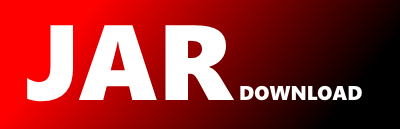
com.github.jasminb.jsonapi.ResourceCache Maven / Gradle / Ivy
Show all versions of jsonapi-converter Show documentation
package com.github.jasminb.jsonapi;
import java.util.HashMap;
import java.util.Map;
/**
* Resource caching provider.
*
* Cache implementation is based on thread-local variables. In order to use cache in a thread, init()
* method must be called. When cache is no longer needed, clear()
method should be used to perform
* thread local cleanup.
*
*
* Cache can be locked by invoking lock()
, after cache is locked, no items will be cached, and calling
* any cache method will be a no-op. To unlock cache and make it accept cache calls again, unlock()
* method must be invoked.
*
*
* If cache is used in recursive calls, it is safe to call init()
and clear()
* multiple times. Init call will not purge current cache state in case it is called recursively. Clear call will
* clear cache state only if recursion exited (initDepth == 0).
*
*
*
* @author jbegic
*/
public class ResourceCache {
private ThreadLocal