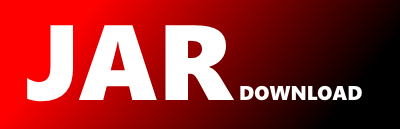
com.github.jasonruckman.uncompressed.AbstractBenchmark Maven / Gradle / Ivy
/**
* Copyright 2014 Jason Ruckman
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.github.jasonruckman.uncompressed;
import com.esotericsoftware.kryo.Kryo;
import com.esotericsoftware.kryo.io.Input;
import com.esotericsoftware.kryo.io.Output;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.github.jasonruckman.sidney.core.AbstractSid;
import com.github.jasonruckman.sidney.core.JavaSid;
import com.github.jasonruckman.sidney.core.Configuration;
import com.github.jasonruckman.sidney.core.type.TypeToken;
import com.github.jasonruckman.sidney.core.serde.Reader;
import com.github.jasonruckman.sidney.core.serde.Writer;
import org.openjdk.jmh.annotations.Scope;
import org.openjdk.jmh.annotations.Setup;
import org.openjdk.jmh.annotations.State;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
@State(Scope.Benchmark)
public abstract class AbstractBenchmark {
private final JavaSid javaSid;
private final JavaSid unsafeSid;
private final TypeToken token;
private final ThreadLocal threadLocalJackson = new ThreadLocal() {
@Override
protected ObjectMapper initialValue() {
return new ObjectMapper();
}
};
private final ThreadLocal threadLocalKryo = new ThreadLocal() {
@Override
protected Kryo initialValue() {
return new Kryo();
}
};
private final ThreadLocal> safeWriter = new ThreadLocal>() {
@Override
protected Writer initialValue() {
return javaSid.newWriter(token);
}
};
private final ThreadLocal> safeReader = new ThreadLocal>() {
@Override
protected Reader initialValue() {
return javaSid.newReader(token);
}
};
private final ThreadLocal> unsafeWriter = new ThreadLocal>() {
@Override
protected Writer initialValue() {
return unsafeSid.newWriter(token);
}
};
private final ThreadLocal> unsafeReader = new ThreadLocal>() {
@Override
protected Reader initialValue() {
return unsafeSid.newReader(token);
}
};
private final ThreadLocal
© 2015 - 2025 Weber Informatics LLC | Privacy Policy