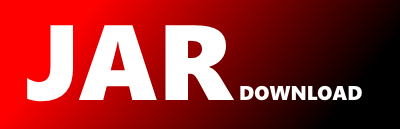
com.github.javaclub.configcenter.client.domain.DurationParser Maven / Gradle / Ivy
The newest version!
/*
* @(#)DurationParser.java 2021-8-24
*
* Copyright (c) 2021. All Rights Reserved.
*
*/
package com.github.javaclub.configcenter.client.domain;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import com.github.javaclub.configcenter.client.ParserException;
/**
* DurationParser
*
* @author Gerald Chen
* @version $Id: DurationParser.java 2021-8-24 16:30:10 Exp $
*/
public enum DurationParser {
INSTANCE;
private static final Pattern PATTERN = Pattern.compile(
"(?:([0-9]+)D)?(?:([0-9]+)H)?(?:([0-9]+)M)?(?:([0-9]+)S)?(?:([0-9]+)(?:MS)?)?", Pattern.CASE_INSENSITIVE);
private static final int HOURS_PER_DAY = 24;
private static final int MINUTES_PER_HOUR = 60;
private static final int SECONDS_PER_MINUTE = 60;
private static final int MILLIS_PER_SECOND = 1000;
private static final int MILLIS_PER_MINUTE = MILLIS_PER_SECOND * SECONDS_PER_MINUTE;
private static final int MILLIS_PER_HOUR = MILLIS_PER_MINUTE * MINUTES_PER_HOUR;
private static final int MILLIS_PER_DAY = MILLIS_PER_HOUR * HOURS_PER_DAY;
public long parseToMillis(String text) throws ParserException {
Matcher matcher = PATTERN.matcher(text);
if (matcher.matches()) {
String dayMatch = matcher.group(1);
String hourMatch = matcher.group(2);
String minuteMatch = matcher.group(3);
String secondMatch = matcher.group(4);
String fractionMatch = matcher.group(5);
if (dayMatch != null || hourMatch != null || minuteMatch != null || secondMatch != null
|| fractionMatch != null) {
int daysAsMilliSecs = parseNumber(dayMatch, MILLIS_PER_DAY);
int hoursAsMilliSecs = parseNumber(hourMatch, MILLIS_PER_HOUR);
int minutesAsMilliSecs = parseNumber(minuteMatch, MILLIS_PER_MINUTE);
int secondsAsMilliSecs = parseNumber(secondMatch, MILLIS_PER_SECOND);
int milliseconds = parseNumber(fractionMatch, 1);
return daysAsMilliSecs + hoursAsMilliSecs + minutesAsMilliSecs + secondsAsMilliSecs + milliseconds;
}
}
throw new ParserException(String.format("Text %s cannot be parsed to duration)", text));
}
private static int parseNumber(String parsed, int multiplier) {
// regex limits to [0-9]+
if (parsed == null || parsed.trim().isEmpty()) {
return 0;
}
return Integer.parseInt(parsed) * multiplier;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy