -> Set(Partial),
Samsung -> Set(Partial))
/**
* CSS text-justify
*
* CSS property to define how text should be justified when `text-align: justify` is set.
*
* https://drafts.csswg.org/css-text-3/#text-justify-property
*/
def textJustify: Subject = Map(
AndroidBrowser -> Set(Unsupported),
AndroidChrome -> Set(Unsupported),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Unsupported),
BaiduBrowser -> Set(Unsupported),
BlackberryBrowser -> Set(Unsupported),
Chrome -> Set(Unsupported),
Edge -> Set(Partial),
Firefox -> Set(Unsupported, Full),
IE -> Set(Unknown, Partial),
IEMobile -> Set(Partial),
IOSSafari -> Set(Unsupported),
Opera -> Set(Unsupported),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported),
QQBrowser -> Set(Unsupported),
Safari -> Set(Unsupported),
Samsung -> Set(Unsupported))
/**
* CSS text-orientation
*
* The CSS `text-orientation` property specifies the orientation of text within a line. Current values only have an effect in vertical typographic modes (defined with the `writing-mode` property)
*
* https://drafts.csswg.org/css-writing-modes-3/#text-orientation
*/
def textOrientation: Subject = Map(
AndroidBrowser -> Set(Unsupported, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Unsupported),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(Unsupported),
Chrome -> Set(Unsupported, Full),
Edge -> Set(Unsupported),
Firefox -> Set(Unsupported, Full),
IE -> Set(Unsupported),
IEMobile -> Set(Unsupported),
IOSSafari -> Set(Unsupported, Full),
Opera -> Set(Unsupported, Full),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported, Full),
QQBrowser -> Set(Unsupported),
Safari -> Set(Unsupported, Unknown, FullX),
Samsung -> Set(Unsupported, Full))
/**
* CSS3 Text-overflow
*
* Append ellipsis when text overflows its containing element
*
* https://www.w3.org/TR/css3-ui/#text-overflow
*/
def textOverflow: Subject = Map(
AndroidBrowser -> Set(Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Full),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(Full),
Chrome -> Set(Full),
Edge -> Set(Full),
Firefox -> Set(Unsupported, Full),
IE -> Set(Unsupported, Full),
IEMobile -> Set(Full),
IOSSafari -> Set(Full),
Opera -> Set(FullX, Full),
OperaMini -> Set(Full),
OperaMobile -> Set(FullX, Full),
QQBrowser -> Set(Full),
Safari -> Set(Full),
Samsung -> Set(Full))
/**
* CSS text-size-adjust
*
* On mobile devices, the text-size-adjust CSS property allows Web authors to control if and how the text-inflating algorithm is applied to the textual content of the element it is applied to.
*
* https://drafts.csswg.org/css-size-adjust/
*/
def textSizeAdjust: Subject = Map(
AndroidBrowser -> Set(Unsupported, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(FullX),
AndroidUC -> Set(FullX),
BaiduBrowser -> Set(Unsupported),
BlackberryBrowser -> Set(Unsupported),
Chrome -> Set(Unsupported, Full),
Edge -> Set(Unsupported),
Firefox -> Set(Unsupported),
IE -> Set(Unsupported),
IEMobile -> Set(FullX),
IOSSafari -> Set(Unsupported, FullX),
Opera -> Set(Unsupported, Full),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported),
QQBrowser -> Set(Unsupported),
Safari -> Set(Unsupported),
Samsung -> Set(Unsupported, Full))
/**
* CSS text-stroke and text-fill
*
* Method of declaring the outline (stroke) width and color for text.
*
* https://compat.spec.whatwg.org/#text-fill-and-stroking
*/
def textStroke: Subject = Map(
AndroidBrowser -> Set(FullX, Unsupported),
AndroidChrome -> Set(FullX),
AndroidFirefox -> Set(FullX),
AndroidUC -> Set(Unsupported),
BaiduBrowser -> Set(FullX),
BlackberryBrowser -> Set(FullX),
Chrome -> Set(FullX),
Edge -> Set(Unsupported, FullX),
Firefox -> Set(Unsupported, FullX),
IE -> Set(Unsupported),
IEMobile -> Set(Unsupported),
IOSSafari -> Set(PartialX, FullX),
Opera -> Set(Unsupported, FullX),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported, FullX),
QQBrowser -> Set(FullX),
Safari -> Set(FullX, Full),
Samsung -> Set(FullX))
/**
* CSS3 Text-shadow
*
* Method of applying one or more shadow or blur effects to text
*
* https://www.w3.org/TR/css-text-decor-3/#text-shadow-property
*/
def textshadow: Subject = Map(
AndroidBrowser -> Set(Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Full),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(Partial, Full),
Chrome -> Set(Full),
Edge -> Set(Full),
Firefox -> Set(Unsupported, Full),
IE -> Set(Unsupported, Full),
IEMobile -> Set(Full),
IOSSafari -> Set(Full),
Opera -> Set(Unsupported, Full),
OperaMini -> Set(Partial),
OperaMobile -> Set(Full),
QQBrowser -> Set(Full),
Safari -> Set(Partial, Full),
Samsung -> Set(Full))
/**
* CSS touch-action property
*
* touch-action is a CSS property that controls filtering of gesture events, providing developers with a declarative mechanism to selectively disable touch scrolling (in one or both axes) or double-tap-zooming.
*
* https://www.w3.org/TR/pointerevents/#the-touch-action-css-property
*/
def touchAction: Subject = Map(
AndroidBrowser -> Set(Unsupported, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Full),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(Unsupported),
Chrome -> Set(Unsupported, Full),
Edge -> Set(Full),
Firefox -> Set(Unsupported, Full),
IE -> Set(Unsupported, FullX, Full),
IEMobile -> Set(FullX, Full),
IOSSafari -> Set(Unsupported, Partial),
Opera -> Set(Unsupported, Full),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported, Full),
QQBrowser -> Set(Full),
Safari -> Set(Unsupported),
Samsung -> Set(Full))
/**
* Combination of transforms2d & transforms3d.
*
*
*
*
*/
def transforms: Subject = Map(
AndroidBrowser -> Set(Unsupported, FullX, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(FullX),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(FullX),
Chrome -> Set(Unsupported, FullX, Full),
Edge -> Set(Full),
Firefox -> Set(Full, FullX, Unsupported),
IE -> Set(FullX, Unsupported, Full),
IEMobile -> Set(Full),
IOSSafari -> Set(FullX, Full),
Opera -> Set(Unsupported, FullX, Full),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported, Full),
QQBrowser -> Set(Full),
Safari -> Set(Unsupported, FullX, Full),
Samsung -> Set(Full))
/**
* CSS3 2D Transforms
*
* Method of transforming an element including rotating, scaling, etc. Includes support for `transform` as well as `transform-origin` properties.
*
* https://www.w3.org/TR/css3-2d-transforms/
*/
def transforms2d: Subject = Map(
AndroidBrowser -> Set(FullX, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(FullX),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(FullX),
Chrome -> Set(FullX, Full),
Edge -> Set(Full),
Firefox -> Set(Unsupported, FullX, Full),
IE -> Set(Unsupported, FullX, Full),
IEMobile -> Set(Full),
IOSSafari -> Set(FullX, Full),
Opera -> Set(Unsupported, FullX, Full),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported, Full),
QQBrowser -> Set(Full),
Safari -> Set(FullX, Full),
Samsung -> Set(Full))
/**
* CSS3 3D Transforms
*
* Method of transforming an element in the third dimension using the `transform` property. Includes support for the `perspective` property to set the perspective in z-space and the `backface-visibility` property to toggle display of the reverse side of a 3D-transformed element.
*
* https://www.w3.org/TR/css3-3d-transforms/
*/
def transforms3d: Subject = Map(
AndroidBrowser -> Set(Unsupported, FullX, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(FullX),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(FullX),
Chrome -> Set(Unsupported, FullX, Full),
Edge -> Set(Full),
Firefox -> Set(Unsupported, FullX, Full),
IE -> Set(Unsupported, Partial),
IEMobile -> Set(Partial),
IOSSafari -> Set(FullX, Full),
Opera -> Set(Unsupported, FullX, Full),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported, Full),
QQBrowser -> Set(Full),
Safari -> Set(Unsupported, FullX, Full),
Samsung -> Set(Full))
/**
* CSS3 Transitions
*
* Simple method of animating certain properties of an element, with ability to define property, duration, delay and timing function.
*
* https://www.w3.org/TR/css3-transitions/
*/
val transitions: Subject = Map(
AndroidBrowser -> Set(FullX, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Full),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(FullX, Full),
Chrome -> Set(FullX, Full),
Edge -> Set(Full),
Firefox -> Set(Unsupported, PartialX, FullX, Full),
IE -> Set(Unsupported, Full),
IEMobile -> Set(Full),
IOSSafari -> Set(PartialX, FullX, Full),
Opera -> Set(Unsupported, PartialX, FullX, Full),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(PartialX, FullX, Full),
QQBrowser -> Set(Full),
Safari -> Set(PartialX, FullX, Full),
Samsung -> Set(Full))
/**
* CSS user-select: none
*
* Method of preventing text/element selection using CSS.
*
* https://drafts.csswg.org/css-ui-4/#valdef-user-select-none
*/
def userSelectNone: Subject = Map(
AndroidBrowser -> Set(FullX, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(FullX),
AndroidUC -> Set(FullX),
BaiduBrowser -> Set(Unsupported),
BlackberryBrowser -> Set(FullX),
Chrome -> Set(FullX, Full),
Edge -> Set(FullX),
Firefox -> Set(FullX),
IE -> Set(Unsupported, FullX),
IEMobile -> Set(FullX),
IOSSafari -> Set(FullX),
Opera -> Set(Unsupported, FullX, Full),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported, FullX),
QQBrowser -> Set(FullX),
Safari -> Set(FullX),
Samsung -> Set(FullX))
/**
* Variable fonts
*
* OpenType font settings that allows a single font file to behave like multiple fonts: it can contain all the allowed variations in width, weight, slant, optical size, or any other exposed axes of variation as defined by the font designer. Variations can be applied via the `font-variation-settings` property.
*
* https://drafts.csswg.org/css-fonts-4/#font-variation-settings-def
*/
def variableFonts: Subject = Map(
AndroidBrowser -> Set(Unsupported, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Unsupported),
AndroidUC -> Set(Unsupported),
BaiduBrowser -> Set(Unsupported),
BlackberryBrowser -> Set(Unsupported),
Chrome -> Set(Unsupported, Full),
Edge -> Set(Unsupported),
Firefox -> Set(Unsupported),
IE -> Set(Unsupported),
IEMobile -> Set(Unsupported),
IOSSafari -> Set(Unsupported, Full),
Opera -> Set(Unsupported, Full),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported),
QQBrowser -> Set(Unsupported),
Safari -> Set(Unsupported, Full),
Samsung -> Set(Unsupported))
/**
* Viewport units: vw, vh, vmin, vmax
*
* Length units representing a percentage of the current viewport dimensions: width (vw), height (vh), the smaller of the two (vmin), or the larger of the two (vmax).
*
* https://www.w3.org/TR/css3-values/#viewport-relative-lengths
*/
def viewportUnits: Subject = Map(
AndroidBrowser -> Set(Unsupported, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Full),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(Unsupported, Full),
Chrome -> Set(Unsupported, Partial, Full),
Edge -> Set(Partial, Full),
Firefox -> Set(Unsupported, Full),
IE -> Set(Unsupported, Partial),
IEMobile -> Set(Partial),
IOSSafari -> Set(Unsupported, Partial, Full),
Opera -> Set(Unsupported, Full),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported, Full),
QQBrowser -> Set(Full),
Safari -> Set(Unsupported, Partial, Full),
Samsung -> Set(Full))
/**
* CSS widows & orphans
*
* CSS properties to control when lines break across pages or columns by defining the amount of lines that must be left before or after the break.
*
* https://drafts.csswg.org/css-break-3/#widows-orphans
*/
def widowsOrphans: Subject = Map(
AndroidBrowser -> Set(Unsupported, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Unsupported),
AndroidUC -> Set(Full),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(Unsupported),
Chrome -> Set(Unsupported, Full),
Edge -> Set(Full),
Firefox -> Set(Unsupported),
IE -> Set(Unsupported, Full),
IEMobile -> Set(Full),
IOSSafari -> Set(Unsupported, Full),
Opera -> Set(Full),
OperaMini -> Set(Full),
OperaMobile -> Set(Unsupported, Full),
QQBrowser -> Set(Full),
Safari -> Set(Unsupported, Full),
Samsung -> Set(Full))
/**
* CSS3 word-break
*
* Property to prevent or allow words to be broken over multiple lines between letters.
*
* https://www.w3.org/TR/css3-text/#word-break
*/
def wordBreak: Subject = Map(
AndroidBrowser -> Set(Partial, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Partial),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(Partial),
Chrome -> Set(Partial, Full),
Edge -> Set(Full),
Firefox -> Set(Unsupported, Full),
IE -> Set(Full),
IEMobile -> Set(Full),
IOSSafari -> Set(Partial, Full),
Opera -> Set(Unsupported, Partial, Full),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported, Partial),
QQBrowser -> Set(Partial),
Safari -> Set(Partial, Full),
Samsung -> Set(Full))
/**
* CSS3 Overflow-wrap
*
* Allows lines to be broken within words if an otherwise unbreakable string is too long to fit. Currently mostly supported using the `word-wrap` property.
*
* https://www.w3.org/TR/css3-text/#overflow-wrap
*/
def wordwrap: Subject = Map(
AndroidBrowser -> Set(Partial, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(Full),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(Partial, Full),
Chrome -> Set(Partial, Full),
Edge -> Set(Partial),
Firefox -> Set(Unsupported, Partial, Full),
IE -> Set(Partial),
IEMobile -> Set(Partial),
IOSSafari -> Set(Partial, Full),
Opera -> Set(Unsupported, Partial, Full),
OperaMini -> Set(Partial),
OperaMobile -> Set(Partial, Full),
QQBrowser -> Set(Full),
Safari -> Set(Partial, Full),
Samsung -> Set(Full))
/**
* CSS writing-mode property
*
* Property to define whether lines of text are laid out horizontally or vertically and the direction in which blocks progress.
*
* https://drafts.csswg.org/css-writing-modes-3/#block-flow
*/
def writingMode: Subject = Map(
AndroidBrowser -> Set(Unsupported, FullX, Full),
AndroidChrome -> Set(Full),
AndroidFirefox -> Set(Full),
AndroidUC -> Set(FullX),
BaiduBrowser -> Set(Full),
BlackberryBrowser -> Set(FullX),
Chrome -> Set(Unsupported, Unknown, FullX, Full),
Edge -> Set(Full),
Firefox -> Set(Unsupported, Full),
IE -> Set(Partial),
IEMobile -> Set(PartialX),
IOSSafari -> Set(Unknown, FullX, Full),
Opera -> Set(Unsupported, FullX, Full),
OperaMini -> Set(Unsupported),
OperaMobile -> Set(Unsupported, Full),
QQBrowser -> Set(FullX),
Safari -> Set(Unsupported, Unknown, FullX, Full),
Samsung -> Set(FullX, Full))
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy