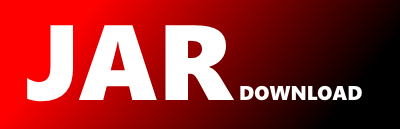
com.github.javaclub.cdl.client.group.AbstractDBSelector Maven / Gradle / Ivy
package com.github.javaclub.cdl.client.group;
import java.sql.SQLException;
import java.util.LinkedHashMap;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import javax.sql.DataSource;
import com.github.javaclub.cdl.client.common.DataSourceHolder;
import com.github.javaclub.cdl.client.common.ExceptionSorter;
import com.github.javaclub.cdl.client.common.MySQLExceptionSorter;
public abstract class AbstractDBSelector implements DBSelector {
private static final int default_retryBadDbInterval = 2000; // milliseconds
protected static int retryBadDbInterval = default_retryBadDbInterval;
protected ExceptionSorter exceptionSorter = new MySQLExceptionSorter();
protected T tryOnDataSourceHolder(DataSourceHolder dsHolder, Map failedDataSources,
DataSourceTryer tryer, int times, Object... args) throws SQLException {
List exceptions = new LinkedList();
if (failedDataSources != null) {
exceptions.addAll(failedDataSources.values());
}
if (failedDataSources != null && failedDataSources.containsKey(dsHolder.dsw)) {
return tryer.onSQLException(exceptions, exceptionSorter, args);
}
try {
if (dsHolder.isNotAvailable) {
boolean toTry = System.currentTimeMillis() - dsHolder.lastRetryTime > default_retryBadDbInterval;
if (toTry && dsHolder.lock.tryLock()) {
try {
T t = tryer.tryOnDataSource(dsHolder.dsw, args);
dsHolder.isNotAvailable = false;
return t;
} finally {
dsHolder.lastRetryTime = System.currentTimeMillis();
dsHolder.lock.unlock();
}
} else {
exceptions.add(new SQLException("dsKey:" + dsHolder.dsw.getDataSourceKey() + " not Available,toTry:" + toTry));
return tryer.onSQLException(exceptions, exceptionSorter, args);
}
} else {
return tryer.tryOnDataSource(dsHolder.dsw, args);
}
} catch (SQLException e) {
if (exceptionSorter.isExceptionFatal(e)) {
dsHolder.isNotAvailable = true;
}
exceptions.add(e);
return tryer.onSQLException(exceptions, exceptionSorter, args);
}
}
public T tryExecute(Map failedDataSources, DataSourceTryer tryer, int times, Object... args)
throws SQLException {
Integer dataSourceIndex = null;
if (args != null && args.length > 0) {
dataSourceIndex = (Integer) args[args.length - 1];
}
if (dataSourceIndex != null && dataSourceIndex != NOT_EXIST_USER_SPECIFIED_INDEX) {
DataSourceHolder dsHolder = findDataSourceWrapperByIndex(dataSourceIndex);
if (dsHolder == null) {
throw new IllegalArgumentException("找不到索引编号为 '" + dataSourceIndex + "'的数据源");
}
return tryOnDataSourceHolder(dsHolder, failedDataSources, tryer, times, args);
} else {
return tryExecuteInternal(failedDataSources, tryer, times, args);
}
}
public T tryExecute(DataSourceTryer tryer, int times, Object... args) throws SQLException {
return this.tryExecute(null, tryer, times, args);
}
protected abstract DataSourceHolder findDataSourceWrapperByIndex(int dataSourceIndex);
protected T tryExecuteInternal(DataSourceTryer tryer, int times, Object... args) throws SQLException {
return this.tryExecuteInternal(new LinkedHashMap(0), tryer, times, args);
}
protected abstract T tryExecuteInternal(Map failedDataSources, DataSourceTryer tryer, int times,
Object... args) throws SQLException;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy