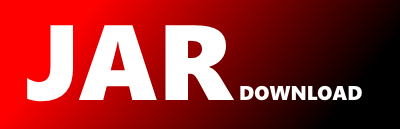
com.github.javaclub.configcenter.client.util.HttpHelper Maven / Gradle / Ivy
package com.github.javaclub.configcenter.client.util;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import org.apache.http.HttpEntity;
import org.apache.http.NameValuePair;
import org.apache.http.StatusLine;
import org.apache.http.client.config.RequestConfig;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClientBuilder;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.message.BasicNameValuePair;
import org.apache.http.util.EntityUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class HttpHelper {
private static final Logger logger = LoggerFactory.getLogger(HttpHelper.class);
private static CloseableHttpClient getHttpClient() {
HttpClientBuilder httpClientBuilder = HttpClients.custom();
String name = "Apache-HttpClient";
String release = "4.5";
String javaVersion = System.getProperty("java.version");
String userAgent = String.format("%s/%s (Java/%s)", name, release, javaVersion);
httpClientBuilder.setUserAgent(userAgent);
httpClientBuilder.setDefaultRequestConfig(RequestConfig.custom().setConnectTimeout(3000).build());
return httpClientBuilder.build();
}
public static String post(String uri, Map params) throws Exception {
CloseableHttpClient httpclient = getHttpClient();
// 创建httppost
HttpPost httppost = new HttpPost(uri);
// 创建参数队列
List formparams = new ArrayList();
if (params != null && !params.isEmpty()) {
for (String key : params.keySet()) {
formparams.add(new BasicNameValuePair(key, params.get(key)));
}
}
UrlEncodedFormEntity uefEntity;
try {
uefEntity = new UrlEncodedFormEntity(formparams, "UTF-8");
httppost.setEntity(uefEntity);
CloseableHttpResponse response = httpclient.execute(httppost);
try {
HttpEntity entity = response.getEntity();
if (entity != null) {
return EntityUtils.toString(entity, "UTF-8");
}
} finally {
response.close();
}
} catch (Exception e) {
throw e;
} finally {
// 关闭连接,释放资源
try {
httpclient.close();
} catch (Exception e) {
throw e;
}
}
return null;
}
public static String post(String uri, String text) throws Exception {
CloseableHttpClient httpclient = getHttpClient();
// 创建httppost
HttpPost httppost = new HttpPost(uri);
try {
StringEntity se = new StringEntity(text, "UTF-8");
httppost.setEntity(se);
CloseableHttpResponse response = httpclient.execute(httppost);
try {
StatusLine statusLine = response.getStatusLine();
int code = 200;
if (statusLine != null) {
code = response.getStatusLine().getStatusCode();
}
if (200 != code) {
logger.warn("post uri:{},code:{}", uri, code);
}
HttpEntity entity = response.getEntity();
if (entity != null) {
String r = EntityUtils.toString(entity, "UTF-8");
if (200 != code) {
logger.warn("resp:{}", r);
}
return r;
}
} finally {
response.close();
}
} catch (Exception e) {
throw e;
} finally {
// 关闭连接,释放资源
try {
httpclient.close();
} catch (Exception e) {
throw e;
}
}
return null;
}
/**
* 发送 get请求
*
* @throws Exception
*/
public static String get(String url) throws Exception {
CloseableHttpClient httpclient = getHttpClient();
try {
// 创建httpget.
HttpGet httpget = new HttpGet(url);
// 执行get请求.
CloseableHttpResponse response = httpclient.execute(httpget);
try {
// 获取响应实体
HttpEntity entity = response.getEntity();
StatusLine statusLine = response.getStatusLine();
int code = 200;
if (statusLine != null) {
code = response.getStatusLine().getStatusCode();
}
if (200 != code) {
logger.warn("get uri:{},code:{}", url, code);
}
// 打印响应状态
// System.out.println(response.getStatusLine());
if (entity != null) {
String r = EntityUtils.toString(entity, "UTF-8");
if (200 != code) {
logger.warn("resp:{}", r);
}
return r;
}
} finally {
response.close();
}
} catch (Exception e) {
throw e;
} finally {
// 关闭连接,释放资源
try {
httpclient.close();
} catch (IOException e) {
throw e;
}
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy