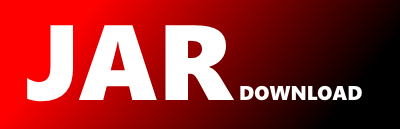
com.github.javaclub.configcenter.client.util.PropUtil Maven / Gradle / Ivy
The newest version!
/*
* @(#)PropUtil.java 2009-2-19
*
* Copyright (c) 2009 by gerald. All Rights Reserved.
*/
package com.github.javaclub.configcenter.client.util;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.net.URL;
import java.util.Date;
import java.util.HashMap;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Properties;
import java.util.ResourceBundle;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* A utility class for processing Properties
*
* @author Gerald Chen
*/
public class PropUtil {
private static final Logger log = LoggerFactory.getLogger(PropUtil.class);
/**
* Holds configuration objects
*/
private static Map map = new HashMap();
/**
* The filename of default configuration file
*/
private static final String DEFAULT_CONFIG_FILE = "server";
private PropUtil() {
}
/**
* Gets system property value by key,return value valIfNull
if
* null.
*
* @param key the key
* @return
*/
public static String getSystemProperty(String key, String valIfNull) {
String val = getSystemProperty(key);
return val == null ? valIfNull : val;
}
/**
*
* Gets a System property, defaulting to null
if the property
* cannot be read.
*
*
*
* If a SecurityException
is caught, the return value is
* null
and a message is written to System.err
.
*
*
* @param property the system property name
* @return the system property value or null
if a security
* problem occurs
*/
public static String getSystemProperty(String property) {
try {
return System.getProperty(property);
} catch (SecurityException ex) {
// we are not allowed to look at this property
System.err.println("Caught a SecurityException reading the system property '"
+ property
+ "'; the SystemUtils property value will default to null.");
return null;
}
}
/**
* Loads Properties from a file.
*
* @param filepath The target file's path
* @return java.util.Properties
*/
public static Properties load(String filepath) {
if (null == filepath || 0 == filepath.length()) {
throw new IllegalArgumentException("the path must not be empty.");
}
File file = new File(filepath);
return load(file);
}
public static Properties load(File file) {
if (!(file.exists() && file.isFile())) {
throw new IllegalArgumentException("the path [" + file.getAbsolutePath()
+ "] is not a correct file path.");
}
Properties prop = new Properties();
InputStream input = null;
try {
input = new FileInputStream(file);
prop.load(input);
} catch (IOException e) {
if (log.isWarnEnabled()) {
log.warn("load file [" + file.getAbsolutePath() + "] failed.");
}
} finally {
if(null != input) {
try {
input.close();
} catch (Exception e) {
}
}
}
return prop;
}
/**
* Loads properties from a classpath resource
*
* @param props
* @param resource e.g. conf/props/test.properties
* @param throwExceptionIfNotFound
* @return loaded properties
*/
public static Properties loadFromClassPath(String resource,
boolean throwExceptionIfNotFound) {
URL url = PropUtil.class.getClassLoader().getResource(resource);
if (url == null) {
if (throwExceptionIfNotFound) {
throw new IllegalStateException(
"Could not find classpath properties resource: "
+ resource);
} else {
return new Properties();
}
}
try {
Properties props = new Properties();
InputStream is = url.openStream();
try {
props.load(url.openStream());
} finally {
is.close();
}
return props;
} catch (IOException e) {
throw new RuntimeException(
"Could not read properties at classpath resource: "
+ resource, e);
}
}
public static Properties setValue(String filepath, String key, String value) throws IOException {
File file = new File(filepath);
if(!file.exists()) {
throw new RuntimeException("The properties file [" + filepath + "] doesn't exist.");
}
FileOutputStream out = null;
try {
Properties p = new Properties();
p.load(new FileInputStream(file));
p.setProperty(key, value);
// save to file
out = new FileOutputStream(file);
p.store(out, "Modified on " + new Date());
return p;
} finally {
if(null != out) {
try {
out.close();
} catch (Exception e) {
}
}
}
}
/**
* Merges multiple {@link Properties} instances into one
*
* @param mode merge mode
* @param sources properties to merge
* @return new instance of {@link Properties} containing merged values
*/
public static Properties merge(MergeMode mode, Properties... sources) {
Properties props = new Properties();
for (int i = 0; i < sources.length; i++) {
final Properties source = sources[i];
for (Entry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy