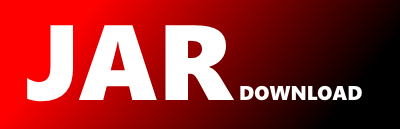
com.github.javaclub.configcenter.spring.utils.ServiceBootstrap Maven / Gradle / Ivy
The newest version!
/*
* @(#)ServiceBootstrap.java 2021-8-20
*
* Copyright (c) 2021. All Rights Reserved.
*
*/
package com.github.javaclub.configcenter.spring.utils;
import java.util.Collections;
import java.util.Comparator;
import java.util.Iterator;
import java.util.List;
import java.util.ServiceLoader;
import org.springframework.core.Ordered;
import com.google.common.collect.Lists;
/**
* ServiceBootstrap
*
* @author Gerald Chen
* @version $Id: ServiceBootstrap.java 2021-8-20 14:30:54 Exp $
*/
public class ServiceBootstrap {
public static S loadFirst(Class clazz) {
Iterator iterator = loadAll(clazz);
if (!iterator.hasNext()) {
throw new IllegalStateException(String.format(
"No implementation defined in /META-INF/services/%s, please check whether the file exists and has the right implementation class!",
clazz.getName()));
}
return iterator.next();
}
public static Iterator loadAll(Class clazz) {
ServiceLoader loader = ServiceLoader.load(clazz);
return loader.iterator();
}
public static List loadAllOrdered(Class clazz) {
Iterator iterator = loadAll(clazz);
if (!iterator.hasNext()) {
throw new IllegalStateException(String.format(
"No implementation defined in /META-INF/services/%s, please check whether the file exists and has the right implementation class!",
clazz.getName()));
}
List candidates = Lists.newArrayList(iterator);
Collections.sort(candidates, new Comparator() {
@Override
public int compare(S o1, S o2) {
// the smaller order has higher priority
return Integer.compare(o1.getOrder(), o2.getOrder());
}
});
return candidates;
}
public static S loadPrimary(Class clazz) {
List candidates = loadAllOrdered(clazz);
return candidates.get(0);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy