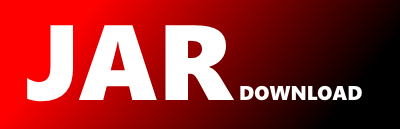
com.github.javaclub.delaytask.DelayJob Maven / Gradle / Ivy
/*
* @(#)DelayJob.java 2022-4-9
*
* Copyright (c) 2022. All Rights Reserved.
*
*/
package com.github.javaclub.delaytask;
import java.io.Serializable;
import java.util.Map;
import java.util.TreeMap;
import com.github.javaclub.toolbox.model.AttributesDO;
/**
* DelayJob
*
* @author Gerald Chen
* @version $Id: DelayJob.java 2022-4-9 10:37:24 Exp $
*/
public class DelayJob implements Serializable {
private static final long serialVersionUID = 3959873982889631156L;
public static final String CONTEXT_PARAM_CRON = "cron"; // cron表达式
public static final String CONTEXT_PARAM_TRIGGER_TIME = "triggerTime"; // 下次执行触发时间
public static final String CONTEXT_PARAM_TRIGGER_TIME_MILLS = "triggerTimeMills"; // 下次执行触发时间
public static final String CONTEXT_PARAM_QUEUE = "queue"; // 投递队列
public static final String CONTEXT_PARAM_DELIVERY_TIMES = "deliveryTimes"; // 被投递次数
public static final String CONTEXT_PARAM_MACHINE_HOST = "machineHost"; // 处理的机器节点host名
public static final String CONTEXT_PARAM_JOB_KEY = "jobKey"; // 当前延时任务实体的唯一jobKey
/**
* 同topic下唯一jobKey
*
* 如需要变更任务(如:提前/延后/取消/更新任务实体),必须指定jobKey的值(在同topic下需全局唯一)
*/
private String jobKey;
/**
* 业务实体对象
*/
private T entity;
private String entityClass;
private Map context;
public static DelayJob build(String jobKey, T entity) {
return new DelayJob(jobKey, entity);
}
public DelayJob() {
}
public DelayJob(T entity) {
this.entity = entity;
this.entityClass = entity.getClass().getName();
this.context = new TreeMap();
}
public DelayJob(T entity, Map context) {
this.entity = entity;
this.entityClass = entity.getClass().getName();
this.context = null == context ? new TreeMap() : context;
if (null != entity && entity instanceof AttributesDO) {
((AttributesDO) entity).putAll(context);
}
}
public DelayJob(String jobKey, T entity) {
this.jobKey = jobKey;
this.entity = entity;
this.entityClass = entity.getClass().getName();
this.addContextParam(CONTEXT_PARAM_JOB_KEY, jobKey);
}
public String getJobKey() {
return jobKey;
}
public void setJobKey(String jobKey) {
this.jobKey = jobKey;
this.addContextParam(CONTEXT_PARAM_JOB_KEY, jobKey);
}
public String getEntityClass() {
return entityClass;
}
public void setEntityClass(String entityClass) {
this.entityClass = entityClass;
}
public T getEntity() {
return entity;
}
public void setEntity(T entity) {
this.entity = entity;
this.entityClass = entity.getClass().getName();
}
public Map getContext() {
return context;
}
public void setContext(Map context) {
this.context = context;
}
public DelayJob addContextParam(String key, Object value) {
if (null == this.context) {
this.context = new TreeMap();
}
this.context.put(key, value);
if (null != entity && entity instanceof AttributesDO) {
((AttributesDO) entity).addAttribute(key, value);
}
return this;
}
public DelayJob addContextParams(Map params) {
if (null == this.context) {
this.context = new TreeMap();
}
this.context.putAll(params);
if (null != entity && entity instanceof AttributesDO) {
((AttributesDO) entity).putAll(params);
}
return this;
}
public DelayJob removeAttribute(String attrKey) {
if (null != entity && entity instanceof AttributesDO) {
((AttributesDO) entity).removeAttribute(attrKey);
}
return this;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy