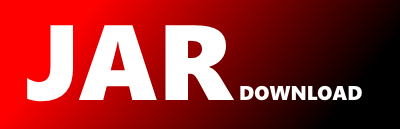
com.github.javaclub.configcenter.client.util.HttpHelper Maven / Gradle / Ivy
The newest version!
package com.github.javaclub.configcenter.client.util;
import java.io.IOException;
import java.io.InterruptedIOException;
import java.net.URLDecoder;
import java.net.URLEncoder;
import java.net.UnknownHostException;
import java.security.cert.CertificateException;
import java.security.cert.X509Certificate;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import java.util.Map;
import java.util.concurrent.TimeUnit;
import javax.net.ssl.SSLContext;
import javax.net.ssl.SSLException;
import javax.net.ssl.SSLHandshakeException;
import org.apache.http.HttpEntity;
import org.apache.http.HttpEntityEnclosingRequest;
import org.apache.http.HttpHost;
import org.apache.http.HttpRequest;
import org.apache.http.NameValuePair;
import org.apache.http.NoHttpResponseException;
import org.apache.http.StatusLine;
import org.apache.http.client.HttpRequestRetryHandler;
import org.apache.http.client.config.RequestConfig;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.client.methods.HttpRequestBase;
import org.apache.http.client.protocol.HttpClientContext;
import org.apache.http.config.Registry;
import org.apache.http.config.RegistryBuilder;
import org.apache.http.config.SocketConfig;
import org.apache.http.conn.ConnectTimeoutException;
import org.apache.http.conn.HttpClientConnectionManager;
import org.apache.http.conn.routing.HttpRoute;
import org.apache.http.conn.socket.ConnectionSocketFactory;
import org.apache.http.conn.socket.PlainConnectionSocketFactory;
import org.apache.http.conn.ssl.NoopHostnameVerifier;
import org.apache.http.conn.ssl.SSLConnectionSocketFactory;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.impl.conn.PoolingHttpClientConnectionManager;
import org.apache.http.message.BasicNameValuePair;
import org.apache.http.pool.PoolStats;
import org.apache.http.protocol.HttpContext;
import org.apache.http.ssl.SSLContextBuilder;
import org.apache.http.ssl.TrustStrategy;
import org.apache.http.util.EntityUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.github.javaclub.AppBootConstants;
import com.github.javaclub.Constants.Contexts;
import com.github.javaclub.configcenter.ConfigServerConstants.Client;
import com.github.javaclub.toolbox.ToolBox.Objects;
import com.github.javaclub.toolbox.thread.ExecutorServiceInstance;
import com.google.common.collect.Maps;
public class HttpHelper {
private static final Logger logger = LoggerFactory.getLogger(HttpHelper.class);
private static final int DEFAULT_TIMEOUT = 10000; // 默认超时时间,单位:ms
private static final int CONN_ALLOC_TIMEOUT = 3000; // 从connectManager获取 Connection 的超时时间,单位:ms
private static final int CONN_DEST_TIMEOUT = 5000; // 连接目标地址超时设置,单位:ms
private static final int SOCK_READ_TIMEOUT = 8000; // 读取超时设置,单位:ms
private static final Object syncLock = new Object();
private static CloseableHttpClient httpClient = null;
private static void config(HttpRequestBase httpRequestBase) {
// 配置请求的超时设置
RequestConfig requestConfig = RequestConfig.custom()
.setConnectionRequestTimeout(CONN_ALLOC_TIMEOUT)
.setConnectTimeout(CONN_DEST_TIMEOUT).setSocketTimeout(SOCK_READ_TIMEOUT).build();
httpRequestBase.setConfig(requestConfig);
}
public static String post(String url, Map params) throws Exception {
CloseableHttpClient httpclient = getHttpClient(url);
// 创建httppost
HttpPost httppost = new HttpPost(url);
try {
config(httppost);
// 创建参数队列
List formparams = new ArrayList();
if (params != null && !params.isEmpty()) {
for (String key : params.keySet()) {
formparams.add(new BasicNameValuePair(key, params.get(key)));
}
}
UrlEncodedFormEntity uefEntity = new UrlEncodedFormEntity(formparams, "UTF-8");
httppost.setEntity(uefEntity);
httppost.addHeader(Contexts.TRACE_ID, Contexts.currentTraceId());
CloseableHttpResponse response = httpclient.execute(httppost, HttpClientContext.create());
try {
HttpEntity entity = response.getEntity();
if (entity != null) {
return EntityUtils.toString(entity, "UTF-8");
}
} finally {
Utils.closeQuietly(response);
}
} catch (Exception e) {
throw e;
} finally {
try {
httppost.releaseConnection();
} catch (Exception e) {
}
// 关闭连接,释放资源
//Utils.closeQuietly(httpclient);
}
return null;
}
public static String post(String url, String text) throws Exception {
CloseableHttpClient httpclient = getHttpClient(url);
// 创建httppost
HttpPost httppost = new HttpPost(url);
try {
config(httppost);
StringEntity se = new StringEntity(text, "UTF-8");
httppost.setEntity(se);
httppost.addHeader("Content-Type", "application/json; charset=UTF-8");
httppost.addHeader(Contexts.TRACE_ID, Contexts.currentTraceId());
CloseableHttpResponse response = httpclient.execute(httppost, HttpClientContext.create());
try {
StatusLine statusLine = response.getStatusLine();
int code = 200;
if (statusLine != null) {
code = response.getStatusLine().getStatusCode();
}
if (200 != code) {
logger.warn("HttpPost: code={}, url={}", code, url);
}
HttpEntity entity = response.getEntity();
if (entity != null) {
String r = EntityUtils.toString(entity, "UTF-8");
if (200 != code) {
// logger.warn("resp:{}", r);
ExecutorServiceInstance.get(Client.CLIENT_WORKER_ALARM_MONITOR).submit(new Runnable() {
@Override
public void run() {
Map map = Maps.newHashMap();
map.put("请求地址", url);
map.put("异常明细", Utils.lessText(r, 60, true));
DingTalkUtils.sendMarkdown("Configcenter连接失败", new Date(), map);
}
});
}
return r;
}
} finally {
Utils.closeQuietly(response);
}
} catch (Exception e) {
throw e;
} finally {
try {
httppost.releaseConnection();
} catch (Exception e) {
}
// 关闭连接,释放资源
// Utils.closeQuietly(httpclient);
}
return null;
}
public static String get(String url, boolean alert) throws Exception {
CloseableHttpClient httpclient = getHttpClient(url);
// 创建httpget.
HttpGet httpget = new HttpGet(url);
try {
httpget.addHeader("Accept", "*/*");
httpget.addHeader("Accept-Encoding", "gzip, deflate, br");
httpget.addHeader("Accept-Language", "zh-CN,zh;q=0.9");
httpget.addHeader("Cache-Control", "no-cache");
httpget.addHeader("Connection", "keep-alive");
httpget.addHeader("User-Agent", "Mozilla/5.0 (Macintosh; Intel Mac OS X 10.13; rv:86.0) Gecko/20100101 Firefox/86.0");
httpget.addHeader(Contexts.TRACE_ID, Contexts.currentTraceId());
// 执行get请求.
CloseableHttpResponse response = httpclient.execute(httpget, HttpClientContext.create());
try {
// 获取响应实体
HttpEntity entity = response.getEntity();
StatusLine statusLine = response.getStatusLine();
int code = 200;
if (statusLine != null) {
code = response.getStatusLine().getStatusCode();
}
if (200 != code) {
logger.warn("HttpGet: code={}, url={}", code, url);
}
if (entity != null) {
String r = EntityUtils.toString(entity, "UTF-8");
if (200 != code) {
// logger.warn("resp:{}", r);
if (alert) {
ExecutorServiceInstance.get(Client.CLIENT_WORKER_ALARM_MONITOR).submit(new Runnable() {
@Override
public void run() {
Map map = Maps.newLinkedHashMap();
map.put("请求地址", url);
map.put("异常明细", Utils.lessText(r, 60, true));
DingTalkUtils.sendMarkdown("ConfigClient Connect ServerNode Error", new Date(), map);
}
});
}
}
return r;
}
} finally {
Utils.closeQuietly(response);
}
} catch (Exception e) {
throw e;
} finally {
try {
httpget.releaseConnection();
} catch (Exception e) {
}
// 关闭连接,释放资源
// Utils.closeQuietly(httpclient);
}
return null;
}
/**
* 发送 get请求
*
* @throws Exception
*/
public static String get(String url) throws Exception {
return get(url, true);
}
public static String urlencode(String param) {
String newVal = param;
try {
newVal = URLEncoder.encode(param, "UTF-8");
} catch (Exception e) {
newVal = param;
}
return newVal;
}
public static String urldecode(String param) {
String newVal = param;
try {
newVal = URLDecoder.decode(param, "UTF-8");
} catch (Exception e) {
newVal = param;
}
return newVal;
}
public static CloseableHttpClient getHttpClient(String url) {
Objects.requireNotEmpty(url, "请求URL地址不能为空!");
if (httpClient == null) {
synchronized (syncLock) {
if (httpClient == null) {
httpClient = createHttpClient(200, 30, 100, url);
}
}
}
return httpClient;
}
public static CloseableHttpClient createHttpClient(int maxTotal, int maxPerRoute, int maxRoute, String url) {
SSLContext sslContext = null;
try {
sslContext = new SSLContextBuilder().loadTrustMaterial(null, new TrustStrategy() {
public boolean isTrusted(X509Certificate[] chain, String authType)
throws CertificateException {
return true;
}
}).build();
} catch (Exception e) {
throw new RuntimeException(e.getMessage());
}
SSLConnectionSocketFactory sslsf = new SSLConnectionSocketFactory(sslContext, NoopHostnameVerifier.INSTANCE);
ConnectionSocketFactory plainsf = PlainConnectionSocketFactory.getSocketFactory();
Registry registry = RegistryBuilder. create()
.register("http", plainsf)
.register("https", sslsf)
.build();
PoolingHttpClientConnectionManager cm = new PoolingHttpClientConnectionManager(registry);
// 将最大连接数增加
cm.setMaxTotal(maxTotal);
// 将每个路由基础的连接增加
cm.setDefaultMaxPerRoute(maxPerRoute);
cm.setValidateAfterInactivity(2000);
cm.setDefaultSocketConfig(SocketConfig.custom().setSoTimeout(DEFAULT_TIMEOUT).setTcpNoDelay(true).build());
HttpHost httpHost = HttpHost.create(url);
// 将目标主机的最大连接数增加
cm.setMaxPerRoute(new HttpRoute(httpHost), maxRoute);
IdleConnectionReaper.setIdleConnectionTime(60 * 1000);
IdleConnectionReaper.registerConnectionManager(cm);
// 请求重试处理
HttpRequestRetryHandler httpRequestRetryHandler = new HttpRequestRetryHandler() {
public boolean retryRequest(IOException exception,
int executionCount, HttpContext context) {
if (executionCount >= 3) {// 如果已经重试了3次,就放弃
return false;
}
if (exception instanceof NoHttpResponseException) {// 如果服务器丢掉了连接,那么就重试
return true;
}
if (exception instanceof SSLHandshakeException) {// 不要重试SSL握手异常
return false;
}
if (exception instanceof InterruptedIOException) {// 超时
return false;
}
if (exception instanceof UnknownHostException) {// 目标服务器不可达
return false;
}
if (exception instanceof ConnectTimeoutException) {// 连接被拒绝
return false;
}
if (exception instanceof SSLException) {// SSL握手异常
return false;
}
HttpClientContext clientContext = HttpClientContext.adapt(context);
HttpRequest request = clientContext.getRequest();
// 如果请求是幂等的,就再次尝试
if (!(request instanceof HttpEntityEnclosingRequest)) {
return true;
}
return false;
}
};
String name = "Javaclub-Configcenter-HttpClient";
String release = "4.5";
String javaVersion = System.getProperty("java.version");
String userAgent = String.format("%s/%s (Java/%s)", name, release, javaVersion);
CloseableHttpClient httpClient = HttpClients.custom()
.setUserAgent(userAgent)
.setConnectionManager(cm)
.setRetryHandler(httpRequestRetryHandler).build();
return httpClient;
}
private static final class IdleConnectionReaper extends Thread {
private static long idleConnectionTime = 60 * 1000; // 毫秒
private static final int REAP_INTERVAL_MILLISECONDS = 5 * 1000;
private volatile boolean shuttingDown;
private static IdleConnectionReaper instance;
private static final ArrayList connectionManagers = new ArrayList();
private IdleConnectionReaper() {
super("httpclient_idle_connection_reaper");
setDaemon(true);
}
public static synchronized boolean registerConnectionManager(HttpClientConnectionManager connectionManager) {
if (instance == null) {
instance = new IdleConnectionReaper();
instance.start();
}
return connectionManagers.add(connectionManager);
}
public static synchronized boolean removeConnectionManager(HttpClientConnectionManager connectionManager) {
boolean b = connectionManagers.remove(connectionManager);
if (connectionManagers.isEmpty()) {
shutdown();
}
return b;
}
private void markShuttingDown() {
shuttingDown = true;
}
@Override
public void run() {
while (true) {
if (shuttingDown) {
logger.warn("Shutting down reaper thread.");
return;
}
try {
Thread.sleep(REAP_INTERVAL_MILLISECONDS);
} catch (InterruptedException e) {
}
try {
List connectionManagers = null;
synchronized (IdleConnectionReaper.class) {
connectionManagers = (List) IdleConnectionReaper.connectionManagers.clone();
}
for (HttpClientConnectionManager connectionManager : connectionManagers) {
try {
connectionManager.closeExpiredConnections();
connectionManager.closeIdleConnections(idleConnectionTime, TimeUnit.MILLISECONDS);
} catch (Exception ex) {
logger.error("Unable to close idle connections");
}
if (AppBootConstants.isLoggerEnabled("logger.switch.http") && logger.isInfoEnabled()) {
if (null != connectionManager && connectionManager instanceof PoolingHttpClientConnectionManager) {
PoolStats ps = ((PoolingHttpClientConnectionManager) connectionManager).getTotalStats();
if (null != ps) {
logger.info("HttpClient Pooling statistics: {}", ps.toString());
}
}
}
}
} catch (Throwable t) {
logger.error("Error when closeExpiredConnections or closeIdleConnections: ", t);
}
}
}
public static synchronized boolean shutdown() {
if (instance != null) {
instance.markShuttingDown();
instance.interrupt();
connectionManagers.clear();
instance = null;
return true;
}
return false;
}
public static synchronized int size() {
return connectionManagers.size();
}
public static synchronized void setIdleConnectionTime(long idletime) {
idleConnectionTime = idletime;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy