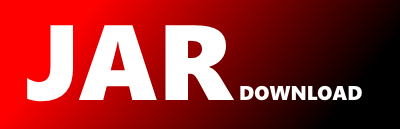
com.github.javaclub.cdl.client.group.SGroupDataSource Maven / Gradle / Ivy
package com.github.javaclub.cdl.client.group;
import java.io.PrintWriter;
import java.sql.Connection;
import java.sql.SQLException;
import java.sql.SQLFeatureNotSupportedException;
import java.util.concurrent.atomic.AtomicBoolean;
import java.util.logging.Logger;
import javax.sql.DataSource;
import com.github.javaclub.cdl.client.common.DataSourceWrapper;
import com.github.javaclub.cdl.client.config.DbConfigManager;
/**
* 一个group表示一组主备的db库,下面可以有若干atom持有数据源
*/
public class SGroupDataSource implements DataSource {
private String groupName;
private static ThreadLocal targetThreadLocal;
private int retryingTimes = 3;
private AtomicBoolean inited = new AtomicBoolean(false);
private DbConfigManager dbConfigManager;
public void init() throws SQLException {
if(inited.compareAndSet(false,true)){
dbConfigManager.registerSGroupDataSource(groupName, this);
}
}
public DataSourceWrapper getCurrentTarget() {
if (targetThreadLocal == null) {
return null;
}
DataSourceWrapper dsw = targetThreadLocal.get();
targetThreadLocal.remove();
return dsw;
}
/**
*/
void setWriteTarget(DataSourceWrapper dsw) {
if (targetThreadLocal != null) {
targetThreadLocal.set(dsw);
}
}
//当运行期间主备发生切换时是否需要查找第一个可写的库
private boolean autoSelectWriteDataSource = false;
public boolean getAutoSelectWriteDataSource() {
return autoSelectWriteDataSource;
}
public void setAutoSelectWriteDataSource(boolean autoSelectWriteDataSource) {
this.autoSelectWriteDataSource = autoSelectWriteDataSource;
}
DBSelector getDBSelector(boolean isRead) throws SQLException {
if(!inited.get()){
throw new SQLException("init method not be invoked at first, check your spring bean");
}
return dbConfigManager.getDBSelector(groupName, isRead);
}
/*---------------------------- jdbc 规范 ----------------------------------- */
private PrintWriter out = null;
@Override
public PrintWriter getLogWriter() throws SQLException {
return out;
}
@Override
public void setLogWriter(PrintWriter out) throws SQLException {
this.out = out;
}
private int seconds = 0;
@Override
public void setLoginTimeout(int seconds) throws SQLException {
this.seconds = seconds;
}
@Override
public int getLoginTimeout() throws SQLException {
return seconds;
}
@Override
public Logger getParentLogger() throws SQLFeatureNotSupportedException {
return null;
}
@SuppressWarnings("unchecked")
@Override
public T unwrap(Class iface) throws SQLException {
try {
return (T) this;
} catch (Exception e) {
throw new SQLException(e);
}
}
@Override
public boolean isWrapperFor(Class> iface) throws SQLException {
return this.getClass().isAssignableFrom(iface);
}
@Override
public Connection getConnection() throws SQLException {
if(!inited.get()){
throw new SQLException("init method not be invoked at first, check your spring bean");
}
return new SGroupConnection(this);
}
@Override
public Connection getConnection(String username, String password) throws SQLException {
if(!inited.get()){
throw new SQLException("init method not be invoked at first, check your spring bean");
}
return new SGroupConnection(this, username, password);
}
/*---------------------------- jdbc 规范 ----------------------------------- */
public int getRetryingTimes() {
return retryingTimes;
}
public void setRetryingTimes(int retryingTimes) {
this.retryingTimes = retryingTimes;
}
public String getGroupName() {
return groupName;
}
public void setGroupName(String groupName) {
this.groupName = groupName;
}
public DbConfigManager getDbConfigManager() {
return dbConfigManager;
}
public void setDbConfigManager(DbConfigManager dbConfigManager) {
this.dbConfigManager = dbConfigManager;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy