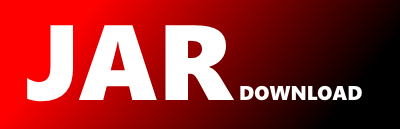
de.japkit.annotationtemplates.BeanValidationAnnotationTemplatesGen Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of japkit-annotationtemplates Show documentation
Show all versions of japkit-annotationtemplates Show documentation
Provides a code generator for Annotation Templates. Annotation Templates provide an easy way to generate annotations.
package de.japkit.annotationtemplates;
import java.lang.annotation.Annotation;
import javax.validation.Constraint;
import javax.validation.ConstraintValidator;
import javax.validation.GroupSequence;
import javax.validation.OverridesAttribute;
import javax.validation.Payload;
import javax.validation.ReportAsSingleViolation;
import javax.validation.Valid;
import javax.validation.constraints.AssertFalse;
import javax.validation.constraints.AssertTrue;
import javax.validation.constraints.DecimalMax;
import javax.validation.constraints.DecimalMin;
import javax.validation.constraints.Digits;
import javax.validation.constraints.Future;
import javax.validation.constraints.Max;
import javax.validation.constraints.Min;
import javax.validation.constraints.NotNull;
import javax.validation.constraints.Null;
import javax.validation.constraints.Past;
import javax.validation.constraints.Pattern;
import javax.validation.constraints.Pattern.Flag;
import javax.validation.constraints.Size;
import de.japkit.annotations.AnnotationTemplate;
import de.japkit.annotations.Generated;
import de.japkit.annotations.Order;
import de.japkit.annotationtemplates.AnnotationTemplates;
@AnnotationTemplates(targetAnnotations = {Constraint.class,
GroupSequence.class,
OverridesAttribute.class,
ReportAsSingleViolation.class,
Valid.class,
AssertFalse.class,
AssertTrue.class,
DecimalMax.class,
DecimalMin.class,
Digits.class,
Future.class,
Max.class,
Min.class,
NotNull.class,
Null.class,
Past.class,
Pattern.class,
Size.class},
shadow = true)
@Generated(src = "de.japkit.annotationtemplates.BeanValidationAnnotationTemplates")
public class BeanValidationAnnotationTemplatesGen {
/** This annotation template will generate an annotation of type {@link javax.validation.Constraint}. */
@AnnotationTemplate(targetAnnotation = Constraint.class)
@Order(0)
public static @interface Constraint_ {
/** @return a constant value for annotation value 'validatedBy'.
@see javax.validation.Constraint#value() */
@Order(0)
public Class extends ConstraintValidator, ?>> [] validatedBy() default {};
/** @return expression to determine if the annotation value 'validatedBy' shall be generated. Default is true. */
@Order(1)
public String validatedBy_cond() default "";
/** @return the expression language for the condition expression for 'validatedBy'. */
@Order(2)
public String validatedBy_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'validatedBy' shall be generated. */
@Order(3)
public Class> [] validatedBy_condFun() default {};
/** @return the src expression for generating annotation value 'validatedBy'. If this results in a collection, an array will be generated. */
@Order(4)
public String validatedBy_src() default "";
/** @return the expression for the value of annotation value 'validatedBy'.
Can be used as alternative to {@link validatedBy()} if the value is not constant. */
@Order(5)
public String validatedBy_expr() default "";
/** An expression to determine the source object for generating this annotation(s).
The source element is available as "src" in expressions and is used in
matchers and other rules. If the src expression is not set, the src
element of the element hosting the annotation is used.
@return */
@Order(6)
public String _src() default "";
/** @return the language of the src expression. Defaults to Java EL. */
@Order(7)
public String _srcLang() default "";
/** As an alternative to the src expression, a function can be called to determine the source object.
@return */
@Order(8)
public Class> [] _srcFun() default {};
/** @return expression to determine if the annotation shall be generated. Default is true. */
@Order(9)
public String _cond() default "";
/** @return the expression language for the condition expression. */
@Order(10)
public String _condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called. */
@Order(11)
public Class> [] _condFun() default {};
}
/** This annotation template will generate an annotation of type {@link javax.validation.GroupSequence}. */
@AnnotationTemplate(targetAnnotation = GroupSequence.class)
@Order(1)
public static @interface GroupSequence_ {
/** @return a constant value for annotation value 'value'.
@see javax.validation.GroupSequence#value() */
@Order(0)
public Class> [] value() default {};
/** @return expression to determine if the annotation value 'value' shall be generated. Default is true. */
@Order(1)
public String value_cond() default "";
/** @return the expression language for the condition expression for 'value'. */
@Order(2)
public String value_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'value' shall be generated. */
@Order(3)
public Class> [] value_condFun() default {};
/** @return the src expression for generating annotation value 'value'. If this results in a collection, an array will be generated. */
@Order(4)
public String value_src() default "";
/** @return the expression for the value of annotation value 'value'.
Can be used as alternative to {@link value()} if the value is not constant. */
@Order(5)
public String value_expr() default "";
/** An expression to determine the source object for generating this annotation(s).
The source element is available as "src" in expressions and is used in
matchers and other rules. If the src expression is not set, the src
element of the element hosting the annotation is used.
@return */
@Order(6)
public String _src() default "";
/** @return the language of the src expression. Defaults to Java EL. */
@Order(7)
public String _srcLang() default "";
/** As an alternative to the src expression, a function can be called to determine the source object.
@return */
@Order(8)
public Class> [] _srcFun() default {};
/** @return expression to determine if the annotation shall be generated. Default is true. */
@Order(9)
public String _cond() default "";
/** @return the expression language for the condition expression. */
@Order(10)
public String _condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called. */
@Order(11)
public Class> [] _condFun() default {};
}
/** This annotation template will generate an annotation of type {@link javax.validation.OverridesAttribute}. */
@AnnotationTemplate(targetAnnotation = OverridesAttribute.class)
@Order(2)
public static @interface OverridesAttribute_ {
/** @return a constant value for annotation value 'constraint'.
@see javax.validation.OverridesAttribute#value() */
@Order(0)
public Class extends Annotation> [] constraint() default {};
/** @return expression to determine if the annotation value 'constraint' shall be generated. Default is true. */
@Order(1)
public String constraint_cond() default "";
/** @return the expression language for the condition expression for 'constraint'. */
@Order(2)
public String constraint_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'constraint' shall be generated. */
@Order(3)
public Class> [] constraint_condFun() default {};
/** @return the src expression for generating annotation value 'constraint'. If this results in a collection, an array will be generated. */
@Order(4)
public String constraint_src() default "";
/** @return the expression for the value of annotation value 'constraint'.
Can be used as alternative to {@link constraint()} if the value is not constant. */
@Order(5)
public String constraint_expr() default "";
/** @return a constant value for annotation value 'name'.
@see javax.validation.OverridesAttribute#value() */
@Order(6)
public String [] name() default {};
/** @return expression to determine if the annotation value 'name' shall be generated. Default is true. */
@Order(7)
public String name_cond() default "";
/** @return the expression language for the condition expression for 'name'. */
@Order(8)
public String name_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'name' shall be generated. */
@Order(9)
public Class> [] name_condFun() default {};
/** @return the src expression for generating annotation value 'name'. If this results in a collection, an array will be generated. */
@Order(10)
public String name_src() default "";
/** @return the expression for the value of annotation value 'name'.
Can be used as alternative to {@link name()} if the value is not constant. */
@Order(11)
public String name_expr() default "";
/** @return a constant value for annotation value 'constraintIndex'.
@see javax.validation.OverridesAttribute#value() */
@Order(12)
public int [] constraintIndex() default {};
/** @return expression to determine if the annotation value 'constraintIndex' shall be generated. Default is true. */
@Order(13)
public String constraintIndex_cond() default "";
/** @return the expression language for the condition expression for 'constraintIndex'. */
@Order(14)
public String constraintIndex_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'constraintIndex' shall be generated. */
@Order(15)
public Class> [] constraintIndex_condFun() default {};
/** @return the src expression for generating annotation value 'constraintIndex'. If this results in a collection, an array will be generated. */
@Order(16)
public String constraintIndex_src() default "";
/** @return the expression for the value of annotation value 'constraintIndex'.
Can be used as alternative to {@link constraintIndex()} if the value is not constant. */
@Order(17)
public String constraintIndex_expr() default "";
/** An expression to determine the source object for generating this annotation(s).
The source element is available as "src" in expressions and is used in
matchers and other rules. If the src expression is not set, the src
element of the element hosting the annotation is used.
@return */
@Order(18)
public String _src() default "";
/** @return the language of the src expression. Defaults to Java EL. */
@Order(19)
public String _srcLang() default "";
/** As an alternative to the src expression, a function can be called to determine the source object.
@return */
@Order(20)
public Class> [] _srcFun() default {};
/** @return expression to determine if the annotation shall be generated. Default is true. */
@Order(21)
public String _cond() default "";
/** @return the expression language for the condition expression. */
@Order(22)
public String _condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called. */
@Order(23)
public Class> [] _condFun() default {};
}
/** This annotation template will generate an annotation of type {@link javax.validation.ReportAsSingleViolation}. */
@AnnotationTemplate(targetAnnotation = ReportAsSingleViolation.class)
@Order(3)
public static @interface ReportAsSingleViolation_ {
/** An expression to determine the source object for generating this annotation(s).
The source element is available as "src" in expressions and is used in
matchers and other rules. If the src expression is not set, the src
element of the element hosting the annotation is used.
@return */
@Order(0)
public String _src() default "";
/** @return the language of the src expression. Defaults to Java EL. */
@Order(1)
public String _srcLang() default "";
/** As an alternative to the src expression, a function can be called to determine the source object.
@return */
@Order(2)
public Class> [] _srcFun() default {};
/** @return expression to determine if the annotation shall be generated. Default is true. */
@Order(3)
public String _cond() default "";
/** @return the expression language for the condition expression. */
@Order(4)
public String _condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called. */
@Order(5)
public Class> [] _condFun() default {};
}
/** This annotation template will generate an annotation of type {@link javax.validation.Valid}. */
@AnnotationTemplate(targetAnnotation = Valid.class)
@Order(4)
public static @interface Valid_ {
/** An expression to determine the source object for generating this annotation(s).
The source element is available as "src" in expressions and is used in
matchers and other rules. If the src expression is not set, the src
element of the element hosting the annotation is used.
@return */
@Order(0)
public String _src() default "";
/** @return the language of the src expression. Defaults to Java EL. */
@Order(1)
public String _srcLang() default "";
/** As an alternative to the src expression, a function can be called to determine the source object.
@return */
@Order(2)
public Class> [] _srcFun() default {};
/** @return expression to determine if the annotation shall be generated. Default is true. */
@Order(3)
public String _cond() default "";
/** @return the expression language for the condition expression. */
@Order(4)
public String _condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called. */
@Order(5)
public Class> [] _condFun() default {};
}
/** This annotation template will generate an annotation of type {@link javax.validation.constraints.AssertFalse}. */
@AnnotationTemplate(targetAnnotation = AssertFalse.class)
@Order(5)
public static @interface AssertFalse_ {
/** @return a constant value for annotation value 'message'.
@see javax.validation.constraints.AssertFalse#value() */
@Order(0)
public String [] message() default {};
/** @return expression to determine if the annotation value 'message' shall be generated. Default is true. */
@Order(1)
public String message_cond() default "";
/** @return the expression language for the condition expression for 'message'. */
@Order(2)
public String message_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'message' shall be generated. */
@Order(3)
public Class> [] message_condFun() default {};
/** @return the src expression for generating annotation value 'message'. If this results in a collection, an array will be generated. */
@Order(4)
public String message_src() default "";
/** @return the expression for the value of annotation value 'message'.
Can be used as alternative to {@link message()} if the value is not constant. */
@Order(5)
public String message_expr() default "";
/** @return a constant value for annotation value 'groups'.
@see javax.validation.constraints.AssertFalse#value() */
@Order(6)
public Class> [] groups() default {};
/** @return expression to determine if the annotation value 'groups' shall be generated. Default is true. */
@Order(7)
public String groups_cond() default "";
/** @return the expression language for the condition expression for 'groups'. */
@Order(8)
public String groups_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'groups' shall be generated. */
@Order(9)
public Class> [] groups_condFun() default {};
/** @return the src expression for generating annotation value 'groups'. If this results in a collection, an array will be generated. */
@Order(10)
public String groups_src() default "";
/** @return the expression for the value of annotation value 'groups'.
Can be used as alternative to {@link groups()} if the value is not constant. */
@Order(11)
public String groups_expr() default "";
/** @return a constant value for annotation value 'payload'.
@see javax.validation.constraints.AssertFalse#value() */
@Order(12)
public Class extends Payload> [] payload() default {};
/** @return expression to determine if the annotation value 'payload' shall be generated. Default is true. */
@Order(13)
public String payload_cond() default "";
/** @return the expression language for the condition expression for 'payload'. */
@Order(14)
public String payload_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'payload' shall be generated. */
@Order(15)
public Class> [] payload_condFun() default {};
/** @return the src expression for generating annotation value 'payload'. If this results in a collection, an array will be generated. */
@Order(16)
public String payload_src() default "";
/** @return the expression for the value of annotation value 'payload'.
Can be used as alternative to {@link payload()} if the value is not constant. */
@Order(17)
public String payload_expr() default "";
/** An expression to determine the source object for generating this annotation(s).
The source element is available as "src" in expressions and is used in
matchers and other rules. If the src expression is not set, the src
element of the element hosting the annotation is used.
@return */
@Order(18)
public String _src() default "";
/** @return the language of the src expression. Defaults to Java EL. */
@Order(19)
public String _srcLang() default "";
/** As an alternative to the src expression, a function can be called to determine the source object.
@return */
@Order(20)
public Class> [] _srcFun() default {};
/** @return expression to determine if the annotation shall be generated. Default is true. */
@Order(21)
public String _cond() default "";
/** @return the expression language for the condition expression. */
@Order(22)
public String _condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called. */
@Order(23)
public Class> [] _condFun() default {};
}
/** This annotation template will generate an annotation of type {@link javax.validation.constraints.AssertTrue}. */
@AnnotationTemplate(targetAnnotation = AssertTrue.class)
@Order(6)
public static @interface AssertTrue_ {
/** @return a constant value for annotation value 'message'.
@see javax.validation.constraints.AssertTrue#value() */
@Order(0)
public String [] message() default {};
/** @return expression to determine if the annotation value 'message' shall be generated. Default is true. */
@Order(1)
public String message_cond() default "";
/** @return the expression language for the condition expression for 'message'. */
@Order(2)
public String message_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'message' shall be generated. */
@Order(3)
public Class> [] message_condFun() default {};
/** @return the src expression for generating annotation value 'message'. If this results in a collection, an array will be generated. */
@Order(4)
public String message_src() default "";
/** @return the expression for the value of annotation value 'message'.
Can be used as alternative to {@link message()} if the value is not constant. */
@Order(5)
public String message_expr() default "";
/** @return a constant value for annotation value 'groups'.
@see javax.validation.constraints.AssertTrue#value() */
@Order(6)
public Class> [] groups() default {};
/** @return expression to determine if the annotation value 'groups' shall be generated. Default is true. */
@Order(7)
public String groups_cond() default "";
/** @return the expression language for the condition expression for 'groups'. */
@Order(8)
public String groups_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'groups' shall be generated. */
@Order(9)
public Class> [] groups_condFun() default {};
/** @return the src expression for generating annotation value 'groups'. If this results in a collection, an array will be generated. */
@Order(10)
public String groups_src() default "";
/** @return the expression for the value of annotation value 'groups'.
Can be used as alternative to {@link groups()} if the value is not constant. */
@Order(11)
public String groups_expr() default "";
/** @return a constant value for annotation value 'payload'.
@see javax.validation.constraints.AssertTrue#value() */
@Order(12)
public Class extends Payload> [] payload() default {};
/** @return expression to determine if the annotation value 'payload' shall be generated. Default is true. */
@Order(13)
public String payload_cond() default "";
/** @return the expression language for the condition expression for 'payload'. */
@Order(14)
public String payload_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'payload' shall be generated. */
@Order(15)
public Class> [] payload_condFun() default {};
/** @return the src expression for generating annotation value 'payload'. If this results in a collection, an array will be generated. */
@Order(16)
public String payload_src() default "";
/** @return the expression for the value of annotation value 'payload'.
Can be used as alternative to {@link payload()} if the value is not constant. */
@Order(17)
public String payload_expr() default "";
/** An expression to determine the source object for generating this annotation(s).
The source element is available as "src" in expressions and is used in
matchers and other rules. If the src expression is not set, the src
element of the element hosting the annotation is used.
@return */
@Order(18)
public String _src() default "";
/** @return the language of the src expression. Defaults to Java EL. */
@Order(19)
public String _srcLang() default "";
/** As an alternative to the src expression, a function can be called to determine the source object.
@return */
@Order(20)
public Class> [] _srcFun() default {};
/** @return expression to determine if the annotation shall be generated. Default is true. */
@Order(21)
public String _cond() default "";
/** @return the expression language for the condition expression. */
@Order(22)
public String _condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called. */
@Order(23)
public Class> [] _condFun() default {};
}
/** This annotation template will generate an annotation of type {@link javax.validation.constraints.DecimalMax}. */
@AnnotationTemplate(targetAnnotation = DecimalMax.class)
@Order(7)
public static @interface DecimalMax_ {
/** @return a constant value for annotation value 'message'.
@see javax.validation.constraints.DecimalMax#value() */
@Order(0)
public String [] message() default {};
/** @return expression to determine if the annotation value 'message' shall be generated. Default is true. */
@Order(1)
public String message_cond() default "";
/** @return the expression language for the condition expression for 'message'. */
@Order(2)
public String message_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'message' shall be generated. */
@Order(3)
public Class> [] message_condFun() default {};
/** @return the src expression for generating annotation value 'message'. If this results in a collection, an array will be generated. */
@Order(4)
public String message_src() default "";
/** @return the expression for the value of annotation value 'message'.
Can be used as alternative to {@link message()} if the value is not constant. */
@Order(5)
public String message_expr() default "";
/** @return a constant value for annotation value 'groups'.
@see javax.validation.constraints.DecimalMax#value() */
@Order(6)
public Class> [] groups() default {};
/** @return expression to determine if the annotation value 'groups' shall be generated. Default is true. */
@Order(7)
public String groups_cond() default "";
/** @return the expression language for the condition expression for 'groups'. */
@Order(8)
public String groups_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'groups' shall be generated. */
@Order(9)
public Class> [] groups_condFun() default {};
/** @return the src expression for generating annotation value 'groups'. If this results in a collection, an array will be generated. */
@Order(10)
public String groups_src() default "";
/** @return the expression for the value of annotation value 'groups'.
Can be used as alternative to {@link groups()} if the value is not constant. */
@Order(11)
public String groups_expr() default "";
/** @return a constant value for annotation value 'payload'.
@see javax.validation.constraints.DecimalMax#value() */
@Order(12)
public Class extends Payload> [] payload() default {};
/** @return expression to determine if the annotation value 'payload' shall be generated. Default is true. */
@Order(13)
public String payload_cond() default "";
/** @return the expression language for the condition expression for 'payload'. */
@Order(14)
public String payload_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'payload' shall be generated. */
@Order(15)
public Class> [] payload_condFun() default {};
/** @return the src expression for generating annotation value 'payload'. If this results in a collection, an array will be generated. */
@Order(16)
public String payload_src() default "";
/** @return the expression for the value of annotation value 'payload'.
Can be used as alternative to {@link payload()} if the value is not constant. */
@Order(17)
public String payload_expr() default "";
/** @return a constant value for annotation value 'value'.
@see javax.validation.constraints.DecimalMax#value() */
@Order(18)
public String [] value() default {};
/** @return expression to determine if the annotation value 'value' shall be generated. Default is true. */
@Order(19)
public String value_cond() default "";
/** @return the expression language for the condition expression for 'value'. */
@Order(20)
public String value_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'value' shall be generated. */
@Order(21)
public Class> [] value_condFun() default {};
/** @return the src expression for generating annotation value 'value'. If this results in a collection, an array will be generated. */
@Order(22)
public String value_src() default "";
/** @return the expression for the value of annotation value 'value'.
Can be used as alternative to {@link value()} if the value is not constant. */
@Order(23)
public String value_expr() default "";
/** @return a constant value for annotation value 'inclusive'.
@see javax.validation.constraints.DecimalMax#value() */
@Order(24)
public boolean [] inclusive() default {};
/** @return expression to determine if the annotation value 'inclusive' shall be generated. Default is true. */
@Order(25)
public String inclusive_cond() default "";
/** @return the expression language for the condition expression for 'inclusive'. */
@Order(26)
public String inclusive_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'inclusive' shall be generated. */
@Order(27)
public Class> [] inclusive_condFun() default {};
/** @return the src expression for generating annotation value 'inclusive'. If this results in a collection, an array will be generated. */
@Order(28)
public String inclusive_src() default "";
/** @return the expression for the value of annotation value 'inclusive'.
Can be used as alternative to {@link inclusive()} if the value is not constant. */
@Order(29)
public String inclusive_expr() default "";
/** An expression to determine the source object for generating this annotation(s).
The source element is available as "src" in expressions and is used in
matchers and other rules. If the src expression is not set, the src
element of the element hosting the annotation is used.
@return */
@Order(30)
public String _src() default "";
/** @return the language of the src expression. Defaults to Java EL. */
@Order(31)
public String _srcLang() default "";
/** As an alternative to the src expression, a function can be called to determine the source object.
@return */
@Order(32)
public Class> [] _srcFun() default {};
/** @return expression to determine if the annotation shall be generated. Default is true. */
@Order(33)
public String _cond() default "";
/** @return the expression language for the condition expression. */
@Order(34)
public String _condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called. */
@Order(35)
public Class> [] _condFun() default {};
}
/** This annotation template will generate an annotation of type {@link javax.validation.constraints.DecimalMin}. */
@AnnotationTemplate(targetAnnotation = DecimalMin.class)
@Order(8)
public static @interface DecimalMin_ {
/** @return a constant value for annotation value 'message'.
@see javax.validation.constraints.DecimalMin#value() */
@Order(0)
public String [] message() default {};
/** @return expression to determine if the annotation value 'message' shall be generated. Default is true. */
@Order(1)
public String message_cond() default "";
/** @return the expression language for the condition expression for 'message'. */
@Order(2)
public String message_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'message' shall be generated. */
@Order(3)
public Class> [] message_condFun() default {};
/** @return the src expression for generating annotation value 'message'. If this results in a collection, an array will be generated. */
@Order(4)
public String message_src() default "";
/** @return the expression for the value of annotation value 'message'.
Can be used as alternative to {@link message()} if the value is not constant. */
@Order(5)
public String message_expr() default "";
/** @return a constant value for annotation value 'groups'.
@see javax.validation.constraints.DecimalMin#value() */
@Order(6)
public Class> [] groups() default {};
/** @return expression to determine if the annotation value 'groups' shall be generated. Default is true. */
@Order(7)
public String groups_cond() default "";
/** @return the expression language for the condition expression for 'groups'. */
@Order(8)
public String groups_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'groups' shall be generated. */
@Order(9)
public Class> [] groups_condFun() default {};
/** @return the src expression for generating annotation value 'groups'. If this results in a collection, an array will be generated. */
@Order(10)
public String groups_src() default "";
/** @return the expression for the value of annotation value 'groups'.
Can be used as alternative to {@link groups()} if the value is not constant. */
@Order(11)
public String groups_expr() default "";
/** @return a constant value for annotation value 'payload'.
@see javax.validation.constraints.DecimalMin#value() */
@Order(12)
public Class extends Payload> [] payload() default {};
/** @return expression to determine if the annotation value 'payload' shall be generated. Default is true. */
@Order(13)
public String payload_cond() default "";
/** @return the expression language for the condition expression for 'payload'. */
@Order(14)
public String payload_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'payload' shall be generated. */
@Order(15)
public Class> [] payload_condFun() default {};
/** @return the src expression for generating annotation value 'payload'. If this results in a collection, an array will be generated. */
@Order(16)
public String payload_src() default "";
/** @return the expression for the value of annotation value 'payload'.
Can be used as alternative to {@link payload()} if the value is not constant. */
@Order(17)
public String payload_expr() default "";
/** @return a constant value for annotation value 'value'.
@see javax.validation.constraints.DecimalMin#value() */
@Order(18)
public String [] value() default {};
/** @return expression to determine if the annotation value 'value' shall be generated. Default is true. */
@Order(19)
public String value_cond() default "";
/** @return the expression language for the condition expression for 'value'. */
@Order(20)
public String value_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'value' shall be generated. */
@Order(21)
public Class> [] value_condFun() default {};
/** @return the src expression for generating annotation value 'value'. If this results in a collection, an array will be generated. */
@Order(22)
public String value_src() default "";
/** @return the expression for the value of annotation value 'value'.
Can be used as alternative to {@link value()} if the value is not constant. */
@Order(23)
public String value_expr() default "";
/** @return a constant value for annotation value 'inclusive'.
@see javax.validation.constraints.DecimalMin#value() */
@Order(24)
public boolean [] inclusive() default {};
/** @return expression to determine if the annotation value 'inclusive' shall be generated. Default is true. */
@Order(25)
public String inclusive_cond() default "";
/** @return the expression language for the condition expression for 'inclusive'. */
@Order(26)
public String inclusive_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'inclusive' shall be generated. */
@Order(27)
public Class> [] inclusive_condFun() default {};
/** @return the src expression for generating annotation value 'inclusive'. If this results in a collection, an array will be generated. */
@Order(28)
public String inclusive_src() default "";
/** @return the expression for the value of annotation value 'inclusive'.
Can be used as alternative to {@link inclusive()} if the value is not constant. */
@Order(29)
public String inclusive_expr() default "";
/** An expression to determine the source object for generating this annotation(s).
The source element is available as "src" in expressions and is used in
matchers and other rules. If the src expression is not set, the src
element of the element hosting the annotation is used.
@return */
@Order(30)
public String _src() default "";
/** @return the language of the src expression. Defaults to Java EL. */
@Order(31)
public String _srcLang() default "";
/** As an alternative to the src expression, a function can be called to determine the source object.
@return */
@Order(32)
public Class> [] _srcFun() default {};
/** @return expression to determine if the annotation shall be generated. Default is true. */
@Order(33)
public String _cond() default "";
/** @return the expression language for the condition expression. */
@Order(34)
public String _condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called. */
@Order(35)
public Class> [] _condFun() default {};
}
/** This annotation template will generate an annotation of type {@link javax.validation.constraints.Digits}. */
@AnnotationTemplate(targetAnnotation = Digits.class)
@Order(9)
public static @interface Digits_ {
/** @return a constant value for annotation value 'message'.
@see javax.validation.constraints.Digits#value() */
@Order(0)
public String [] message() default {};
/** @return expression to determine if the annotation value 'message' shall be generated. Default is true. */
@Order(1)
public String message_cond() default "";
/** @return the expression language for the condition expression for 'message'. */
@Order(2)
public String message_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'message' shall be generated. */
@Order(3)
public Class> [] message_condFun() default {};
/** @return the src expression for generating annotation value 'message'. If this results in a collection, an array will be generated. */
@Order(4)
public String message_src() default "";
/** @return the expression for the value of annotation value 'message'.
Can be used as alternative to {@link message()} if the value is not constant. */
@Order(5)
public String message_expr() default "";
/** @return a constant value for annotation value 'groups'.
@see javax.validation.constraints.Digits#value() */
@Order(6)
public Class> [] groups() default {};
/** @return expression to determine if the annotation value 'groups' shall be generated. Default is true. */
@Order(7)
public String groups_cond() default "";
/** @return the expression language for the condition expression for 'groups'. */
@Order(8)
public String groups_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'groups' shall be generated. */
@Order(9)
public Class> [] groups_condFun() default {};
/** @return the src expression for generating annotation value 'groups'. If this results in a collection, an array will be generated. */
@Order(10)
public String groups_src() default "";
/** @return the expression for the value of annotation value 'groups'.
Can be used as alternative to {@link groups()} if the value is not constant. */
@Order(11)
public String groups_expr() default "";
/** @return a constant value for annotation value 'payload'.
@see javax.validation.constraints.Digits#value() */
@Order(12)
public Class extends Payload> [] payload() default {};
/** @return expression to determine if the annotation value 'payload' shall be generated. Default is true. */
@Order(13)
public String payload_cond() default "";
/** @return the expression language for the condition expression for 'payload'. */
@Order(14)
public String payload_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'payload' shall be generated. */
@Order(15)
public Class> [] payload_condFun() default {};
/** @return the src expression for generating annotation value 'payload'. If this results in a collection, an array will be generated. */
@Order(16)
public String payload_src() default "";
/** @return the expression for the value of annotation value 'payload'.
Can be used as alternative to {@link payload()} if the value is not constant. */
@Order(17)
public String payload_expr() default "";
/** @return a constant value for annotation value 'integer'.
@see javax.validation.constraints.Digits#value() */
@Order(18)
public int [] integer() default {};
/** @return expression to determine if the annotation value 'integer' shall be generated. Default is true. */
@Order(19)
public String integer_cond() default "";
/** @return the expression language for the condition expression for 'integer'. */
@Order(20)
public String integer_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'integer' shall be generated. */
@Order(21)
public Class> [] integer_condFun() default {};
/** @return the src expression for generating annotation value 'integer'. If this results in a collection, an array will be generated. */
@Order(22)
public String integer_src() default "";
/** @return the expression for the value of annotation value 'integer'.
Can be used as alternative to {@link integer()} if the value is not constant. */
@Order(23)
public String integer_expr() default "";
/** @return a constant value for annotation value 'fraction'.
@see javax.validation.constraints.Digits#value() */
@Order(24)
public int [] fraction() default {};
/** @return expression to determine if the annotation value 'fraction' shall be generated. Default is true. */
@Order(25)
public String fraction_cond() default "";
/** @return the expression language for the condition expression for 'fraction'. */
@Order(26)
public String fraction_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'fraction' shall be generated. */
@Order(27)
public Class> [] fraction_condFun() default {};
/** @return the src expression for generating annotation value 'fraction'. If this results in a collection, an array will be generated. */
@Order(28)
public String fraction_src() default "";
/** @return the expression for the value of annotation value 'fraction'.
Can be used as alternative to {@link fraction()} if the value is not constant. */
@Order(29)
public String fraction_expr() default "";
/** An expression to determine the source object for generating this annotation(s).
The source element is available as "src" in expressions and is used in
matchers and other rules. If the src expression is not set, the src
element of the element hosting the annotation is used.
@return */
@Order(30)
public String _src() default "";
/** @return the language of the src expression. Defaults to Java EL. */
@Order(31)
public String _srcLang() default "";
/** As an alternative to the src expression, a function can be called to determine the source object.
@return */
@Order(32)
public Class> [] _srcFun() default {};
/** @return expression to determine if the annotation shall be generated. Default is true. */
@Order(33)
public String _cond() default "";
/** @return the expression language for the condition expression. */
@Order(34)
public String _condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called. */
@Order(35)
public Class> [] _condFun() default {};
}
/** This annotation template will generate an annotation of type {@link javax.validation.constraints.Future}. */
@AnnotationTemplate(targetAnnotation = Future.class)
@Order(10)
public static @interface Future_ {
/** @return a constant value for annotation value 'message'.
@see javax.validation.constraints.Future#value() */
@Order(0)
public String [] message() default {};
/** @return expression to determine if the annotation value 'message' shall be generated. Default is true. */
@Order(1)
public String message_cond() default "";
/** @return the expression language for the condition expression for 'message'. */
@Order(2)
public String message_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'message' shall be generated. */
@Order(3)
public Class> [] message_condFun() default {};
/** @return the src expression for generating annotation value 'message'. If this results in a collection, an array will be generated. */
@Order(4)
public String message_src() default "";
/** @return the expression for the value of annotation value 'message'.
Can be used as alternative to {@link message()} if the value is not constant. */
@Order(5)
public String message_expr() default "";
/** @return a constant value for annotation value 'groups'.
@see javax.validation.constraints.Future#value() */
@Order(6)
public Class> [] groups() default {};
/** @return expression to determine if the annotation value 'groups' shall be generated. Default is true. */
@Order(7)
public String groups_cond() default "";
/** @return the expression language for the condition expression for 'groups'. */
@Order(8)
public String groups_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'groups' shall be generated. */
@Order(9)
public Class> [] groups_condFun() default {};
/** @return the src expression for generating annotation value 'groups'. If this results in a collection, an array will be generated. */
@Order(10)
public String groups_src() default "";
/** @return the expression for the value of annotation value 'groups'.
Can be used as alternative to {@link groups()} if the value is not constant. */
@Order(11)
public String groups_expr() default "";
/** @return a constant value for annotation value 'payload'.
@see javax.validation.constraints.Future#value() */
@Order(12)
public Class extends Payload> [] payload() default {};
/** @return expression to determine if the annotation value 'payload' shall be generated. Default is true. */
@Order(13)
public String payload_cond() default "";
/** @return the expression language for the condition expression for 'payload'. */
@Order(14)
public String payload_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'payload' shall be generated. */
@Order(15)
public Class> [] payload_condFun() default {};
/** @return the src expression for generating annotation value 'payload'. If this results in a collection, an array will be generated. */
@Order(16)
public String payload_src() default "";
/** @return the expression for the value of annotation value 'payload'.
Can be used as alternative to {@link payload()} if the value is not constant. */
@Order(17)
public String payload_expr() default "";
/** An expression to determine the source object for generating this annotation(s).
The source element is available as "src" in expressions and is used in
matchers and other rules. If the src expression is not set, the src
element of the element hosting the annotation is used.
@return */
@Order(18)
public String _src() default "";
/** @return the language of the src expression. Defaults to Java EL. */
@Order(19)
public String _srcLang() default "";
/** As an alternative to the src expression, a function can be called to determine the source object.
@return */
@Order(20)
public Class> [] _srcFun() default {};
/** @return expression to determine if the annotation shall be generated. Default is true. */
@Order(21)
public String _cond() default "";
/** @return the expression language for the condition expression. */
@Order(22)
public String _condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called. */
@Order(23)
public Class> [] _condFun() default {};
}
/** This annotation template will generate an annotation of type {@link javax.validation.constraints.Max}. */
@AnnotationTemplate(targetAnnotation = Max.class)
@Order(11)
public static @interface Max_ {
/** @return a constant value for annotation value 'message'.
@see javax.validation.constraints.Max#value() */
@Order(0)
public String [] message() default {};
/** @return expression to determine if the annotation value 'message' shall be generated. Default is true. */
@Order(1)
public String message_cond() default "";
/** @return the expression language for the condition expression for 'message'. */
@Order(2)
public String message_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'message' shall be generated. */
@Order(3)
public Class> [] message_condFun() default {};
/** @return the src expression for generating annotation value 'message'. If this results in a collection, an array will be generated. */
@Order(4)
public String message_src() default "";
/** @return the expression for the value of annotation value 'message'.
Can be used as alternative to {@link message()} if the value is not constant. */
@Order(5)
public String message_expr() default "";
/** @return a constant value for annotation value 'groups'.
@see javax.validation.constraints.Max#value() */
@Order(6)
public Class> [] groups() default {};
/** @return expression to determine if the annotation value 'groups' shall be generated. Default is true. */
@Order(7)
public String groups_cond() default "";
/** @return the expression language for the condition expression for 'groups'. */
@Order(8)
public String groups_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'groups' shall be generated. */
@Order(9)
public Class> [] groups_condFun() default {};
/** @return the src expression for generating annotation value 'groups'. If this results in a collection, an array will be generated. */
@Order(10)
public String groups_src() default "";
/** @return the expression for the value of annotation value 'groups'.
Can be used as alternative to {@link groups()} if the value is not constant. */
@Order(11)
public String groups_expr() default "";
/** @return a constant value for annotation value 'payload'.
@see javax.validation.constraints.Max#value() */
@Order(12)
public Class extends Payload> [] payload() default {};
/** @return expression to determine if the annotation value 'payload' shall be generated. Default is true. */
@Order(13)
public String payload_cond() default "";
/** @return the expression language for the condition expression for 'payload'. */
@Order(14)
public String payload_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'payload' shall be generated. */
@Order(15)
public Class> [] payload_condFun() default {};
/** @return the src expression for generating annotation value 'payload'. If this results in a collection, an array will be generated. */
@Order(16)
public String payload_src() default "";
/** @return the expression for the value of annotation value 'payload'.
Can be used as alternative to {@link payload()} if the value is not constant. */
@Order(17)
public String payload_expr() default "";
/** @return a constant value for annotation value 'value'.
@see javax.validation.constraints.Max#value() */
@Order(18)
public long [] value() default {};
/** @return expression to determine if the annotation value 'value' shall be generated. Default is true. */
@Order(19)
public String value_cond() default "";
/** @return the expression language for the condition expression for 'value'. */
@Order(20)
public String value_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'value' shall be generated. */
@Order(21)
public Class> [] value_condFun() default {};
/** @return the src expression for generating annotation value 'value'. If this results in a collection, an array will be generated. */
@Order(22)
public String value_src() default "";
/** @return the expression for the value of annotation value 'value'.
Can be used as alternative to {@link value()} if the value is not constant. */
@Order(23)
public String value_expr() default "";
/** An expression to determine the source object for generating this annotation(s).
The source element is available as "src" in expressions and is used in
matchers and other rules. If the src expression is not set, the src
element of the element hosting the annotation is used.
@return */
@Order(24)
public String _src() default "";
/** @return the language of the src expression. Defaults to Java EL. */
@Order(25)
public String _srcLang() default "";
/** As an alternative to the src expression, a function can be called to determine the source object.
@return */
@Order(26)
public Class> [] _srcFun() default {};
/** @return expression to determine if the annotation shall be generated. Default is true. */
@Order(27)
public String _cond() default "";
/** @return the expression language for the condition expression. */
@Order(28)
public String _condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called. */
@Order(29)
public Class> [] _condFun() default {};
}
/** This annotation template will generate an annotation of type {@link javax.validation.constraints.Min}. */
@AnnotationTemplate(targetAnnotation = Min.class)
@Order(12)
public static @interface Min_ {
/** @return a constant value for annotation value 'message'.
@see javax.validation.constraints.Min#value() */
@Order(0)
public String [] message() default {};
/** @return expression to determine if the annotation value 'message' shall be generated. Default is true. */
@Order(1)
public String message_cond() default "";
/** @return the expression language for the condition expression for 'message'. */
@Order(2)
public String message_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'message' shall be generated. */
@Order(3)
public Class> [] message_condFun() default {};
/** @return the src expression for generating annotation value 'message'. If this results in a collection, an array will be generated. */
@Order(4)
public String message_src() default "";
/** @return the expression for the value of annotation value 'message'.
Can be used as alternative to {@link message()} if the value is not constant. */
@Order(5)
public String message_expr() default "";
/** @return a constant value for annotation value 'groups'.
@see javax.validation.constraints.Min#value() */
@Order(6)
public Class> [] groups() default {};
/** @return expression to determine if the annotation value 'groups' shall be generated. Default is true. */
@Order(7)
public String groups_cond() default "";
/** @return the expression language for the condition expression for 'groups'. */
@Order(8)
public String groups_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'groups' shall be generated. */
@Order(9)
public Class> [] groups_condFun() default {};
/** @return the src expression for generating annotation value 'groups'. If this results in a collection, an array will be generated. */
@Order(10)
public String groups_src() default "";
/** @return the expression for the value of annotation value 'groups'.
Can be used as alternative to {@link groups()} if the value is not constant. */
@Order(11)
public String groups_expr() default "";
/** @return a constant value for annotation value 'payload'.
@see javax.validation.constraints.Min#value() */
@Order(12)
public Class extends Payload> [] payload() default {};
/** @return expression to determine if the annotation value 'payload' shall be generated. Default is true. */
@Order(13)
public String payload_cond() default "";
/** @return the expression language for the condition expression for 'payload'. */
@Order(14)
public String payload_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'payload' shall be generated. */
@Order(15)
public Class> [] payload_condFun() default {};
/** @return the src expression for generating annotation value 'payload'. If this results in a collection, an array will be generated. */
@Order(16)
public String payload_src() default "";
/** @return the expression for the value of annotation value 'payload'.
Can be used as alternative to {@link payload()} if the value is not constant. */
@Order(17)
public String payload_expr() default "";
/** @return a constant value for annotation value 'value'.
@see javax.validation.constraints.Min#value() */
@Order(18)
public long [] value() default {};
/** @return expression to determine if the annotation value 'value' shall be generated. Default is true. */
@Order(19)
public String value_cond() default "";
/** @return the expression language for the condition expression for 'value'. */
@Order(20)
public String value_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'value' shall be generated. */
@Order(21)
public Class> [] value_condFun() default {};
/** @return the src expression for generating annotation value 'value'. If this results in a collection, an array will be generated. */
@Order(22)
public String value_src() default "";
/** @return the expression for the value of annotation value 'value'.
Can be used as alternative to {@link value()} if the value is not constant. */
@Order(23)
public String value_expr() default "";
/** An expression to determine the source object for generating this annotation(s).
The source element is available as "src" in expressions and is used in
matchers and other rules. If the src expression is not set, the src
element of the element hosting the annotation is used.
@return */
@Order(24)
public String _src() default "";
/** @return the language of the src expression. Defaults to Java EL. */
@Order(25)
public String _srcLang() default "";
/** As an alternative to the src expression, a function can be called to determine the source object.
@return */
@Order(26)
public Class> [] _srcFun() default {};
/** @return expression to determine if the annotation shall be generated. Default is true. */
@Order(27)
public String _cond() default "";
/** @return the expression language for the condition expression. */
@Order(28)
public String _condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called. */
@Order(29)
public Class> [] _condFun() default {};
}
/** This annotation template will generate an annotation of type {@link javax.validation.constraints.NotNull}. */
@AnnotationTemplate(targetAnnotation = NotNull.class)
@Order(13)
public static @interface NotNull_ {
/** @return a constant value for annotation value 'message'.
@see javax.validation.constraints.NotNull#value() */
@Order(0)
public String [] message() default {};
/** @return expression to determine if the annotation value 'message' shall be generated. Default is true. */
@Order(1)
public String message_cond() default "";
/** @return the expression language for the condition expression for 'message'. */
@Order(2)
public String message_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'message' shall be generated. */
@Order(3)
public Class> [] message_condFun() default {};
/** @return the src expression for generating annotation value 'message'. If this results in a collection, an array will be generated. */
@Order(4)
public String message_src() default "";
/** @return the expression for the value of annotation value 'message'.
Can be used as alternative to {@link message()} if the value is not constant. */
@Order(5)
public String message_expr() default "";
/** @return a constant value for annotation value 'groups'.
@see javax.validation.constraints.NotNull#value() */
@Order(6)
public Class> [] groups() default {};
/** @return expression to determine if the annotation value 'groups' shall be generated. Default is true. */
@Order(7)
public String groups_cond() default "";
/** @return the expression language for the condition expression for 'groups'. */
@Order(8)
public String groups_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'groups' shall be generated. */
@Order(9)
public Class> [] groups_condFun() default {};
/** @return the src expression for generating annotation value 'groups'. If this results in a collection, an array will be generated. */
@Order(10)
public String groups_src() default "";
/** @return the expression for the value of annotation value 'groups'.
Can be used as alternative to {@link groups()} if the value is not constant. */
@Order(11)
public String groups_expr() default "";
/** @return a constant value for annotation value 'payload'.
@see javax.validation.constraints.NotNull#value() */
@Order(12)
public Class extends Payload> [] payload() default {};
/** @return expression to determine if the annotation value 'payload' shall be generated. Default is true. */
@Order(13)
public String payload_cond() default "";
/** @return the expression language for the condition expression for 'payload'. */
@Order(14)
public String payload_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'payload' shall be generated. */
@Order(15)
public Class> [] payload_condFun() default {};
/** @return the src expression for generating annotation value 'payload'. If this results in a collection, an array will be generated. */
@Order(16)
public String payload_src() default "";
/** @return the expression for the value of annotation value 'payload'.
Can be used as alternative to {@link payload()} if the value is not constant. */
@Order(17)
public String payload_expr() default "";
/** An expression to determine the source object for generating this annotation(s).
The source element is available as "src" in expressions and is used in
matchers and other rules. If the src expression is not set, the src
element of the element hosting the annotation is used.
@return */
@Order(18)
public String _src() default "";
/** @return the language of the src expression. Defaults to Java EL. */
@Order(19)
public String _srcLang() default "";
/** As an alternative to the src expression, a function can be called to determine the source object.
@return */
@Order(20)
public Class> [] _srcFun() default {};
/** @return expression to determine if the annotation shall be generated. Default is true. */
@Order(21)
public String _cond() default "";
/** @return the expression language for the condition expression. */
@Order(22)
public String _condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called. */
@Order(23)
public Class> [] _condFun() default {};
}
/** This annotation template will generate an annotation of type {@link javax.validation.constraints.Null}. */
@AnnotationTemplate(targetAnnotation = Null.class)
@Order(14)
public static @interface Null_ {
/** @return a constant value for annotation value 'message'.
@see javax.validation.constraints.Null#value() */
@Order(0)
public String [] message() default {};
/** @return expression to determine if the annotation value 'message' shall be generated. Default is true. */
@Order(1)
public String message_cond() default "";
/** @return the expression language for the condition expression for 'message'. */
@Order(2)
public String message_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'message' shall be generated. */
@Order(3)
public Class> [] message_condFun() default {};
/** @return the src expression for generating annotation value 'message'. If this results in a collection, an array will be generated. */
@Order(4)
public String message_src() default "";
/** @return the expression for the value of annotation value 'message'.
Can be used as alternative to {@link message()} if the value is not constant. */
@Order(5)
public String message_expr() default "";
/** @return a constant value for annotation value 'groups'.
@see javax.validation.constraints.Null#value() */
@Order(6)
public Class> [] groups() default {};
/** @return expression to determine if the annotation value 'groups' shall be generated. Default is true. */
@Order(7)
public String groups_cond() default "";
/** @return the expression language for the condition expression for 'groups'. */
@Order(8)
public String groups_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'groups' shall be generated. */
@Order(9)
public Class> [] groups_condFun() default {};
/** @return the src expression for generating annotation value 'groups'. If this results in a collection, an array will be generated. */
@Order(10)
public String groups_src() default "";
/** @return the expression for the value of annotation value 'groups'.
Can be used as alternative to {@link groups()} if the value is not constant. */
@Order(11)
public String groups_expr() default "";
/** @return a constant value for annotation value 'payload'.
@see javax.validation.constraints.Null#value() */
@Order(12)
public Class extends Payload> [] payload() default {};
/** @return expression to determine if the annotation value 'payload' shall be generated. Default is true. */
@Order(13)
public String payload_cond() default "";
/** @return the expression language for the condition expression for 'payload'. */
@Order(14)
public String payload_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'payload' shall be generated. */
@Order(15)
public Class> [] payload_condFun() default {};
/** @return the src expression for generating annotation value 'payload'. If this results in a collection, an array will be generated. */
@Order(16)
public String payload_src() default "";
/** @return the expression for the value of annotation value 'payload'.
Can be used as alternative to {@link payload()} if the value is not constant. */
@Order(17)
public String payload_expr() default "";
/** An expression to determine the source object for generating this annotation(s).
The source element is available as "src" in expressions and is used in
matchers and other rules. If the src expression is not set, the src
element of the element hosting the annotation is used.
@return */
@Order(18)
public String _src() default "";
/** @return the language of the src expression. Defaults to Java EL. */
@Order(19)
public String _srcLang() default "";
/** As an alternative to the src expression, a function can be called to determine the source object.
@return */
@Order(20)
public Class> [] _srcFun() default {};
/** @return expression to determine if the annotation shall be generated. Default is true. */
@Order(21)
public String _cond() default "";
/** @return the expression language for the condition expression. */
@Order(22)
public String _condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called. */
@Order(23)
public Class> [] _condFun() default {};
}
/** This annotation template will generate an annotation of type {@link javax.validation.constraints.Past}. */
@AnnotationTemplate(targetAnnotation = Past.class)
@Order(15)
public static @interface Past_ {
/** @return a constant value for annotation value 'message'.
@see javax.validation.constraints.Past#value() */
@Order(0)
public String [] message() default {};
/** @return expression to determine if the annotation value 'message' shall be generated. Default is true. */
@Order(1)
public String message_cond() default "";
/** @return the expression language for the condition expression for 'message'. */
@Order(2)
public String message_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'message' shall be generated. */
@Order(3)
public Class> [] message_condFun() default {};
/** @return the src expression for generating annotation value 'message'. If this results in a collection, an array will be generated. */
@Order(4)
public String message_src() default "";
/** @return the expression for the value of annotation value 'message'.
Can be used as alternative to {@link message()} if the value is not constant. */
@Order(5)
public String message_expr() default "";
/** @return a constant value for annotation value 'groups'.
@see javax.validation.constraints.Past#value() */
@Order(6)
public Class> [] groups() default {};
/** @return expression to determine if the annotation value 'groups' shall be generated. Default is true. */
@Order(7)
public String groups_cond() default "";
/** @return the expression language for the condition expression for 'groups'. */
@Order(8)
public String groups_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'groups' shall be generated. */
@Order(9)
public Class> [] groups_condFun() default {};
/** @return the src expression for generating annotation value 'groups'. If this results in a collection, an array will be generated. */
@Order(10)
public String groups_src() default "";
/** @return the expression for the value of annotation value 'groups'.
Can be used as alternative to {@link groups()} if the value is not constant. */
@Order(11)
public String groups_expr() default "";
/** @return a constant value for annotation value 'payload'.
@see javax.validation.constraints.Past#value() */
@Order(12)
public Class extends Payload> [] payload() default {};
/** @return expression to determine if the annotation value 'payload' shall be generated. Default is true. */
@Order(13)
public String payload_cond() default "";
/** @return the expression language for the condition expression for 'payload'. */
@Order(14)
public String payload_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'payload' shall be generated. */
@Order(15)
public Class> [] payload_condFun() default {};
/** @return the src expression for generating annotation value 'payload'. If this results in a collection, an array will be generated. */
@Order(16)
public String payload_src() default "";
/** @return the expression for the value of annotation value 'payload'.
Can be used as alternative to {@link payload()} if the value is not constant. */
@Order(17)
public String payload_expr() default "";
/** An expression to determine the source object for generating this annotation(s).
The source element is available as "src" in expressions and is used in
matchers and other rules. If the src expression is not set, the src
element of the element hosting the annotation is used.
@return */
@Order(18)
public String _src() default "";
/** @return the language of the src expression. Defaults to Java EL. */
@Order(19)
public String _srcLang() default "";
/** As an alternative to the src expression, a function can be called to determine the source object.
@return */
@Order(20)
public Class> [] _srcFun() default {};
/** @return expression to determine if the annotation shall be generated. Default is true. */
@Order(21)
public String _cond() default "";
/** @return the expression language for the condition expression. */
@Order(22)
public String _condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called. */
@Order(23)
public Class> [] _condFun() default {};
}
/** This annotation template will generate an annotation of type {@link javax.validation.constraints.Pattern}. */
@AnnotationTemplate(targetAnnotation = Pattern.class)
@Order(16)
public static @interface Pattern_ {
/** @return a constant value for annotation value 'regexp'.
@see javax.validation.constraints.Pattern#value() */
@Order(0)
public String [] regexp() default {};
/** @return expression to determine if the annotation value 'regexp' shall be generated. Default is true. */
@Order(1)
public String regexp_cond() default "";
/** @return the expression language for the condition expression for 'regexp'. */
@Order(2)
public String regexp_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'regexp' shall be generated. */
@Order(3)
public Class> [] regexp_condFun() default {};
/** @return the src expression for generating annotation value 'regexp'. If this results in a collection, an array will be generated. */
@Order(4)
public String regexp_src() default "";
/** @return the expression for the value of annotation value 'regexp'.
Can be used as alternative to {@link regexp()} if the value is not constant. */
@Order(5)
public String regexp_expr() default "";
/** @return a constant value for annotation value 'flags'.
@see javax.validation.constraints.Pattern#value() */
@Order(6)
public Flag [] flags() default {};
/** @return expression to determine if the annotation value 'flags' shall be generated. Default is true. */
@Order(7)
public String flags_cond() default "";
/** @return the expression language for the condition expression for 'flags'. */
@Order(8)
public String flags_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'flags' shall be generated. */
@Order(9)
public Class> [] flags_condFun() default {};
/** @return the src expression for generating annotation value 'flags'. If this results in a collection, an array will be generated. */
@Order(10)
public String flags_src() default "";
/** @return the expression for the value of annotation value 'flags'.
Can be used as alternative to {@link flags()} if the value is not constant. */
@Order(11)
public String flags_expr() default "";
/** @return a constant value for annotation value 'message'.
@see javax.validation.constraints.Pattern#value() */
@Order(12)
public String [] message() default {};
/** @return expression to determine if the annotation value 'message' shall be generated. Default is true. */
@Order(13)
public String message_cond() default "";
/** @return the expression language for the condition expression for 'message'. */
@Order(14)
public String message_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'message' shall be generated. */
@Order(15)
public Class> [] message_condFun() default {};
/** @return the src expression for generating annotation value 'message'. If this results in a collection, an array will be generated. */
@Order(16)
public String message_src() default "";
/** @return the expression for the value of annotation value 'message'.
Can be used as alternative to {@link message()} if the value is not constant. */
@Order(17)
public String message_expr() default "";
/** @return a constant value for annotation value 'groups'.
@see javax.validation.constraints.Pattern#value() */
@Order(18)
public Class> [] groups() default {};
/** @return expression to determine if the annotation value 'groups' shall be generated. Default is true. */
@Order(19)
public String groups_cond() default "";
/** @return the expression language for the condition expression for 'groups'. */
@Order(20)
public String groups_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'groups' shall be generated. */
@Order(21)
public Class> [] groups_condFun() default {};
/** @return the src expression for generating annotation value 'groups'. If this results in a collection, an array will be generated. */
@Order(22)
public String groups_src() default "";
/** @return the expression for the value of annotation value 'groups'.
Can be used as alternative to {@link groups()} if the value is not constant. */
@Order(23)
public String groups_expr() default "";
/** @return a constant value for annotation value 'payload'.
@see javax.validation.constraints.Pattern#value() */
@Order(24)
public Class extends Payload> [] payload() default {};
/** @return expression to determine if the annotation value 'payload' shall be generated. Default is true. */
@Order(25)
public String payload_cond() default "";
/** @return the expression language for the condition expression for 'payload'. */
@Order(26)
public String payload_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'payload' shall be generated. */
@Order(27)
public Class> [] payload_condFun() default {};
/** @return the src expression for generating annotation value 'payload'. If this results in a collection, an array will be generated. */
@Order(28)
public String payload_src() default "";
/** @return the expression for the value of annotation value 'payload'.
Can be used as alternative to {@link payload()} if the value is not constant. */
@Order(29)
public String payload_expr() default "";
/** An expression to determine the source object for generating this annotation(s).
The source element is available as "src" in expressions and is used in
matchers and other rules. If the src expression is not set, the src
element of the element hosting the annotation is used.
@return */
@Order(30)
public String _src() default "";
/** @return the language of the src expression. Defaults to Java EL. */
@Order(31)
public String _srcLang() default "";
/** As an alternative to the src expression, a function can be called to determine the source object.
@return */
@Order(32)
public Class> [] _srcFun() default {};
/** @return expression to determine if the annotation shall be generated. Default is true. */
@Order(33)
public String _cond() default "";
/** @return the expression language for the condition expression. */
@Order(34)
public String _condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called. */
@Order(35)
public Class> [] _condFun() default {};
}
/** This annotation template will generate an annotation of type {@link javax.validation.constraints.Size}. */
@AnnotationTemplate(targetAnnotation = Size.class)
@Order(17)
public static @interface Size_ {
/** @return a constant value for annotation value 'message'.
@see javax.validation.constraints.Size#value() */
@Order(0)
public String [] message() default {};
/** @return expression to determine if the annotation value 'message' shall be generated. Default is true. */
@Order(1)
public String message_cond() default "";
/** @return the expression language for the condition expression for 'message'. */
@Order(2)
public String message_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'message' shall be generated. */
@Order(3)
public Class> [] message_condFun() default {};
/** @return the src expression for generating annotation value 'message'. If this results in a collection, an array will be generated. */
@Order(4)
public String message_src() default "";
/** @return the expression for the value of annotation value 'message'.
Can be used as alternative to {@link message()} if the value is not constant. */
@Order(5)
public String message_expr() default "";
/** @return a constant value for annotation value 'groups'.
@see javax.validation.constraints.Size#value() */
@Order(6)
public Class> [] groups() default {};
/** @return expression to determine if the annotation value 'groups' shall be generated. Default is true. */
@Order(7)
public String groups_cond() default "";
/** @return the expression language for the condition expression for 'groups'. */
@Order(8)
public String groups_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'groups' shall be generated. */
@Order(9)
public Class> [] groups_condFun() default {};
/** @return the src expression for generating annotation value 'groups'. If this results in a collection, an array will be generated. */
@Order(10)
public String groups_src() default "";
/** @return the expression for the value of annotation value 'groups'.
Can be used as alternative to {@link groups()} if the value is not constant. */
@Order(11)
public String groups_expr() default "";
/** @return a constant value for annotation value 'payload'.
@see javax.validation.constraints.Size#value() */
@Order(12)
public Class extends Payload> [] payload() default {};
/** @return expression to determine if the annotation value 'payload' shall be generated. Default is true. */
@Order(13)
public String payload_cond() default "";
/** @return the expression language for the condition expression for 'payload'. */
@Order(14)
public String payload_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'payload' shall be generated. */
@Order(15)
public Class> [] payload_condFun() default {};
/** @return the src expression for generating annotation value 'payload'. If this results in a collection, an array will be generated. */
@Order(16)
public String payload_src() default "";
/** @return the expression for the value of annotation value 'payload'.
Can be used as alternative to {@link payload()} if the value is not constant. */
@Order(17)
public String payload_expr() default "";
/** @return a constant value for annotation value 'min'.
@see javax.validation.constraints.Size#value() */
@Order(18)
public int [] min() default {};
/** @return expression to determine if the annotation value 'min' shall be generated. Default is true. */
@Order(19)
public String min_cond() default "";
/** @return the expression language for the condition expression for 'min'. */
@Order(20)
public String min_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'min' shall be generated. */
@Order(21)
public Class> [] min_condFun() default {};
/** @return the src expression for generating annotation value 'min'. If this results in a collection, an array will be generated. */
@Order(22)
public String min_src() default "";
/** @return the expression for the value of annotation value 'min'.
Can be used as alternative to {@link min()} if the value is not constant. */
@Order(23)
public String min_expr() default "";
/** @return a constant value for annotation value 'max'.
@see javax.validation.constraints.Size#value() */
@Order(24)
public int [] max() default {};
/** @return expression to determine if the annotation value 'max' shall be generated. Default is true. */
@Order(25)
public String max_cond() default "";
/** @return the expression language for the condition expression for 'max'. */
@Order(26)
public String max_condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called to determine if the annotation value 'max' shall be generated. */
@Order(27)
public Class> [] max_condFun() default {};
/** @return the src expression for generating annotation value 'max'. If this results in a collection, an array will be generated. */
@Order(28)
public String max_src() default "";
/** @return the expression for the value of annotation value 'max'.
Can be used as alternative to {@link max()} if the value is not constant. */
@Order(29)
public String max_expr() default "";
/** An expression to determine the source object for generating this annotation(s).
The source element is available as "src" in expressions and is used in
matchers and other rules. If the src expression is not set, the src
element of the element hosting the annotation is used.
@return */
@Order(30)
public String _src() default "";
/** @return the language of the src expression. Defaults to Java EL. */
@Order(31)
public String _srcLang() default "";
/** As an alternative to the src expression, a function can be called to determine the source object.
@return */
@Order(32)
public Class> [] _srcFun() default {};
/** @return expression to determine if the annotation shall be generated. Default is true. */
@Order(33)
public String _cond() default "";
/** @return the expression language for the condition expression. */
@Order(34)
public String _condLang() default "";
/** @return as an alternative to the cond expression, a boolean function can be called. */
@Order(35)
public Class> [] _condFun() default {};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy