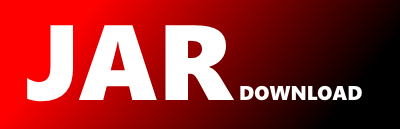
com.github.javaclub.cdl.client.matrix.jdbc.WrapperAdapter Maven / Gradle / Ivy
package com.github.javaclub.cdl.client.matrix.jdbc;
import java.sql.SQLException;
import java.sql.Wrapper;
import java.util.ArrayList;
import java.util.Collection;
public class WrapperAdapter implements Wrapper {
private Collection jdbcMethodInvocations = new ArrayList<>();
@SuppressWarnings("unchecked")
@Override
public final T unwrap(final Class iface) throws SQLException {
if (isWrapperFor(iface)) {
return (T) this;
}
throw new SQLException(String.format("[%s] cannot be unwrapped as [%s]", getClass().getName(), iface.getName()));
}
@Override
public final boolean isWrapperFor(final Class> iface) throws SQLException {
return iface.isInstance(this);
}
/**
* 记录方法调用.
*
* @param targetClass 目标类
* @param methodName 方法名称
* @param argumentTypes 参数类型
* @param arguments 参数
*/
protected final void recordMethodInvocation(final Class> targetClass, final String methodName, final Class>[] argumentTypes, final Object[] arguments) {
try {
jdbcMethodInvocations.add(new JdbcMethodInvocation(targetClass.getMethod(methodName, argumentTypes), arguments));
} catch (final NoSuchMethodException ex) {
throw new RuntimeException(ex);
}
}
/**
* 回放记录的方法调用.
*
* @param target 目标对象
*/
protected final void replayMethodsInvovation(final Object target) {
for (JdbcMethodInvocation each : jdbcMethodInvocations) {
each.invoke(target);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy