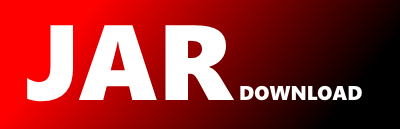
com.github.jasminb.jsonapi.JSONAPIDocument Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jsonapi-converter Show documentation
Show all versions of jsonapi-converter Show documentation
JSONAPI-Converter is a library that provides means for integrating with services using JSON API specification.
package com.github.jasminb.jsonapi;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.github.jasminb.jsonapi.models.errors.Error;
import java.util.HashMap;
import java.util.Map;
/**
* JSON API Document wrapper.
*
* @param the type parameter
* @author jbegic
*/
public class JSONAPIDocument {
private T data;
private ObjectMapper deserializer;
private Iterable extends Error> errors;
/**
* Top level response link object.
*/
private Links links;
/**
* A map of meta fields, keyed by the meta field name
*/
private Map meta;
/**
* Creates new JsonApiDocument.
*
* @param data {@link T} API resource type
*/
public JSONAPIDocument(T data) {
this.data = data;
}
/**
* Creates new JSONAPIDocument.
*
* @param data {@link T} API resource type
* @param deserializer {@link ObjectMapper} deserializer to be used for handling meta conversion
*/
public JSONAPIDocument(T data, ObjectMapper deserializer) {
this(data);
this.deserializer = deserializer;
}
/**
* Creates new JSONAPIDocument.
*/
public JSONAPIDocument() {
// Default constructor
}
/**
* Factory method for creating JSONAPIDocument that holds the Error object.
*
*
* This method should be used in case error response is being built by the server side.
*
* @param errors
*/
public static JSONAPIDocument> createErrorDocument(Iterable extends Error> errors) {
JSONAPIDocument> result = new JSONAPIDocument();
result.errors = errors;
return result;
}
/**
* Gets resource object
*
* @return {@link T} resource object
*/
public T get() {
return data;
}
/**
* Get meta data.
*
* @return {@link Map} meta
*/
public Map getMeta() {
return meta;
}
/**
* Sets meta data.
*
* @param meta {@link Map} meta
*/
public void setMeta(Map meta) {
this.meta = new HashMap<>(meta);
}
/**
* Gets links.
*
* @return the links
*/
public Links getLinks() {
return links;
}
/**
* Sets links.
*
* @param links the links
*/
public void setLinks(Links links) {
this.links = links;
}
/**
* Returns typed meta-data object or null
if no meta is present.
* @param metaType {@link Class} target type
* @param type
* @return meta or null
*/
public T getMeta(Class> metaType) {
if (meta != null && deserializer != null) {
return (T) deserializer.convertValue(meta, metaType);
}
return null;
}
/**
* Returns error objects or null
in case no errors were set.
* @return {@link Iterable} errors
*/
public Iterable extends Error> getErrors() {
return errors;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy