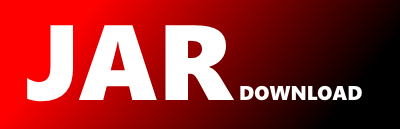
com.github.javaclub.jorm.common.UuidUtil Maven / Gradle / Ivy
/*
* @(#)UuidUtil.java 2011-6-16
*
* Copyright (c) 2011. All Rights Reserved.
*
*/
package com.github.javaclub.jorm.common;
import java.net.InetAddress;
import java.util.UUID;
/**
* A utility class of UUID.
*
* @author Gerald Chen
* @version $Id: UuidUtil.java 193 2011-08-03 15:51:58Z [email protected] $
*/
public abstract class UuidUtil {
/**
* Creates a new UUID string generated by JDK.
*
* @return UUID string
*/
public static String jdkUUID() {
UUID uuid = UUID.randomUUID();
return uuid.toString().replace("-", "");
}
/**
* This method is originated from hibernate framework.
*
* @return UUID string
*/
public static String newUUID() {
return new StringBuffer(36)
.append( format( getIP() ) ).append(sep)
.append( format( getJVM() ) ).append(sep)
.append( format( getHiTime() ) ).append(sep)
.append( format( getLoTime() ) ).append(sep)
.append( format( getCount() ) )
.toString();
}
private static String format(int intval) {
String formatted = Integer.toHexString(intval);
StringBuffer buf = new StringBuffer("00000000");
buf.replace( 8-formatted.length(), 8, formatted );
return buf.toString();
}
private static String format(short shortval) {
String formatted = Integer.toHexString(shortval);
StringBuffer buf = new StringBuffer("0000");
buf.replace( 4-formatted.length(), 4, formatted );
return buf.toString();
}
/**
* Unique across JVMs on this machine (unless they load this class
* in the same quater second - very unlikely)
*/
private static int getJVM() {
return JVM;
}
/**
* Unique in a millisecond for this JVM instance (unless there
* are > Short.MAX_VALUE instances created in a millisecond)
*/
private static short getCount() {
synchronized(UuidUtil.class) {
if (counter<0) counter=0;
return counter++;
}
}
/**
* Unique in a local network
*/
private static int getIP() {
return IP;
}
/**
* Unique down to millisecond
*/
private static short getHiTime() {
return (short) ( System.currentTimeMillis() >>> 32 );
}
private static int getLoTime() {
return (int) System.currentTimeMillis();
}
private static final int IP;
private static String sep = "";
static {
int ipadd;
try {
ipadd = CommonUtil.toInt( InetAddress.getLocalHost().getAddress() );
}
catch (Exception e) {
ipadd = 0;
}
IP = ipadd;
}
private static short counter = (short) 0;
private static final int JVM = (int) ( System.currentTimeMillis() >>> 8 );
public static void main(String[] args) {
for (int i = 0; i < 100; i++) {
System.out.println(newUUID());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy