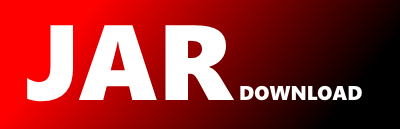
com.github.javaclub.configcenter.client.domain.ValueWrapper Maven / Gradle / Ivy
The newest version!
/*
* @(#)ValueWrapper.java 2021-8-24
*
* Copyright (c) 2021. All Rights Reserved.
*
*/
package com.github.javaclub.configcenter.client.domain;
import java.io.Serializable;
import java.util.Date;
import java.util.List;
import com.alibaba.fastjson.JSON;
import com.github.javaclub.configcenter.client.ParserException;
import com.github.javaclub.configcenter.client.util.Utils;
import com.google.common.base.Splitter;
import com.google.common.base.Strings;
/**
* ValueWrapper 字符串数据包装类型
*
* @author Gerald Chen
* @version $Id: ValueWrapper.java 2021-8-24 16:10:57 Exp $
*/
public class ValueWrapper implements Serializable {
private static final long serialVersionUID = 1L;
private String value;
public ValueWrapper() {
}
public ValueWrapper(String value) {
this.value = value;
}
public static ValueWrapper create(String value) {
return new ValueWrapper(value);
}
public void setValue(String value) {
this.value = value;
}
public boolean isNullOrBlank() {
return (null == this.value || "".equals(this.value.trim()));
}
public String getValue() {
return value;
}
public byte byteValue() {
return Byte.parseByte(getValue());
}
public byte byteValue(byte defaultValue) {
try {
return Byte.parseByte(getValue());
} catch (NumberFormatException e) {
return defaultValue;
}
}
public short shortValue() {
return Short.parseShort(getValue());
}
public short shortValue(short defaultValue) {
try {
return Short.parseShort(getValue());
} catch (NumberFormatException e) {
return defaultValue;
}
}
public int intValue() {
return Integer.parseInt(getValue());
}
public int intValue(int defaultValue) {
try {
return Integer.parseInt(getValue());
} catch (NumberFormatException e) {
return defaultValue;
}
}
public long longValue() {
return Long.parseLong(getValue());
}
public long longValue(long defaultValue) {
try {
return Long.parseLong(getValue());
} catch (NumberFormatException e) {
return defaultValue;
}
}
public float floatValue() {
return Float.parseFloat(getValue());
}
public float floatValue(float defaultValue) {
try {
return Float.parseFloat(getValue());
} catch (NumberFormatException e) {
return defaultValue;
}
}
public double doubleValue() {
return Double.parseDouble(getValue());
}
public double doubleValue(double defaultValue) {
try {
return Double.parseDouble(getValue());
} catch (NumberFormatException e) {
return defaultValue;
}
}
public boolean booleanValue() {
return ("true".equalsIgnoreCase(getValue()) ||
"T".equalsIgnoreCase(getValue()) ||
"1".equalsIgnoreCase(getValue()));
}
public List arrayValue(final String delimiter, List defaultValue) {
String value = getValue();
if(Strings.isNullOrEmpty(value)) {
return defaultValue;
}
return Splitter.on(delimiter).trimResults().splitToList(value);
}
public Date datetimeValue(String format, Date defaultValue) {
String value = getValue();
if(Strings.isNullOrEmpty(value)) {
return defaultValue;
}
Long timestamp = Utils.parseLong(value);
if (null != timestamp && timestamp > 0) {
return new Date(timestamp);
}
Date datetime = Utils.toDate(value.trim(), format);
return (null == datetime ? defaultValue : datetime);
}
public T objectValue(Class clazz) {
String val = getValue();
if (Strings.isNullOrEmpty(val)) {
return null;
}
return JSON.parseObject(val.trim(), clazz);
}
/**
* Return the duration property value(in milliseconds) with the given name, or {@code
* defaultValue} if the name doesn't exist. Please note the format should comply with the follow
* example (case insensitive). Examples:
*
* "123MS" -- parses as "123 milliseconds"
* "20S" -- parses as "20 seconds"
* "15M" -- parses as "15 minutes" (where a minute is 60 seconds)
* "10H" -- parses as "10 hours" (where an hour is 3600 seconds)
* "2D" -- parses as "2 days" (where a day is 24 hours or 86400 seconds)
* "2D3H4M5S123MS" -- parses as "2 days, 3 hours, 4 minutes, 5 seconds and 123 milliseconds"
*
*
* @param key the property name
* @param defaultValue the default value when name is not found or any error occurred
* @return the parsed property value(in milliseconds)
*/
public long durationValue(long defaultValue) {
try {
return DurationParser.INSTANCE.parseToMillis(getValue());
} catch (ParserException e) {
return defaultValue;
}
}
@Override
public String toString() {
return "ValueWrapper => {value=" + value + "}";
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + ((value == null) ? 0 : value.hashCode());
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
ValueWrapper other = (ValueWrapper) obj;
if (value == null) {
if (other.value != null)
return false;
} else if (!value.equals(other.value))
return false;
return true;
}
public static void main(String[] args) {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy