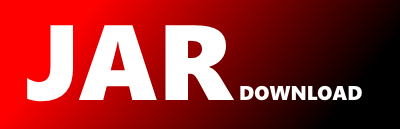
com.github.javaclub.configcenter.spring.config.ConfigPropertySource Maven / Gradle / Ivy
The newest version!
package com.github.javaclub.configcenter.spring.config;
import java.util.List;
import java.util.Set;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.core.env.EnumerablePropertySource;
import com.github.javaclub.configcenter.client.AppConfig;
import com.github.javaclub.configcenter.client.ConfigChangeListener;
import com.github.javaclub.toolbox.ToolBox.Strings;
import com.google.common.collect.Lists;
import com.google.common.collect.Sets;
/**
* Property source wrapper for AppConfig
*/
public class ConfigPropertySource extends EnumerablePropertySource {
private static final Logger log = LoggerFactory.getLogger(ConfigPropertySource.class);
private static final String[] EMPTY_ARRAY = new String[0];
private ConfigChangeListener listener;
private Set originPropertyNames = Sets.newConcurrentHashSet();
ConfigPropertySource(String name, AppConfig source) {
super(name, source);
}
@Override
public String[] getPropertyNames() {
Set propertyNames = this.source.getPropertyNames();
if (propertyNames.isEmpty()) {
return EMPTY_ARRAY;
}
List newPropertyNames = Lists.newArrayList();
for (String name : propertyNames) {
if(!originPropertyNames.contains(name)) {
newPropertyNames.add(name);
}
}
if (originPropertyNames.isEmpty()) {
log.info("{} ConfigKeys are adding to AutoUpdatelistener monitor.", newPropertyNames.size());
}
originPropertyNames.clear();
originPropertyNames.addAll(propertyNames);
// 新增加的key加入监听
for (String configKey : newPropertyNames) {
String configValue = source.getPropertyWithListener(configKey, listener);
if (!originPropertyNames.isEmpty() && log.isDebugEnabled()) {
log.debug("ConfigKey={} was Added to AutoUpdatelistener, configValue={}", configKey,
Strings.filter(configValue, true));
}
}
return propertyNames.toArray(new String[propertyNames.size()]);
}
@Override
public Object getProperty(String name) {
return this.source.getProperty(name, null);
}
public void addChangeListener(ConfigChangeListener listener) {
String[] array = getPropertyNames();
if (null != array && 0 < array.length) {
for (String configKey : array) {
source.getPropertyWithListener(configKey, listener);
}
}
this.listener = listener;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy