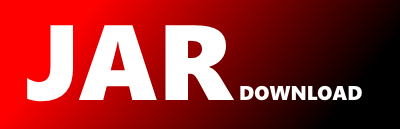
com.github.javaclub.configcenter.client.conf.LocalConfigserverConf Maven / Gradle / Ivy
The newest version!
/*
* @(#)LocalConfigserverConf.java 2021-8-24
*
* Copyright (c) 2021. All Rights Reserved.
*
*/
package com.github.javaclub.configcenter.client.conf;
import java.util.List;
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.annotation.JSONField;
import com.github.javaclub.Constants.SepChars;
import com.github.javaclub.configcenter.ConfigServerConstants.BootConfig;
import com.github.javaclub.configcenter.client.DefaultConfigCenterClient;
import com.github.javaclub.configcenter.client.util.Utils;
import com.github.javaclub.toolbox.conf.CompositeAppConfigProperties;
import com.google.common.base.Splitter;
import com.google.common.base.Strings;
import com.google.common.collect.Lists;
/**
* Configserver的本地应用启动的一些配置项
*
* @author Gerald Chen
* @version $Id: LocalConfigserverConf.java 2021-8-24 10:29:22 Exp $
*/
public class LocalConfigserverConf {
private static class SingletonHolder {
private static final LocalConfigserverConf INSTANCE = new LocalConfigserverConf();
}
/**
* 是否启用了 configserver 来进行配置管理
*/
private boolean enabled;
/**
* configserver 的请求服务访问地址,配置形式如:192.168.31.216:7001;192.168.31.60:7001;config.idev.com
*/
private String serverAddress;
/**
* 当前启动应用的 appId
*/
private int appId;
/**
* 当前应用名称
*/
private String appName;
/**
* 当前应用访问configserver的授权key
*/
@JSONField(serialize = false)
private String appKey;
private boolean onlyOneServer;
private boolean isLocal;
/**
* 是否允许内网IP直接请求
*/
private boolean intranetAllowed;
/**
* 选择固定的内网IP作为通讯节点
*/
private String fixedNode;
private LocalConfigserverConf() {
CompositeAppConfigProperties conf = CompositeAppConfigProperties.getInstance();
this.isLocal = conf.boolValue(BootConfig.CONFIGSERVER_ISLOCAL);
this.onlyOneServer = conf.boolValue(BootConfig.CONFIGSERVER_ONLYONE);
this.enabled = conf.boolValue(BootConfig.CONFIGSERVER_ENABLED);
this.appId = conf.intValue(BootConfig.CONFIGSERVER_APPID);
this.appKey = conf.getValue(BootConfig.CONFIGSERVER_APPKEY);
this.appName = conf.getValue(BootConfig.CONFIGSERVER_APPNAME);
this.serverAddress = conf.getValue(BootConfig.CONFIGSERVER_ADDRESS);
this.intranetAllowed = conf.boolValue(BootConfig.CONFIGSERVER_INTRANET_ALLOWED);
this.fixedNode = conf.getValue(BootConfig.CONFIGSERVER_SLECTED_FIXED_NODE);
}
public static final LocalConfigserverConf getInstance() {
LocalConfigserverConf conf = SingletonHolder.INSTANCE;
if (conf.enabled && 0 < conf.appId && Utils.isNotBlank(conf.appKey)) {
DefaultConfigCenterClient.getInstance().init(conf.appId, conf.appKey);
}
return conf;
}
public boolean isEnabled() {
return enabled;
}
public void setEnabled(boolean enabled) {
this.enabled = enabled;
}
public String getServerAddress() {
return serverAddress;
}
public void setServerAddress(String serverAddress) {
this.serverAddress = serverAddress;
}
public int getAppId() {
return appId;
}
public String getAppName() {
return appName;
}
public String getAppKey() {
return appKey;
}
public boolean isOnlyOneServer() {
return onlyOneServer;
}
public boolean isLocal() {
return isLocal;
}
public boolean isIntranetAllowed() {
return intranetAllowed;
}
public String getFixedNode() {
return fixedNode;
}
@JSONField(serialize = false)
public List getServerList() {
if (Strings.isNullOrEmpty(serverAddress)) {
return Lists.newArrayList();
}
return Splitter.on(SepChars.SEMICOLON).trimResults().splitToList(serverAddress);
}
@Override
public String toString() {
return JSON.toJSONString(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy